Contact forms can be useful way for visitors to contact the owner of a site. They’re easy to use, and since they don’t expose the site owner’s email address in the page, they cut down on spam too.
However, contact forms can also be cumbersome, especially as they’re usually on a separate page. The visitor has to visit the contact form page, fill in the details, view yet another response page, and then try to make their way back to the page they were originally reading.
Fortunately, Ajax gives us a way round this problem. By embedding the form in the page, and submitting the form data via Ajax, the user never has to leave the current page. It also provides a smoother experience for the user.
In this tutorial we’ll build a nice-looking, embedded Ajax contact form that the user can summon up by clicking a link in the page. Along the way, we’ll explore various topics, including:
- HTML5 form fields
- How to use fallback techniques to make the form function even if the browser has JavaScript turned off
- Using CSS techniques to create attractive forms
- Writing a secure form mailer using PHP
- Animating page elements with jQuery, and, of course…
- Using jQuery to make Ajax requests
Before you begin, check out the finished product by clicking the View Demo button above. This opens a new page with some dummy content, and a couple of “Send us an email” links. Click one of these links to display the form.
The demo doesn’t actually send an email anywhere, but the finished code in the download is fully functional. To get the code, click the Download Code button above.
Ready? Let’s get started!
Step 1: Create the markup
Let’s start with the HTML for our page. This includes the form itself — we’ll hide it initially using JavaScript when the page loads — and also some dummy content and a couple of “Send us an email” links that will display the form when clicked:
<!doctype html> <html lang="en"> <head> <title>A Slick Ajax Contact Form with jQuery and PHP</title> <meta http-equiv="Content-Type" content="text/html;charset=utf-8"> </head> <body> <div id="content"> <p style="padding-bottom: 50px; font-weight: bold; text-align: center;"><a href="#contactForm">~ Send us an email ~</a></p> <!-- Content here --> <p style="padding-top: 50px; font-weight: bold; text-align: center;"><a href="#contactForm">~ Send us an email ~</a></p> </div> <form id="contactForm" action="processForm.php" method="post"> <h2>Send us an email...</h2> <ul> <li> <label for="senderName">Your Name</label> <input type="text" name="senderName" id="senderName" placeholder="Please type your name" required="required" maxlength="40" /> </li> <li> <label for="senderEmail">Your Email Address</label> <input type="email" name="senderEmail" id="senderEmail" placeholder="Please type your email address" required="required" maxlength="50" /> </li> <li> <label for="message" style="padding-top: .5em;">Your Message</label> <textarea name="message" id="message" placeholder="Please type your message" required="required" cols="80" rows="10" maxlength="10000"></textarea> </li> </ul> <div id="formButtons"> <input type="submit" id="sendMessage" name="sendMessage" value="Send Email" /> <input type="button" id="cancel" name="cancel" value="Cancel" /> </div> </form> <div id="sendingMessage" class="statusMessage"><p>Sending your message. Please wait...</p></div> <div id="successMessage" class="statusMessage"><p>Thanks for sending your message! We'll get back to you shortly.</p></div> <div id="failureMessage" class="statusMessage"><p>There was a problem sending your message. Please try again.</p></div> <div id="incompleteMessage" class="statusMessage"><p>Please complete all the fields in the form before sending.</p></div> </body> </html>
I’ve omitted the dummy content in the above code, since it’s not relevant to the tutorial.
The form sends its data to a processForm.php
script that does the actual emailing. (We’ll write this PHP script in a moment.) By setting the form’s action
attribute to "processForm.php"
, we ensure that the form is usable even with JavaScript disabled. Later, our JavaScript will read this action
attribute so that it knows where to send the Ajax request.
The form itself uses some HTML5 form features such as placeholders, the email
field type, and the required
attribute to ensure that all the fields have been filled in. We’ll also add JavaScript validation for browsers that don’t yet support HTML5 validation.
Step 2: Add the CSS
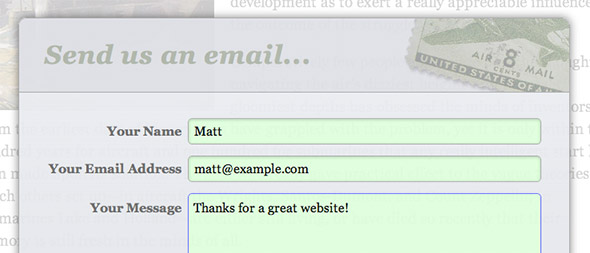
Now we’ll add the CSS to our HTML page in order to style the page and form. The bulk of the CSS positions the form and status messages in the centre of the window, and styles the form and form fields.
<style type="text/css"> /* Add some margin to the page and set a default font and colour */ body { margin: 30px; font-family: "Georgia", serif; line-height: 1.8em; color: #333; } /* Set the content dimensions */ #content { width: 800px; padding: 50px; margin: 0 auto; display: block; font-size: 1.2em; } #content h2 { line-height: 1.5em; } /* Add curved borders to various elements */ #contactForm, .statusMessage, input[type="submit"], input[type="button"] { -moz-border-radius: 10px; -webkit-border-radius: 10px; border-radius: 10px; } /* Style for the contact form and status messages */ #contactForm, .statusMessage { color: #666; background-color: #ebedf2; background: -webkit-gradient( linear, left bottom, left top, color-stop(0,#dfe1e5), color-stop(1, #ebedf2) ); background: -moz-linear-gradient( center bottom, #dfe1e5 0%, #ebedf2 100% ); border: 1px solid #aaa; -moz-box-shadow: 0 0 1em rgba(0, 0, 0, .5); -webkit-box-shadow: 0 0 1em rgba(0, 0, 0, .5); box-shadow: 0 0 1em rgba(0, 0, 0, .5); opacity: .95; } /* The form dimensions */ #contactForm { width: 40em; height: 33em; padding: 0 1.5em 1.5em 1.5em; margin: 0 auto; } /* Position the form in the middle of the window (if JavaScript is enabled) */ #contactForm.positioned { position: fixed; top: 0; bottom: 0; left: 0; right: 0; margin-top: auto; margin-bottom: auto; } /* Dimensions and position of the status messages */ .statusMessage { display: none; margin: auto; width: 30em; height: 2em; padding: 1.5em; position: fixed; top: 0; bottom: 0; left: 0; right: 0; } .statusMessage p { text-align: center; margin: 0; padding: 0; } /* The header at the top of the form */ #contactForm h2 { font-size: 2em; font-style: italic; letter-spacing: .05em; margin: 0 0 1em -.75em; padding: 1em; width: 19.5em; color: #aeb6aa; background: #dfe0e5 url('images/stamp.jpg') no-repeat 15em -3em; /* http://morguefile.com/archive/display/606433 */ border-bottom: 1px solid #aaa; -moz-border-radius: 10px 10px 0 0; -webkit-border-radius: 10px 10px 0 0; border-radius: 10px 10px 0 0; } /* Give form elements consistent margin, padding and line height */ #contactForm ul { list-style: none; margin: 0; padding: 0; } #contactForm ul li { margin: .9em 0 0 0; padding: 0; } #contactForm input, #contactForm label { line-height: 1em; } /* The field labels */ label { display: block; float: left; clear: left; text-align: right; width: 28%; padding: .4em 0 0 0; margin: .15em .5em 0 0; font-weight: bold; } /* The fields */ input, textarea { display: block; margin: 0; padding: .4em; width: 67%; font-family: "Georgia", serif; font-size: 1em; border: 1px solid #aaa; -moz-border-radius: 5px; -webkit-border-radius: 5px; border-radius: 5px; -moz-box-shadow: rgba(0,0,0,.2) 0 1px 4px inset; -webkit-box-shadow: rgba(0,0,0,.2) 0 1px 4px inset; box-shadow: rgba(0,0,0,.2) 0 1px 4px inset; background: #fff; } textarea { height: 13em; line-height: 1.5em; resize: none; } /* Place a border around focused fields, and hide the inner shadow */ #contactForm *:focus { border: 1px solid #66f; outline: none; box-shadow: none; -moz-box-shadow: none; -webkit-box-shadow: none; } /* Display correctly filled-in fields with a green background */ input:valid, textarea:valid { background: #dfd; } /* The Send and Cancel buttons */ input[type="submit"], input[type="button"] { float: right; margin: 2em 1em 0 1em; width: 10em; padding: .5em; border: 1px solid #666; -moz-border-radius: 10px; -webkit-border-radius: 10px; border-radius: 10px; -moz-box-shadow: 0 0 .5em rgba(0, 0, 0, .8); -webkit-box-shadow: 0 0 .5em rgba(0, 0, 0, .8); box-shadow: 0 0 .5em rgba(0, 0, 0, .8); color: #fff; background: #0a0; font-size: 1em; line-height: 1em; font-weight: bold; opacity: .7; -webkit-appearance: none; -moz-transition: opacity .5s; -webkit-transition: opacity .5s; -o-transition: opacity .5s; transition: opacity .5s; } input[type="submit"]:hover, input[type="submit"]:active, input[type="button"]:hover, input[type="button"]:active { cursor: pointer; opacity: 1; } input[type="submit"]:active, input[type="button"]:active { color: #333; background: #eee; -moz-box-shadow: 0 0 .5em rgba(0, 0, 0, .8) inset; -webkit-box-shadow: 0 0 .5em rgba(0, 0, 0, .8) inset; box-shadow: 0 0 .5em rgba(0, 0, 0, .8) inset; } input[type="button"] { background: #f33; } /* Header/footer boxes */ .wideBox { clear: both; text-align: center; margin: 70px; padding: 10px; background: #ebedf2; border: 1px solid #333; } .wideBox h1 { font-weight: bold; margin: 20px; color: #666; font-size: 1.5em; } </style> <!-- Some IE7 hacks and workarounds --> <!--[if lt IE 8]> <style> /* IE7 needs the fields to be floated as well as the labels */ input, textarea { float: right; } #formButtons { clear: both; } /* IE7 needs an ickier approach to vertical/horizontal centring with fixed positioning. The negative margins are half the element's width/height. */ #contactForm.positioned, .statusMessage { left: 50%; top: 50%; } #contactForm.positioned { margin-left: -20em; margin-top: -16.5em; } .statusMessage { margin-left: -15em; margin-top: -1em; } </style> <![endif]-->
Let’s look at some interesting sections of the CSS:
- Style for the contact form and status messages
We give the form and status boxes a nice gentle top-to-bottom gradient using-webkit-gradient
and-moz-linear-gradient
, and we also add a drop shadow withbox-shadow
(and its vendor-specific variants). Finally, we give the form and message boxes an opacity of .95 (95%), which makes the page content just show through — a nice subtle effect. - Position the form in the middle of the window (if JavaScript is enabled)
Initially, we simply place the form inline after the page content. This is so that the form can be used for non-JavaScript-enabled browsers without getting in the way of the content. However, for JavaScript browsers, we want the form to appear in the centre of the window, over the top of the content.Our
#contactForm.positioned
rule does just that. It uses fixed positioning, sets thetop
,bottom
,left
andright
values all to zero, and ensures that all 4 margins are set toauto
. This centres the element both horizontally and vertically in most modern browsers. Later we’ll use our JavaScript to add thepositioned
class to the form.We also position the status message boxes in the same way.
- The header at the top of the form
Our form includes a nice “Send us an email…” header with an image of a postage stamp. Our#contactForm h2
rule styles this header. We give the text a large italic style and space the letters out slightly. We also add margin and padding to create space around and inside the header. We use some negative left margin (-.75em) on the header to bypass the padding on the form, so that the header goes right to the left edge of the form. We also set the width of the header to 19.5em so that it exactly matches the width of the form.Why -.75em and 19.5em? Because ems cascade, and we’ve set our font size to 2em. So -.75em actually becomes -1.5em (the width of the form’s padding), and 19.5em becomes 39em (the width of the form, minus 1em for the
h2
‘s padding). Phew! Maybe I’ll use pixels next time… 🙂We also set the heading’s colour, give it a dark background, position the postage stamp image in the top right corner, add a thin bottom border, and add curved top corners.
- The fields
We give theinput
andtextarea
fields an attractive font, a rounded border usingborder-radius
, and a gentle inner shadow usingbox-shadow
. We also float the field labels left so that they sit alongside the fields. When a field is focused (clicked on or moved to with the Tab key), we give it a blue border and remove the shadow. We also setoutline: none
to remove the blue outline added by some browsers. Finally, we use the:valid
pseudo-class to give correctly completed fields a green background, for those browsers that support HTML5 form validation. - The Send and Cancel buttons
input[type="submit"]
selects the Send Email button, whileinput[type="button"]
selects the Cancel button. We float them right to position them side by side, and add some margin to give them space. We give them a fixed width, and some padding to make them a decent size. We add a rounded border and subtle drop shadow, and specify text and background colours. We also make the buttons slightly transparent (opacity: .7
), and make them fully transparent when hovered over to highlight them. We use a CSS transition to fade the opacity slowly. Finally, when the buttons are clicked (:active
) we move the shadow inside the buttons to give a “pressed” appearance, and give them a black-on-white colour scheme.
Step 3: Build the PHP form mailer
We’ve now created our form page, and styled the form. The next step is to build a short PHP script to actually send the email messages. This script will reside on the web server. When the user submits the form, the form’s data is sent to the PHP script, which then sends the email and returns a response indicating whether or not the email was sent successfully.
Here’s the PHP script — call it processForm.php
, and save it in the same folder as the form page you created in Steps 1 and 2:
<?php // Define some constants define( "RECIPIENT_NAME", "John Smith" ); define( "RECIPIENT_EMAIL", "[email protected]" ); define( "EMAIL_SUBJECT", "Visitor Message" ); // Read the form values $success = false; $senderName = isset( $_POST['senderName'] ) ? preg_replace( "/[^.-' a-zA-Z0-9]/", "", $_POST['senderName'] ) : ""; $senderEmail = isset( $_POST['senderEmail'] ) ? preg_replace( "/[^.-_@a-zA-Z0-9]/", "", $_POST['senderEmail'] ) : ""; $message = isset( $_POST['message'] ) ? preg_replace( "/(From:|To:|BCC:|CC:|Subject:|Content-Type:)/", "", $_POST['message'] ) : ""; // If all values exist, send the email if ( $senderName && $senderEmail && $message ) { $recipient = RECIPIENT_NAME . " <" . RECIPIENT_EMAIL . ">"; $headers = "From: " . $senderName . " <" . $senderEmail . ">"; $success = mail( $recipient, EMAIL_SUBJECT, $message, $headers ); } // Return an appropriate response to the browser if ( isset($_GET["ajax"]) ) { echo $success ? "success" : "error"; } else { ?> <html> <head> <title>Thanks!</title> </head> <body> <?php if ( $success ) echo "<p>Thanks for sending your message! We'll get back to you shortly.</p>" ?> <?php if ( !$success ) echo "<p>There was a problem sending your message. Please try again.</p>" ?> <p>Click your browser's Back button to return to the page.</p> </body> </html> <?php } ?>
This script is fairly straightforward. Let’s break it down:
-
- Define some constants
First we define some config options for the name and email address of the person who will receive the email message. (Change these to your own name and email address.) We also set a subject for the message. - Read the form values
Next we check for our 3 form fields,senderName
,senderEmail
andmessage
, in the posted form data. For each field, we check if it exists. If it does then we pass its value through a regular expression to remove any potentially malicious characters that a spammer might try to use, and store the result in a variable. If it doesn’t exist then we set the variable to an empty value. - If all values exist, send the email
If the 3 field values all contain data then we send the email. First we construct the recipient string from the recipient name and email address. Then we add a"From:"
header to the message using the name and email address that the visitor entered in the form. This is the “From:” value that the recipient will see in their email program. Finally, we use the PHPmail()
function to send the email message, storing the return value in the variable$success
. (mail()
returnstrue
if it managed to send the email, orfalse
otherwise.) - Return an appropriate response to the browser
Once we’ve attempted to send the email, we send a “success” or “error” message back to the browser as appropriate. If the request URL contained an"ajax"
parameter then we know the form was submitted via Ajax using our JavaScript code, so we simply return the value"success"
or"error"
to the JavaScript, which will then display an appropriate message to the user. However, if the form was submitted without using Ajax then the user must have JavaScript turned off in their browser. In this situation, we display a more helpful error message in the browser, with instructions to the user to use their Back button to return to the page.Our JavaScript will add the
ajax
parameter to the URL when it submits the form, as you’ll see in Step 6.
- Define some constants
Step 4: Include the jQuery library and set the delay
Our form is actually functional now. You can open the page in a browser, click the “Send us an email” link to jump to the form, fill in the fields, and submit the form to send the message.
However, we’re now going to enhance our form using JavaScript to make the experience nicer for the user.
We’ll use jQuery to do most of the heavy lifting, so the first step is to include the jQuery library in the page’s head
element:
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.5.1/jquery.min.js"></script>
Here we’ve linked directly to the jQuery library on Google’s CDN, but you can download the library and host it on your own server if you prefer.
We’ll also add a global config variable, messageDelay
, to control how long the message boxes appear on the screen. This value is in milliseconds. Feel free to change it to a shorter or longer time:
<script type="text/javascript"> var messageDelay = 2000; // How long to display status messages (in milliseconds)
Step 5: Write the init()
function
The first stage of our form-enhancing JavaScript is the init()
function. This sets up the form so that it can be shown and hidden on demand, and also modifies the form so that it will be submitted using our JavaScript, rather than sent natively by the browser.
Here’s the code:
// Init the form once the document is ready $( init ); // Initialize the form function init() { // Hide the form initially. // Make submitForm() the form’s submit handler. // Position the form so it sits in the centre of the browser window. $('#contactForm').hide().submit( submitForm ).addClass( 'positioned' ); // When the "Send us an email" link is clicked: // 1. Fade the content out // 2. Display the form // 3. Move focus to the first field // 4. Prevent the link being followed $('a[href="#contactForm"]').click( function() { $('#content').fadeTo( 'slow', .2 ); $('#contactForm').fadeIn( 'slow', function() { $('#senderName').focus(); } ) return false; } ); // When the "Cancel" button is clicked, close the form $('#cancel').click( function() { $('#contactForm').fadeOut(); $('#content').fadeTo( 'slow', 1 ); } ); // When the "Escape" key is pressed, close the form $('#contactForm').keydown( function( event ) { if ( event.which == 27 ) { $('#contactForm').fadeOut(); $('#content').fadeTo( 'slow', 1 ); } } ); }
Let’s look at each chunk of the above code:
-
-
-
- Init the form once the document is ready
We use the jQuery object,$
, to trigger ourinit()
function once the DOM is ready. - Hide the form, set the submit handler, and position the form
The first thing we do inside theinit()
function itself is make some changes to our form,#contactForm
.First we hide it from the page using the jQuery
hide()
method. Then we set itssubmit
event handler to oursubmitForm()
function (which we’ll write in a moment). This ensures that, when the user submits the form,submitForm()
is called instead of the native browser form submission kicking in. Finally, we add thepositioned
CSS class to the form to reposition it in the centre of the browser window. - Make the “Send us an email” links open the form
Next we bind an anonymous event handler function to the “Send us an email” links’click
events. This function fades out the page content so it’s only just visible in the background; fades the contact form in; and sets the focus on the “Your Name” field, ready for the user to start filling in the form. Finally, the function returnsfalse
to prevent the links from being followed. - When the “Cancel” button is clicked, close the form
Now we bind another anonymous function to the “Cancel” button’sclick
event, so that the user can close the form by clicking the button. The function simply fades the form out, and fades the page content back in. - When the “Escape” key is pressed, close the form
Similarly we bind a function to the contact form’skeydown
event, so that we can read any key the user presses when they’re viewing the form. In this function, we check if the user has pressed the “Escape” key (character code: 27). If they have then we close the form by fading it out, and fading the content in.
- Init the form once the document is ready
-
-
Step 6: Write the submitForm()
function
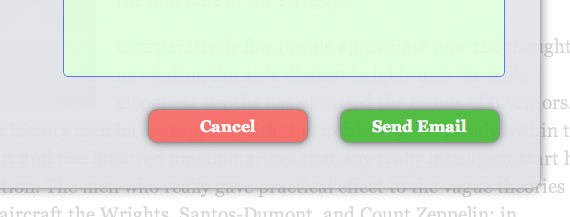
We’ve now set up our form so that, rather than being submitted in the usual fashion, it will trigger the submitForm()
function when the user submits it. This function needs to do some validation and, if all is well, submit the form data to the PHP script via Ajax.
Here’s the function in full:
// Submit the form via Ajax function submitForm() { var contactForm = $(this); // Are all the fields filled in? if ( !$('#senderName').val() || !$('#senderEmail').val() || !$('#message').val() ) { // No; display a warning message and return to the form $('#incompleteMessage').fadeIn().delay(messageDelay).fadeOut(); contactForm.fadeOut().delay(messageDelay).fadeIn(); } else { // Yes; submit the form to the PHP script via Ajax $('#sendingMessage').fadeIn(); contactForm.fadeOut(); $.ajax( { url: contactForm.attr( 'action' ) + "?ajax=true", type: contactForm.attr( 'method' ), data: contactForm.serialize(), success: submitFinished } ); } // Prevent the default form submission occurring return false; }
Here’s how the function works:
-
-
-
- Store the contact form in a variable
Since we’ll be using it a lot throughout the function, we start off by storing the contact form element in acontactForm
variable. This element is available to our function as thethis
variable, since the function is the event handler for the element’ssubmit
event. We wrap the element in a jQuery object to make it easier to work with. - Check all the fields are filled in
Now we check that each field’s value is not empty by using the jQueryval()
method on each field. - Display a warning if the form isn’t completed
If 1 or more of the fields are empty, we fade out the form, then fade in the#incompleteMessage
div
, which contains the “Please complete all the fields…” message. We keep the message there for the time specified by themessageDelay
variable, then fade it out again. Once it’s faded out, we fade the form back in so that the user can complete it. - Submit the form if it is completed
Now we get to the meat of the JavaScript. If the form is completed then we first fade out the form, and fade in the “Sending your message…” box. Now we call the jQueryajax()
method to submit the form via Ajax to the PHP script. We pass the following arguments to the method:url
- The URL to send the form to. We grab this from the form’s
action
attribute, and append anajax=true
parameter to the query string so that our PHP script knows the form was sent via Ajax, rather than via the usual method. type
- The type of request to make (
"POST"
or"GET"
). We grab this from the form’smethod
attribute, which in this case is set to"POST"
. data
- The data to send with the request. For this, we call the jQuery
serialize()
method on the contact form object. This method takes all the field names and values in the form and encodes the data in a query string. We then pass this string to theajax()
method so it can send the data to the PHP script. success
- This is a callback function that will be called once the Ajax request has finished and the browser has received the response from the server. We set this to our
submitFinished()
function, which we’ll write in a moment.
- Prevent the default form submission occurring
Finally, our event handler returnsfalse
to prevent the form being submitted in the usual way.
- Store the contact form in a variable
-
-
Step 7: Write the submitFinished()
function
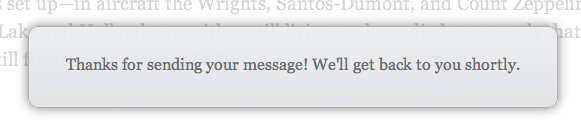
The last function we need to write is submitFinished()
, which is called once the Ajax response from the PHP script has been received by the browser. This function needs to check the response and display a success or error message as appropriate:
// Handle the Ajax response function submitFinished( response ) { response = $.trim( response ); $('#sendingMessage').fadeOut(); if ( response == "success" ) { // Form submitted successfully: // 1. Display the success message // 2. Clear the form fields // 3. Fade the content back in $('#successMessage').fadeIn().delay(messageDelay).fadeOut(); $('#senderName').val( "" ); $('#senderEmail').val( "" ); $('#message').val( "" ); $('#content').delay(messageDelay+500).fadeTo( 'slow', 1 ); } else { // Form submission failed: Display the failure message, // then redisplay the form $('#failureMessage').fadeIn().delay(messageDelay).fadeOut(); $('#contactForm').delay(messageDelay+500).fadeIn(); } } </script>
The function works as follows:
-
-
-
- Get the response
jQuery passes the response from the PHP script as an argument to thesubmitFinished()
function. We take this string and pass it through the jQuerytrim()
method to remove any whitespace. - Fade out the “sending” message
Next we fade out the “Sending your message…” box by calling the jQueryfadeOut()
method. - If email was sent successfully, display a success message
If theresponse
variable holds the string"success"
, as returned by our PHP script, then we know that the email was successfully queued for delivery. So we fade in the success message, hold it for a couple of seconds, then fade it out. We also reset the form fields to empty values, in case the user wants to send another message. Finally, once the success message has faded out, we fade the page content back in. - If there was a problem, display a failure message
If the PHP script returned anything other than"success"
then we know there was a problem with the submission, so we display the failure message stored in the#failureMessage
div
, then fade the form back in so that the user can correct any problems with the form.
- Get the response
-
-
And that’s the end of our JavaScript!
Summary
We’ve now built our slick Ajax contact form. Not only does it look good, but it’s easy to use, and the visitor can send an email without ever having to leave the page they are reading. Nice!
Here’s the demo again:
I hope you enjoyed following this tutorial. Feel free to use the code in your own projects if you like. As always, if you have any comments, suggestions or questions on the tutorial, please do post them in the responses below.
Have fun!
I can’t believe nobody has commented yet! This tutorial is amazing and so helpful! Thank you!
@DestMoonDesigns: Yay, thanks for your feedback – glad you liked it! 🙂
Yes, this is amazing and well done. Bravo and thank you.
I’m trying to use it for a registration form. It’s very nice.
I wonder what is the syntax for adding to the submitForm function a regex validation.
Let’s say the regex below.
All my best.
Slick tool. I like it!
I have a question though…
I would like to place the css and javascript code that are now in the index.html file, in separate files, which I can include with tags like so:
<link rel=”stylesheet” type=”text/css” href=”/ajax-contact-form/ajax-contact-form.css”>
<script type=”text/javascript” src=”/ajax-contact-form/ajax-contact-form.js”>
<script type=”text/javascript” src=”http://ajax.googleapis.com/ajax/libs/jquery/1.5.1/jquery.min.js”></script>
Is this possible?
@hcharles: Thanks!
How about something like:
?
Thank you Matt.
Yes it’s exactly what I needed.
Great site, great job.
@mktngguy: Yep, totally possible. Although you should put the jQuery include before the others.
@hcharles: Great stuff!
wow matt, THIS is exactly what i have been looking for in a long time!
This is one of the most comprehensive tutorials i have read in a long long time! So much effort in it… i can tell you love what you do and i do respect that a lot!
I just registered to tell you that 🙂
Greetings from Germany!
@phrill: Thank you so much for your kind words – that’s really great to hear! 🙂
Cheers,
Matt
this is excellent, thanks for making it available..
i have a small problem, the form comes up on the site, i can fill in details, and send, but…
it says…sending message….
but then says…there was a problem, try again….
but i’m receiving the sent message in my inbox, so as far as i can tell there was no problem sending ?
@naval1: That’s strange. Your PHP script might be exiting abnormally for some reason – that would stop it returning the correct response to the JavaScript. You’ll need to do some debugging I think. You could try disabling JavaScript in your browser, and adding ?ajax=true to your form action:
<form id=”contactForm” action=”processForm.php?ajax=true” method=”post”>
This should make the PHP script think it’s handling an Ajax request. You can then see the response from the PHP script in the page.
You could also try using PHP’s error_log() function and the error_log directive to log diagnostic messages to a log file on your server. This might help you work out what’s happening inside the PHP script.
Thanks Matt,
I’m very new to web site design and its taken me months to design my own site with no previous html, java script or php experience.
I’ve followed your instructions to the letter, and saw the response from the php script and found that the problem was with script recommended by my site hosts, details as follows:
To prevent spam being sent through our webservers, there are certain conditions that must be met before our SMTP servers will send the email.
Email must be sent to, or from, an existing email address hosted by Fasthosts. This must be a mailbox on the same domain name on which the form to mail script is being hosted.
To stop misuse of your form by third parties the sendmail_from variable should be set to your Fasthosts hosted email address. While access to the php.ini file is restricted on our shared environment, you can sent this variable using the ini_set() command, shown below.
A fifth parameter, -f, should be added to the sendmail function. This will set the name of the from email address.
In its basic form, a simple sendmail script will look like this:
<?php
ini_set(“sendmail_from”, “[email protected]”);
mail($email_to, $email_subject, $email_message, $headers, “[email protected]”);
?>
Provided that your form to mail script meets the requirements above, you should have no problems.
I’ve removed their recommended php script and everything works fine now. Is there anything I need to do or do I just leave things as they are ?
Again thank you very much for your time, what a very informative site you run, thank you.
@naval1: Not sure how your mail was getting sent in that case, but anyway…
If it ain’t broke, don’t fix it, is my motto! 🙂
Matt
Hello Matt,
Based on your wonderful tutorial, I’ve created a registration Form.
After the form is submitted, I’m using a php script to create an image (imagecreatefrompng).
Emailing the created image to the registered user is working fine.
Now I’m trying to send the img to the browser.
The header is set in my php code
My question is how to reprocess the return by Ajax in order to display the image for download purpose.
I read lot today about this question; until I get lost. 😀
In advance Thanks.
-Charles
Just had to say this is TOTALLY AWESOME!!! I incorporated it into the new wordpress theme I am designing for my site and it works great.
Can’t thank you enough. Keep up the good work.
Hello Matt,
In my previous post I was asking you: “how to reprocess the return by Ajax in order to display an image for download purpose”.
The idea is to use AJAX to modify the content which was displayed from the index.html before the form is submitted; as part of the form’s submission result.
I managed to do so. 🙂
To share with others, here’s how:
In File “index.html”, I added a new div.
That’s the div I’m going to modify through the AJAX Response.
In processFrom.php, I modified the response to the browser. I just added my info (file url) to the success variable.
The original code from elated.com to modify is
After my modifications it looks like this
The var file_link was created by some earlier php codes in the processFrom.php file. It contains an img tag or a “File not created error message”.
The last thing to do was to modify the response processing code in index.html.
The original code from elated.com to modify is within the submitFinished function
Changes are like this
Just after this code I changed the original
to
and just before the original code
I added my new info (img tag) to the div I’ve created at the beginning
I started learning AJAX a few days ago thanks to this tutorial from Matt.
Thanks Matt.
[Edited by hcharles on 08-May-11 02:49]
Awesome tutorial!!
I am using all this information for a website that I am building, but there is one problem that I can’t solve…
When I submit the form it always return an error:
“There was a problem sending your message. Please try again.” (The message from failureMessage)
I have checked the code several times but found no errors… I’m at a loss…
Can someone help me?
Here is the code for the form:
Here is the code for the JavaScript:
Here is the code for the php mailer:
Thank you in advance!
[Edited by maruse on 09-May-11 05:32]
@Maruse,
Hello.
I had the same problem with php mail function.
It was in relation with the recipient’s DNS configuration.
MX records is not configured.
Try to use the PEAR::Mail function. It’s more robust.
Working for me.
Good luck.
-Charles
@hcharles
Thank you for your response but, please excuse my ignorance but I’m new to PHP and my knowledge is very limited.
Can you show me how to implement that function in my code?
Thank you.
@Maruse,
Pear is a PHP Extension.
You need to check if it is installed on your server.
Follow this doc:
There are a lot of “Pear – howto” in the net.
You may give a look at this one for some samples of Text or html email, with one or more attachments:
Hope this helps.
-Charles
@tsquez: Great stuff, thanks for your kind words 🙂
@hcharles: Yep, your image mod is pretty much how I’d have done it too!
@maruse: You might also want to turn on PHP error logging – that might tell you exactly what the problem is with the script:
http://www.php.net/manual/en/errorfunc.configuration.php#ini.log-errors
http://www.php.net/manual/en/errorfunc.configuration.php#ini.error-log
It’s likely that your PHP mail config needs tweaking. See @naval1’s post earlier in the thread re sendmail_from etc.
Matt
Thank you all for your help, but it seems I can’t get it to work…
I found what is the error.
If I fill the contact form using English, then everything is fine but if I fill the form in Japanese, then it breaks and I get the error message.
The encoding of my page is UTF-8 so I don’t understand why I get that error.
Any ideas?
Edited:
I found the problem. It wasn’t the encoding but one of the functions in the php file.
I replaced preg_replace with strip_tags and now everything is working fine even in Japanese.
[Edited by maruse on 10-May-11 23:16]
@maruse: Glad you got it working! Re preg_replace(), try using the ‘u’ flag:
http://th1rty7.blogspot.com/2008/12/unicode-and-pregreplace.html
strip_tags() is OK but it’s better to use preg_replace() with a whitelist if possible.
Hi Matt,
Thank you for this great tutorial. It’s looking beautiful but unfortunately I can’t get it to work properly. After I fill in all the fields and submit the form I get the “incompleteMessage” error message. I imagine there’s a problem with the validation process but as I’m new the php I can be sure. Any way you can take a look for me?
I kept things pretty simple as you had in your demo, here’s my html:
Here’s the php:
And here’s the javascript, I wanted to remove the hide functionality you had for the demo which is why some of the code has been commented out. I want the form to be there once you arrive at the section and only hide once you submit the form.
Thank you! I will thoroughly appreciate your (additional) help!
Denise
@drot: Please post the URL of your complete page in a new topic so we can see the exact problem in action:
http://www.elated.com/forums/authoring-and-programming/topic/new/
you are awesome! thank you!
@ninka: You’re welcome 🙂
Thanks Matt for the awesome tutorial…
I have a question : I want to add more fields to the form. When I tried adding, some fields and the buttons below were no longer visible in the form.
How do I add a scroll bar to the form in case there are more fields for input? Please help.
@aditi_sharma: You could try:
Does that help?
Cheers,
Matt
How can I change the form to a registration form? I tried to change the processform.php to insert the information but I guess that’s not the way, can anyone help? thanks!!
@topsyk: That certainly is the way! If you’re having specific problems then please post your PHP script in a separate topic and we can take a look:
http://www.elated.com/forums/authoring-and-programming/topic/new/
Hi,
Nice Contact Form Sample. I make an alternative for those are using servers like yahoo server.
with yahoo server or any other similar the email has to be send it from inside or it won’t go trough.
enjoy
mbarcala
Hi Matt,
This is the exact functioning form I have been looking for. Looks great and works great! Except for one little thing…sorry.
I fill out the form and click submit. I get the “Sending your message” message. Then the “thanks for sending your message” message. But then the message just stays there. I’ve tried adjusting the
var messageDelay = 2000; line of code to a lower number, but not avail.
I should mention that the email does go through, with all headers, email addresses and text in place.
My page is here: http://www.ericmlyons.com/index_contact.html.
And here is the code I’m using:
the javascript (please note that I replaced #content with #linkwrapper as this is where my content is)
and
My php code:
I would really appreciate the help. It’s working exactly how i need it to work, except for that glaring issue. You rock! Thank you so much.
Eric
Love this tutorial, but I am new to all of this. I want to add a field for a phone number and a drop down selection box to choose your product, but it won’t post.
Also now, (for some reason) When I try the form out I get the success message, but no email comes. What am I doing wrong?
@edub187: You’re using a very old version of jQuery (1.3.2). Try the latest version (1.6.2): http://jquery.com/
@10000nails: Please post the URL of your form in a new topic so we can take a look at it in action:
http://www.elated.com/forums/authoring-and-programming/topic/new/
Matt,
Thank you SO much. I updated my jquery link and it works flawlessly. Great tutorial. Great outcome. And great assistance. Thanks again.
Eric
Hi Matt,
I would like to know how could I pass an info to the subject or textarea part in the form from a button or a regular link using innerhtml action.
let say that I have a button that say “click me” and i want when click the button i pass the “picture number or name” inside the subject or the Textarea part.
I try to follow many samples from the internet but any of those samples let me place the value or the text i want inside the input field in the form? so can you give me a hand on this please?
thanks
mbarcala
@edub187: Great stuff 🙂
@mbarcala: If I understand it correctly, your question is not really related to the article – it’s more a general JavaScript forms question. Please post it in a new topic:
http://www.elated.com/forums/authoring-and-programming/topic/new/
Cheers,
Matt
I was wondering if there was a way to CC a couple of email addresses? Great script by the way. works like a charm.
Kate
I just set the forward in the mail client. =)
@redfraggle42: Yep:
This is a wonderful contact form. It is exactly what I wanted…
I just have one question: When the contact form is visible, there’s kind of a white layer beneath the form and over part of the page (like a veil).
Is it possible to extend this “veil” to the entire page?
I’m not sure if I’m explaining myself well….
@perfisa
I believe in order to veil the entire page, you need to select which div you want in the fade to portion of the javascript init function. For example:
where linkwrapper is the area of the page you wish to white out. That’s how I did it on my page, and it seems to work. Please correct me if I’m wrong.
@ edub187
Thank you very much. It worked perfectly.
Bravo.
Toutes mes félicitations.
After a little issue I finally made it.
Tous mes remerciements.
(that means, warm thanks).
@Sventovit: Pas de problème. Je suis content qu’il est fixé maintenant! 🙂
Ah ah!
Beware… As you improve my coding skills, I’m might improve your french. ;-p
Matt, may I suggest one thing?
Thank you.
You should add the Google Plus 1 button (http://www.google.com/+1/button/) to your pages.
Your articles worth it.
@Sventovit: Ha ha, yes my French is not very good these days 🙂
Thanks for the +1 suggestion – we’ll look into it.
Hi Matt,
I have another question in reference to this email form, i would like to know how I can format the email body that I will receive from the web in the php file? I mean how i can put it like this
name
phone
message here
I don’t know if this have to be a new post?
I add a new field for a phone information but it came under the message part? this is for my own eyes purpose only but i really would like to know if this can be done in some point in this way
Regards
mabrcala
i need to define the from address and have the senders email address be set as a reply to address. it seems to be required by godaddy that the from address be from the same domain as the website itself otherwise no email is sent. i have tried and tried to get it to work but no matter what i do to try and fix still it gives me the you must fill in all boxes message when i hit send. in the html file i renamed the box for email address “replyEmail” and set a define in the top of php for the from… here’s my php i am fairly sure the problem is here:
also here is the link to my test page (just the original demo page until i get contact form working) :
http://theaxnyc.com/newformfile.html
thanks for any help!
@mbarcala @theaxnyc: Please post your questions in new topics:
http://www.elated.com/forums/authoring-and-programming/topic/new/
ok… but my post did pertain directly to this thread… i think, perhaps, i will need to place a link back to this thread in my new one as this was my starting code.?
Hi Matt,
I’ve got a problem in setting charset = “utf-8”
How do you insert the charset = “utf-8” to the processForm.php
Thanks
i don’t know what i am doing wrong. I am getting two problems.
1. The status message font colour is same as the status window. So cant see anything.
2. the message is stuck at please wait sending you email..
Then nothing happens.
pls . help
Anupam Thakur
@xarkan: See http://www.elated.com/forums/topic/5263/ and make sure your contact form page has a <meta charset=”utf-8″ /> tag.
@anupam347: Please post the URL of your form in a new topic, along with a description of the problem:
http://www.elated.com/forums/authoring-and-programming/topic/new/
Can someone help me with adding SNTP authentication? Neat code btw 🙂
Hey matt..
First of all, hats off for such a good tutorial.!! Great work!!
I am having a problem with this tutorial. I am new to php and ajax..
I followed your tutorial as it is, but when I fill the form and click Send Email, I get the error message “Please complete all the fields in the form before sending.” even though all fields in the form are filled.
Also the error message doesn’t fade out.. Is there any additional step to do this.
Here is the link to my page having the contact form: http://kunalaggarwal.eu5.org/website/home/
Click the Contact button on top.
Thanks in advance.!!
And seriously, very nice tutorial. Thank You!!
@itz_tim_y0: You mean SMTP auth? You can do this with the PEAR Mail package:
http://pear.php.net/package/Mail/redirected
@Matt, cheers for that. Also, how would i put this on a contact page where the form is displayed by default?
Hey Matt..
I fixed the problem that I posted above. It was a small error on my part, I missed the id attribute for the name text field, that was causing the problem..
Now it works like a charm!!
Thanks a lot for this tutorial. Simply amazing!! 🙂
Hey Matt..
Sorry to trouble again, but can you please provide some help on how to include a reCaptcha in this form. I obtained my key from Google for the reCaptcha.
Basically, I need help with the CSS on how to align it properly.
Thanks again!!
@itz_tim_y0: Just take out the JavaScript lines that hide & position the form:
etc…
@KunalAggarwal: Sure, just post your question in a new topic as it’s veering away from the article’s topic somewhat:
http://www.elated.com/forums/authoring-and-programming/topic/new/
Hi great Tut! looks great! works perfectly, on Safari, Opera, Chrome, Firefox..IE 7, on my iPad, android phone, Xoom tablet(yes I bought one)…however, IE 9 is giving problems. It loads on the bottom left side of the page…thnx in advance!
edit oh err – my site http://www.movilenglishhd.com/Que_es_Movil_English.html
[Edited by Mark5555 on 20-Sep-11 13:48]
@Mark5555: Nice site!
You need to use the HTML5 doctype to stop IE9 going into quirks mode.
Awesome! and thnx! I was going nuts with .noconflict() , css ie hacks..
Thnx again Matt!
@Mark5555: No problem – glad I could help 🙂
Very cool, except I’m having a problem when I try adding checkboxes to the mix. I’ve tried a number of things, but this is the way I figure would make the most sense.
I just want the checkbox to appear on the same line right after the text, but when I do this the checkbox literally ends up right on top of the text. I’m sure I need to make some kind of change to the stylesheet, but I haven’t been able to figure it out.
@sjsnider: Try adding something like this to your CSS:
Thanks so much. That was very helpful.
Hi I was wondering if there is an example using a captcha field to prevent non human sending.
I have a site that has this problem so want to use somethng like this cool example.
I also need to bring in another field to send the form to someone else but thats another story 🙂
so thought I would start with the captcha first any ideas?
Thansk for a great article and example
Andrew
I too am trying to use recaptcha with this to no avail. Can I get a little help?
@amcoms: Shouldn’t be a problem. There are many captcha scripts that you should be able to integrate, eg:
http://www.phpcaptcha.org/
There’s also reCAPTCHA which has plugins for various server-side languages and software, as well as a nice Ajax API:
http://code.google.com/apis/recaptcha/docs/display.html
@mydudechris: Sure, I’ll try anyway! What problem are you having exactly?
hi,
how can i do it over flash?
Hi Matt
Firstly thanks for a well written and easy to follow tutorial.
I’m doing a site for free for my children’s primary school, and when I test it on my own websites server it works fine but as soon as i transfer it to their server it goes through the motions and thanks me for sending etc but I don’t receive the email?
I’m guessing that their server doesn’t handle ajax or it’s not enabled – is there anything else it could be?
Thanks again
P.
This is a great tutorial,
I have one problem though, I am not recieving any emails from the form, I have never made one of these before so I am clueless as to where the .php file goes, I just dumped it in with all the others and specified my email address in constants, what am I doing wrong ?
Hi,
I want to make a multiple choice quiz of 100 questions using javascript or php .
i want that when user fill the answer & submit it . The no. of correct , wrong & left answers (including correct answers) & score shown in the next tab .
can anyone help me ? Plz , its very urgent….
Thanx in advance.
This is the best tutorial I’ve ever seen on writing code of any kind. You do it right, Mr. Doyle!
@pixelist: More likely a mail server issue – it’s not getting to your server’s MTA, or the MTA is having trouble delivering it. This is a bit out of the scope of my article I’m afraid. You can post the problem in a separate topic if you like:
http://www.elated.com/forums/authoring-and-programming/topic/new/
@tonyb67: Same answer! The email is likely getting sent by the PHP script, but then the MTA isn’t delivering it for some reason. If possible, check your PHP error log and/or your MTA’s mail log to see if you can narrow down the issue. Do other PHP email scripts on the server send mail OK from and to the same addresses?
@AustinTxous: Thank you sir! 🙂
Hi Matt and Tony B
Thanks for getting back to me. I think it is a server problem – other php mail scripts work fine using the same address so it appears that it doesn’t like the ajax?? On my own server the form functions as expected.
As this is an Education Authority server everything is slow and the Administrator has not even got back to me.
So I’ve gone back to a simple php mailer with redirect for the time being – can’t thank you enough for your help though
Cheers!
P
Excellent work! 5 out of 5 stars! Clean and relatively straightforward to customize.
@pixelist: As far as web servers are concerned, Ajax requests are no different from regular HTTP requests. I don’t think Ajax is your problem. I expect your PHP script isn’t running correctly. You could try adding some debugging to the PHP script to see where the problem is.
You can also try disabling JS in your browser then reloading the page to get the non-Ajax fallback form, then try submitting that. That might give you a better idea of what’s happening to your PHP script.
@jb0063: Thanks for the compliment 🙂
Hi,
I’ve been reading your articles and they have helped me BIGTIME! I really like this lightbox form, its fantastic but I am trying to add a captcha that I use in some of my other forms and I’m getting nowhere! Please could you help me with it!? I can show you what I’ve done so far …
Keep up the killer work!!
@reflex84: Sure, please post your question in a new topic and we’ll see if we can help!
http://www.elated.com/forums/authoring-and-programming/topic/new/
Greetings Matt;
Great tutorial, it seems to do exactly what I’m looking to do. I’ve tweaked a few things to reveal hidden layers rather than simply feeding the info. It seems to work as far as I can tell, but it hangs on the “sending” message and nothing comes through the email.
I have reverted to your php script, thinking that it may have been a mistake in my code, it doesn’t work either. Other php mail scripts work on this server, so I don’t know what it could be. Any help would be appreciated.
the URL is here, still using your demo.
http://betyar.comeze.com/Slick.html
Thanks.
Greetings again Matt;
Update. Don’t know what was wrong, I started over from scratch and now it works.
One thing I was wondering though; I am clearing the form fields on “success”, I was wondering how I could delay that action though. Using the same delay tag for
doesn’t delay anything.
A little help?
@IBetyar: Your URL doesn’t work for me (takes me to a hosting signup page).
You can use setTimeout to create a delay:
http://www.elated.com/articles/javascript-timers-with-settimeout-and-setinterval/
Hope that helps!
Matt
thanx for nice tutorial..
i m trying to add Mobile No. below email ID but i m unable to customize… please help with mobile no.
here is my edited php code for mobile no. 🙁
<?php
// Define some constants
define( “RECIPIENT_NAME”, “Site Form” );
define( “RECIPIENT_EMAIL”, “[email protected]” );
define( “EMAIL_SUBJECT”, “Visitor Message” );
// Read the form values
$success = false;
$senderName = isset( $_POST[‘senderName’] ) ? preg_replace( “/[^.-‘ a-zA-Z0-9]/”, “”, $_POST[‘senderName’] ) : “”;
$senderEmail = isset( $_POST[‘senderEmail’] ) ? preg_replace( “/[^.-_@a-zA-Z0-9]/”, “”, $_POST[‘senderEmail’] ) : “”;
$senderEmail = isset( $_POST[‘senderMobile’] ) ? preg_replace( “/[^.-_a-zA-Z0-9]/”, “”, $_POST[‘senderMobile’] ) : “”;
$message = isset( $_POST[‘message’] ) ? preg_replace( “/(From:|To:|BCC:|CC:|Subject:|Content-Type:)/”, “”, $_POST[‘message’] ) : “”;
// If all values exist, send the email
if ( $senderName && $senderEmail && $message ) {
$recipient = RECIPIENT_NAME . ” <” . RECIPIENT_EMAIL . “>” ” <” . RECIPIENT_MOBILE . “>”;
$headers = “From: ” . $senderName . ” <” . $senderEmail . “>” . ” <” . $senderMobile . “>”;
$success = mail( $recipient, EMAIL_SUBJECT, $message, $headers );
}
// Return an appropriate response to the browser
if ( isset($_GET[“ajax”]) ) {
echo $success ? “success” : “error”;
} else {
?>
Hey Matt;
Thanks for the reply. That page got taken down after I resolved the problem. Sorry.
I couldn’t get the delay to work on closing, so I simply cleared the fields when the user re-clicks to reopen the form. That worked. I will look into your suggestion though, it is definitely more graceful.
I do however have another problem, I have emailed you the details of the problem, as well as the proper link to your email from this site.
Thanks.
@avdhesh: What is the problem or error message that you’re getting?
@IBetyar: Sorry, I only answer tech queries in the forums, not via email. You can post your problem in a new topic if you like:
http://www.elated.com/forums/authoring-and-programming/topic/new/
problem i s when i fill up mobile no. its shows email is not valid 🙁
can anybody provide me code with mobile no. verification please
@avdhesh: You have at least 1 bug in your code.
should be:
still the same
and also mobile no. shud not accept aplha value……. but now its accept aplha….
i notice this while i send the message :-
There was a problem sending your message. Please try again.
@avdhesh: I can’t see any other obvious problems from just looking at your code. You’ll need to add some debugging to your PHP script. e.g. use echo to display the values of variables at various points, and see which variables are (or aren’t) receiving the values from the form.
Hi, im trying too use this script. Only thing is ive changed the buttons from send email too Skicka (Swedish for Send).
But when Ive made all inputs and press “Send” It says the message “please hold while we send the email” and it never sends the email.
If its at any help I use one.com as host and hotmail as recceiver.
Id like if you can send me a tip over email or something. Gonna try look over the code today and see if I can find something. But else I must say this is the nicest thing ive ever seen. Hope you will make more things like this for the community.
WibbenZ
Email: [email protected]
@wibbenz: So you just changed the line:
to:
?
If so then I can’t see any reason why this would break the script.
Try changing it back to:
Does it work now?
Cheers,
Matt
Hi – great stuff- its been working great..Initially I had a little issue making it run on IE, it was a bone head move by me, which you helped me fix..
I fear I’ve gotten too ambitious and got my self into trouble again..I got 4 jQuery plug-in/Libraries working at the same time
Nivo Slider
Lightbox
ThickBox (for videos)
Contact form…
I ve done some jQuery.noConflict(); tinkering to get them all to work, and it works great on Safari, Opera, Chrome, Firefox..except for, ofcourse IE..
its not hiding the form, it displays it on the bottom of the page
if you have some time could you look over my frankensteinian code, and see what might be the issue?
http://www.movilenglishhd.com
THNX again
I Love this!
Is there a way to add Section Drop downs sections with different options to it???
Thanks!
Can anyone answer this question pertaining to this ajax contact form not focusing in firefox when you have embedded divs?
http://www.elated.com/forums/topic/5351/
[Edited by altaone on 05-Dec-11 22:49]
@Mark5555: Try turning on IE Developer Tools (F12) and see if you get any errors in the JavaScript console. If there are no errors, try inserting console.log() calls at various points throughout the script to see what’s getting run, and what isn’t. For example, it may be that an event handler isn’t firing in IE for some reason.
@mylifeinfiction: Thanks! Not sure what you mean by “Section Drop downs sections”. You should be able to add in a <select> menu fairly easily if that’s what you mean?
Yes thats what I meant a <select> menu 🙂
Hello Matt;
So I have been tinkering with the StopPropagation and event.preventDefault click handlers as you suggested. I am new at this and am probably still doing something wrong. I couldn’t get it to work.
I gave up on using that scroller and opted to remove the offending conflicting scroller and am now using a Parallax type BG scroller. It works much better and no conflict.
Thank you for your patience and all of your help with this endeavour.
Hi, Matt!
After working on your tutorial for the whole day I was able to modify the code to fit my web site. It work amazingly! No more hours of digging for code!!!
However, I run into a couple of issues that I cannot resolve and am in need of your help, if I may.
1. When I receive a response from the mail form, in the field “From” I see my own E-mail address and not the name of the sender. Also, when I click “reply” I only see my own E-mail address. For some reason information from fields “senderName” and “senderEmail” getting lost (I attached all the codes below). Any idea what am I doing wrong?
2. I installed HTML code in one of the main content window of my web site (www.25bits.com). Everything works OK only if the “contact us” hyperlink is located in the same DIV. How can I move the hyperlink to any other place on the website?
Steven
HTML code:
PHP code:
and I haven’t changes anything else.
Thanks for answering my other question. You’ve been great. as is the site.
I have one more as I am really new at PHP.
Would the following code be correct if I want to add a phone field? I found this online.
Great – Thnx again!! 🙂
@mylifeinfiction: Cool, just add your <select> element and style it in the CSS. You can read its value in your PHP handler just like the other fields. eg:
and…
The value of the selected option will be passed to $_POST[‘mySelect’] in the PHP.
@IBetyar: Glad you got a solution in the end 🙂
@stevenmahoney:
1) It’s because you’ve commented out the correct line of code:
and replaced it with:
…which will send the mail from your email address instead (RECIPIENT_EMAIL).
2) What happens if you move the hyperlink outside the div? Can you post the URL of a page that has the problem you mention?
Cheers,
Matt
I tried to add some spam measures and they did not work properly.
anyone know how to fix?
I also could not get it to truly check for proper email format using:
I input about 20 random alpha characters and it still got through in the email area as did 3 urls in the msg area.
Thanks
@altaone: No, I doubt that would work. This isn’t even valid syntax:
I assume the other block of code is to filter and reformat phone numbers? Looks like it should work, although you don’t want to return the results of preg_replace(). Assign them to $phone instead.
hello and thank you very much for this tutorial,
i hope that you’ve got time to respond to this stupid question
If for example I’m adding a field for phone number like this:
What i have to change into processForm.php, if i want to receive this phone number into the message body .
sorry for my frenchglish and thanks
@altaone: This topic is for questions about the code in the tutorial. If you need help with an anti-spam system, please post your question in a new topic, with a description of any error(s) and the URL of the problem page:
http://www.elated.com/forums/authoring-and-programming/topic/new/
@truitas:
etc…
thanks for this quick response, it’s working well….
Hi,
I hope you can help. I’m trying to incorporate this excellent code into a website i’m working on.
I have jquery working to rotate images in the header of the website. However when I click the email us link, the form appears under the rolling images?
Also when I click Send Email, it says “Sending your message. Please wait…” but nothing happens from here?
Any ideas? heres the link: http://d1358011.ali41.something.ie/
thanks
Matt?
Hi dude.
I have a weird issue.
Those lines doesn’t work at all:
I don’t understand since if I ask to show it instead of hide the form when the page loads, it works.
It works too if I put $(‘#contactForm’).show()…
(note that #centre is my content wrapper)
I can see it and it works.
#center #contactForm are not fading at all and the form is not being displayed. ???
I’ve no more ideas now to fix that issue.
Do you have one?
Thanks for your reply, Matt.
[EDIT] Oops. Forgot to tell .positioned class is never added to the form. Even in your demo.
Do I misunderstand something?
[EDIT 2] I’ve tried with your jQuery link (mine was using the latest] with the same result.
As I made working easily on a stand alone page a few month ago, I don’t see why it can’t work in the CMS I use (which is using XML for storage instead of a database).
[Edited by Sventovit on 16-Dec-11 09:50]
@spencer: I couldn’t view your URL (“Error establishing a database connection”). Also, might be best to post in a new topic since your question isn’t about the original tutorial code:
http://www.elated.com/forums/authoring-and-programming/topic/new/
@Sventovit: Please post the URL of the page with the problem, so we can see it in action.
Hello Mr Matt
thanks a lot for having replied.
I just sent the files and now the whole enchilada is online.
This is the URL of the blog home page :
-http://sventovit.fr/logbook/
The box should open when clicking on the #contact menu in the nav section. (-http://sventovit.fr/logbook/#contactForm)
I’m really sorry to annoy ya with such an issue. I’ve spent about 2 hours to make it work and nope: I just still can’t see where my error is.
Maybe you’ll find it…
Thanks again.
Phil
This tutorial is awesome, can you tell me how to make a zip file download when the form is submitted.
I assume it would be some kind of if statement in the PHP, but I am not sure exactly what to say there.
It would be very helpful if you could tell me how to do that.
Thanks a lot for this nice submit form!
I have one question, how can i refresh DIV ‘content’ when de form is succesfull submittet?
I figured it out, I just put:
window.location.href = “images/download/file/name”; in the if/else statement at the end. Works perfect now.
VERY nice form!
I have a question: the #incompleteMessage seems to be unused, it will never appear in any situation. Instead, an image pop up with the words “please fill out this field” will appear if I leave anything blank. This occurs for both your demo and after I implemented it in my website. I don’t really mind as I think “please fill out this field” right below the field that needs filling is more useful, but I can’t seem to change this message (can’t find anywhere within css/html/php file that produces this pop-up).
Thanks for your help!
i have a same problem like windbrand ;
how can i change the “please fill out this field” phrase ?
and i want change the css of this phrase. please help . its necessary
Hi Matt
what should I do now?
@Sventovit: First thing that springs to mind is that your Contact link’s href attribute is:
“http://sventovit.fr/logbook/#contactForm”
But you’re trying to select it with:
$(‘a[href=”#contactForm”]’)
I’d wager that jQuery’s selector engine sees these as 2 different URLs.
Try:
$(‘a[href=”http://sventovit.fr/logbook/#contactForm”]’)
instead.
Hi Matt
thanks for having replied. I much appreciate.
Your suggestion does it… on the blog home page and only on it.
If the visitor lands on an article page, the URL won’t be the same (eg ../logbook/article15/balisage-semantique-gabarit-html5-over/#contact) and the contact form won’t be displayed.
Weird, isn’t it?
Does that mean your script will only work on a “stand alone page”?
Regards,
Philippe
EDIT: there was a typo.
[Edited by Sventovit on 06-Jan-12 06:24]
hi,
your form is working well, but i’ve got some problems with special characters: ‘ à , é, è, ü etc .
Fore example the word l’activité will appear like this into the message body: l’activité
How i can solve this?
thanks
By saving the php file “processform” in UTF-8 without BOM maybe?
thanks, but i think that’s a problem between utf8 and iso, i found this:
the page displays the characters of this type: “Ã ©”, “Ã ®”, “A” …
=> The data was saved in UTF-8 format = the browser think it is
ISO format.
the page displays the characters of this type : “�”
=> The data was saved in ISO format = the browser think it is
UTF-8.
PHP natively work in ISO, it will switch entirely to UTF-8 after v6.
@windbrand: What you’re seeing is your browser’s HTML5 form validation, triggered by the “required” attributes in the form’s fields. It is browser-specific. As far as I know, you can’t alter the message that the browser displays. #incompleteMessage is used as a fallback if the browser doesn’t support HTML5 validation.
If you’d rather just use the #incompleteMessage validation at all time, remove the “required” attributes. Personally I’d use the HTML5 validation if the browser supports it.
@Sventovit: I would try making all your Contact links just point to the URL:
“#contactForm”
Then the URL will be the same on all documents, and you can select the link with:
$(‘a[href=”#contactForm”]’)
You will of course need to include the contact form JS in every HTML document in your site.
@truitas: Make sure you use UTF-8 encoding throughout the whole chain: include <meta charset=”utf-8″>in the HTML document; serve the document with the “Content-Type: text/html; charset=utf-8” HTTP header; and set the MIME content type in the email body to “text/plain; charset=utf-8”.
This is the original code which doesn’t work Matt.
@Sventovit: I don’t understand your response. Are you saying there’s a bug in the original code?
You need to include the JavaScript and markup for the contact form on every page where you want to use it.
I meant “which doesn’t work” on dynamic URL.
I can’t say there’s a bug in the original code as I’m not skilled enough. I know it’s working on a single page but there’s an issue with such URI.
Hello Matt,
Thank you for the post it is really interesting and well explained. I was able to make it work alone, but now I am trying to implement the ajax animation in another form. Trying to get the notifications and fadein, fadeTo effects.
But I am having problems with this part of the code:
// Return an appropriate response to the browser
if ( isset($_GET[“ajax”]) ) {
echo $success ? “success” : “error”;
} else {
?>
<html>
<head>
<title>Thanks!</title>
</head>
<body>
<?php if ( $success ) echo “<p>Thanks for sending your message! We’ll get back to you shortly.</p>” ?>
<?php if ( !$success ) echo “<p>There was a problem sending your message. Please try again.</p>” ?>
<p>Click your browser’s Back button to return to the page.</p>
</body>
</html>
<?php
}
Im always getting the answers:
“There was a problem sending your message. Please try again.”
how can i check where is the problem, I know its not a precise question, but maybe you can help me.
what is the difference between?
define(‘IS_AJAX’, isset($_SERVER[‘HTTP_X_REQUESTED_WITH’]) && strtolower($_SERVER[‘HTTP_X_REQUESTED_WITH’]) == ‘xmlhttprequest’);
and
if ( isset($_GET[“ajax”]) ) {
echo $success ? “success” : “error”;
} else {
Why and when use each one?
Thanks again
This is the message to all participants and guests of this blog. After spending a couple of months playing with the code provided here, I was able to figure out why my code did not work and I was able to fix it.
Here is what the problem was: server restrictions. It appears that different sites impose different restrictions or rules as to how PHP handled. (some do not allow “mail” command, some restrict the way you define functions, some impose an obligatory code you have to use, etc.) Absolutely same code would produce different result, depending on your web server.
After pulling my hair, I began shopping around and was able to find a free web hosting, that has very few restrictions. And, voila! – the code works without a glitch!
I hope this message will help others and eliminate frustration.
I am not a professional coder, so, please excuse me if I sound like an amateur. 🙂
Steven
@Sventovit: I’m not familiar with how your site navigation works, but in theory there’s no reason you couldn’t use the contact form script on your site. If you’re using some JavaScript code for your navigation then you might need to rewrite some of the contact form code to play nicely with it. I’d guess that there’s some conflict between the contact form code and your navigation code.
Try adding console.log() throughout the contact form code to see which bits (if any) are being run when you click the Contact link.
@bernardao: Could be a number of things. As @stevenmahoney points out (thanks Steven!) it could be a server restriction. It might also be that the PHP script isn’t getting the data correctly from the form. See here for some troubleshooting tips:
http://www.elated.com/forums/topic/5171/#post20765
HTTP_X_REQUESTED_WITH refers to the X-Requested-With header sent by most JS frameworks along with an Ajax request. It does much the same thing as my code, but it does depend on the framework sending the header (and the web server allowing PHP to access the header). More here:
http://stackoverflow.com/questions/2579254/php-does-serverhttp-x-requested-with-exist-or-not
Mr Matt?
Hello.
I didn’t find the reason of this issue but I fixed it by changing the onclick call this way:
$(‘a[href=”‘+self.location.href.split(‘?’)[0]+’#contactForm”]’).click( function() {
And now, tada! It works everywhere.
Thanks a lot for your patience and kindness.
I really appreciate.
Oh! I forgot to test the sending and it fails.
I’m having the message:
“There was a problem sending your message. Please try again.”
Is it due to my code?
@Sventovit: See here for some tips for troubleshooting the mail sending:
http://www.elated.com/forums/topic/5171/#post20765
It’s a bit more complicated since it works on the blog home paginated page and not on a the single page of an article.
And there I don’t see why the form doesnt’ work.
@Sventovit: Put some debugging in your PHP script to see what data the script is receiving from the form. Then see what happens when you post the form on both the blog home page and on the single article page. Does the PHP script receive the data correctly in both cases?
Hey everyone,
I am new jquery(new as in one day old :D). I cannot get this working even if I just download the code and modify the PHP file so that it has my email it does not work. So help? I have a gmail email address.
Thanks.
@isheets: You need to give more information – what doesn’t work exactly? Do you get any error message?
Hi Matt!
Thanks for posting this wonderful tutorial!
Because I’m using a form with many more inputs (and for extra security), I prefer to use Tectite’s FormMail script for handling the form.
However, one major disadvantage of Tectite’s FormMail is that it doesn’t support AJAX form submission out of the box. I spent a few hours integrating your JQuery script with Tectite’s FormMail and thought I’d share the result for anyone else interested!
I just inserted the AJAX browser response lines before the FormMail script redirects to the Thanks page. In my FormMail.php file, the code to redirect is on line 13482
With your AJAX browser response code inserted, the code now looks like this:
I’m also using my own Thanks page instead of Tectite’s auto-generated one, so I inserted the AJAX browser response code before the Thanks Page template is called.
I also used the jQuery serializeArray() method instead of serialize() in order to include hidden inputs from the form, which is crucial to the functionality of FormMail.
It took me a few hours to figure it out, and since I got it working, I thought I’d share with others!
Hello Matt
thanks again for the support.
I’ve tried this weekend to “debug” and only found this error when trying to send a mail using the form on a single article page:
A session had already been started – ignoring session_start()
Hum…
Matt this is an awesome tutorial and i think you done great job!! I tested the form and it works like a charm. 🙂
One thing I want, if you can help me! I’m trying to fill the form with greek characters and the message appears weird. I scroll the messages and i saw that maruse also had the same issue with Japanese and he solve it! Unfortunately I don’t know how to use the strip_tag(). If you can post a solution about, I will appreciate a lot!
Also, is it possible to write at fields John Smith and Visitor Message something in different language and appears correct??
Thank you!!
@kimbo6365: Great tip – thanks for posting 🙂
@Sventovit: Hmm, not sure. I’d have to see your whole PHP script. I assume you are calling session_start() in the script for some reason?
My guess is that, since your PHP script works on one page and not another, there’s a problem in the second page (either in the form JavaScript, the markup, or some other conflicting JavaScript) that is preventing the data getting to the PHP script.
@apokyro: Try just adding the ‘u’ flag to the calls to preg_replace(), as I describe here:
http://www.elated.com/forums/topic/5171/#post20818
Also make sure the HTML document containing the form uses UTF-8 encoding:
Hey,
first thanks for this great script!
I added an extra field for a telephone number and a checkbox where the user can request to be called back.
Is there any possibilty for set the telephone-field to “required” when the checkbox is checked?
thanks for you help. 🙂
@68bydesign: Not using the HTML5 required attribute, but you could do it with JS inside the submitForm() function. eg:
etc…
Hey Matt!
I followed your tutorial but I’d like to expand on it a little more to allow attachable files and a few other fields. Can you help me do this? I am having quite the time figuring out why it isn’t working for me.
My HTML
And my PHP which doesn’t seem to grab my items and attach them.
@delvok: If you’re sending form data from a ‘file’ input type then you need to add the attribute enctype=”multipart/form-data” to your <form> tag.
Also it doesn’t look like you’re capturing the uploaded data in your PHP script. You need to open the file stored at $_FILES[‘senderResume’][‘tmp_name’], read its contents, and attach it to your email message using something like the PEAR Mail_Mime package (give it a MIME type of “application/pdf”).
More info:
http://www.php.net/manual/en/features.file-upload.post-method.php
http://pear.php.net/package/Mail_Mime/docs
This is a wonderful little tutorial, thank you for sharing it. It’s very well written and easy to follow. I have asked my fiance to buy me the book!
I read the whole thing from start to finish and did all the steps in order with no problems. It was a complete success and I’m now looking at ways to incorporate this into a project I’m working on.
One question:
Do you have any other tutorials that would explain to me the basics of how to add conditional fields to the form? (i.e. if field A = ‘yes’, then additional fields are hidden/shown).
Or if it’s not too much trouble, could you provide me with a basic example using this tutorial as a reference point? I catch on fairly well, so a code snippet would do.
Thanks again 🙂
EDITED:
I’ve done a bit of Googling and (after a bit of tinkering) solved my own question by applying the logic of some code explained in this other post –> http://anthonygthomas.com/2010/03/14/display-form-fields-based-on-selection-using-jquery/
Here is the the code I have put together, based on the original tutorial given above to demonstrate conditional show/hide fields within the Slick Ajax Form –> http://jsfiddle.net/r262B/1/
Happy days 🙂
[Edited by BunnyBomb on 21-Feb-12 10:08]
What an excellent tutorial. Thanks for the good work!
I implemented the contact form into my website, and all seems to be well except for a few minor things.
http://www.tosstemple.com
When I added the code for the contact form, the background I set for <html> seems to vanish. The first css rule pertains to setting the background to a full image, and while it worked prior to adding the contact form, it seems to now be in conflict.
Is there anything you guys see that could be blocking the background image from appearing? Any help would be greatly appreciated!
Thanks!
P.S. on an unrelated note, I’d also like to center images/finalbackground.jpg, but all the various css positioning techniques I’ve attempted have resulted in no dice. Any suggestions?
-J
I’m no expert but I took at look at your CSS and noticed a couple of obvious problems.
You have <style> tags within your CSS file, which seemed to make it about as useful as a ginger-haired-step-child. Also, the background image is being added to the <html> tag, which I’m told is not ideal. IE doesn’t understand this, so it’s best to use your <body> tag instead.
Here is a new version of your CSS file that does what you want:
http://pastebin.com/7GuQtW5Q
Lastly, the way you had included conditional statements at the end of your main CSS file won’t work. I recommend creating a special file called “IE7styles.css” then adding code along the lines of this into your <head> tag within your html file:
I’m no coder, but it’s amazing what you can learn with a bit of fiddling around using Firebug.
Cheers
PS: I recommend adding a blank “index.html” file into your /images folder so folks can’t see all your gibsons.
[Edited by BunnyBomb on 22-Feb-12 11:09]
Is it possible to modify this so that there are 2 different contact forms on one html page. I need one that is just a generic contact form like the demo to send to [email protected] and another contact form on the same page with one additional field (drop down field) that sends an email to [email protected]. Any advice on how to accomplish this would be greatly appreciated.
thanks
Ahh, thanks so much BunnyBomb. Your advice helped me greatly and now everything is looking just peachy.
I hadn’t heard of Firebug before, but will be sure to check it out.
Also, for the sake of learning, what does adding a blank ‘index.html’ file in the /images folder do such that it hides my gibsons?
-J
Glad to have helped.
Yeah I use a couple of things now, after doing some reading. I use two Firefox extensions called Firebug and Web Developer. Firebug is great for inspecting CSS via right click, to work out what the hell is going on. Web Developer is great for editing the CSS to generate a new CSS file (like I did for you in like…2 mins).
As for the blank index.html – before you added the file I could navigate directly to ‘http://www.tosstemple.com/images/’ and browse through every image file you had in that folder, save them down, etc. It’s just best practice not to make it easy for folks to copy all your media in one click. Glad to see you added the file.
Best of luck 🙂
So here is an example of what I’m trying to do. http://twistedsisterscupcakes.com/Contact2.html
I can get the 2 different forms (contactForm, contactForm2) to pop up and fade out but neither will send an email. It says there is a problem sending your message. I moved the jquery from the html page to specialorder.js and contact.js. Does processForm.php need to be in the same folder as my Contact2.html? I have it in a different folder (php).
@BunnyBomb: That’s a nice solution for adding conditional fields to the form – thanks for posting it 🙂
@dzoid11: It doesn’t matter where your processForm.php script is, as long as the paths to processForm.php in your form tags are correct.
See my previous hints in this thread for debugging the email sending (search the page for ‘debug’).
Matt
@matt thanks.
I’ve got the first contact form working but the second one says “please complete all fields in the form before sending” even though all the fields are filled out.
I added ?ajax=true to the form action and turned off javascript in my browser. The first form returns “Thanks for sending your message! We’ll get back to you shortly.” and successfully sends the email. The second form returns “success” and successfully sends the email.
Any ideas on why the second form would send an email when java was turned off but not when it’s turned on?
http://twistedsisterscupcakes.com/Contact2.html
Hi matt,
great stufff love it,,
also i have a question,, how to change tooltips “Please fill out this field” and “Please enter an email address” i can’t find out this text on source,, am noob for this,, any help would be nice,,
Thanks,
@dzoid11: You can’t just duplicate the form in the page, using the same id attributes, and expect the JavaScript to know which form it’s working with. id attributes have to be unique in a document. Once you have unique IDs you’ll also either need 2 different contact form scripts to work with the different ids, or modify the one script to accept a base id (eg “contact-” and “special-order-“) that it then uses to determine the ids of the form fields it needs to work with.
@adoet_t: I already answered this earlier in the thread.
Hi Matt,
Superb contact form, I’ve looked at many, and this was by far the best and easiest to implement.
I used it on my wife’s site here:
http://namaste-naturaltherapy.com
It’s working very well, but she is getting a lot of spam from bots,
Is it possible to implement some kind of anti spam measure, like a captcha?
Thanks
[Edited by Moog on 04-Mar-12 15:28]
hello matt…
Thanks for the amagind script… i am using your form for getting details from the customer before downloading the pdf.. so naturally no change in form page.. but when it throws to processForm.php i have to send details in database. that i done. but after that i hv to send pdf file to user for save. it works but ont in scusees mode.. and form is still appearing on the page..!!! 🙁 one more thing pdf path is also come from database by the select query on the same page i.e processForm.php i dont required to send any email
here is the code for my processForm.php
i hv to submit this projet in my college so plz help me solve this matter as soon as possible thanks a lot…!!! 🙂
if u hv any doubt feel free to ask me…!!!
Akil Shaikh
[Edited by akilshaikh on 08-Mar-12 22:58]
@Moog: Have you tried reCAPTCHA? Should be fairly easy to integrate if you generate the contact form page in PHP:
http://www.google.com/recaptcha
@akilshaikh: The contact form JS expects an Ajax response (success/error), so sending back a stream of PDF data isn’t going to work. I suggest you modify the JS so that, once it receives a “success” response, it redirects to your PDF:
This can either be the actual PDF file, or a PHP script that serves the PDF data.
Thanks Matt
thanks for quick reply.. but still i am little bit confuse. where should i write this code.. in jquery.min.js file
or
in my processForm.php page. after my insert query.
And after applying this i hv to write the php code for download file?
i try after insert query. it loads the pdf but in same the page. and not prompt to save .pdf file and user have to click back button of browser to go back to site.. u know its not user friendly.. so plz give me some hint about where should i have to write this code. i hope i am not bothering you.. i am littlebit new to js. so don’t wont to take any-type of risk with the js file.. 😉 hope u understand that….
thanks a lot for your quick response
Akil Shaikh
[Edited by akilshaikh on 10-Mar-12 00:59]
Thanks Matt, it looks easy enough, though it will need some restyling to suit
Firstly what a slick form this is. I got it working quite promptly but when I validated it I got the following error, right over my head opinions would be most welcome. Best wishes Nick
W3C Mark up validator
Validation Output: 1 Error
Error Line 301, Column 75: value of attribute “type” cannot be “email”; must be one of “text”, “password”, “checkbox”, “radio”, “submit”, “reset”, “file”, “hidden”, “image”, “button”
…ail Address</label> <input type=”email” name=”senderEmail” id=”senderEmail” ma…
✉
The value of the attribute is defined to be one of a list of possible values but in the document it contained something that is not allowed for that type of attribute. For instance, the “selected†attribute must be either minimized as “selected†or spelled out in full as “selected=”selected”â€; a value like “selected=”true”†is not allowed.
[Edited by nicallen on 15-Mar-12 07:14]
Hello and nice work Matt!
is possible to offer us a little tutorial how i can use it in wordpress?
I’ve try allots of tricks but no results…
I’ll be so happy if you can explain a bit 🙂
GJ!
Hi, i was wondering if you could advise on a small piece of code or link to incorporate this form to an existing website for instance i have different contact options on my site like ones an image then there is some text id like to link it too.
@akilshaikh: In the JavaScript in your contact form page. Modify submitFinished() to redirect to the PDF URL if a “success” message is received. You don’t have to use PHP; just upload your PDF file somewhere and let the web server serve it to the browser.
Thanks for quick the reply Matt, unfortunately I’ve been trying without much success i know its probably very simple but i haven’t been able to get it right could you please past the code for me and direct me were to slot it, otherwise i want be able to use this form for my website and i have to be honest I’ve seen a lot of contact forms but this is the mother of them all and really want to use it.
Hello Matt..
First of all lot of thanks to you coz even in your busy schedule u spare time for solving the issues of people..
Now coming to my issue.. after your first solution i.e.
i used it in my processForm.php like this
Now when i apply this code pdf is downloaded successfully but it doesn’t want prompt to download and still open in same page. now you can see that this code work in else portion. so naturally success part is not working u can look at my site how i customize your form for my site here is the link
http://elevatormachine.in/productdetails.php?id=2
click on download pdf button u will understand what i want to achieve.
Now in your second reply u said that no change in php file. and change must be in JavaScript.
Now try to understand the flow of this from pdf path is static but pdf name is dynamic it comes from database with the help of product id. now when i use your code
Now what happen in this case form is simply display in product details page instead of dynamic display. i mean it appear bottom side of my product details page.(hope u got it)
Now one more thing when i remove message field from the validation i can’t get the value of email in database. (it’s strange for me).
Hope u are clear with this. If u have any doubts plz feel free to ask me.
so kindly help me for about this if u give any kind of hint or suggestion for solving this..
Lot lot thanks to you
Akil Shaikh
[Edited by akilshaikh on 22-Mar-12 02:06]
hi, great tutorial ive imbedded the model into an module in cmsms, and i thought id finished – but then realised that the validation processes on both sides client and server dont seem to work in IE7-9. your tutorial version does not either so I wonder if you have any ideas ?
javascripts are obviously running because the fadeouts and hiding work, as well as the ajax call and email processes, but in ie i can fill in garbage in the email field (the only field im using) and it passes right through to email, in chrome, firefox etc all works as expected
be very grateful for a suggestion
and thanks again for a great tutorial !
@nicallen: Sounds like you’re not using the HTML5 doctype, and/or you didn’t select the “HTML5 (experimental)” option for the Document Type option in the validator.
thanks for prompt response, actually I have used the correct doc type but if i had not – that would not explain why your demo run from your site does not work either ! is there perhaps an issue with windows 7 and ie and the tutorial? i have tried running your demo from both a pc and a laptop and both allow rubbish through the email field and behave as though it is successful – running your demo from your site (eg here) in all other browsers seem to work as expected and the rubbish is stopped.
sorry to persist – it would be hugel-y useful to resolve this whatever the reason! many thanks
@Ferris: If you post the URL of your contact form in a new topic, as well as exactly what you want to achieve, then someone may be able to point you in the right direction:
http://www.elated.com/forums/authoring-and-programming/topic/new/
@akilshaikh: You need to modify the submitFinished() JS function to do the redirect. You can’t spit out the JS from your PHP script like that. The contact form JS expects an Ajax response string (“success” or “error”). If you need to redirect to a dynamically-generated URL then I suggest you get your PHP script to return the URL in the Ajax response. Then modify submitFinished() to treat a response string beginning with “http://” as “success”, then just do ‘window.location.href=response’.
@bruceleigh: The fallback JS validation (for non-HTML5-aware browsers) only checks for non-empty fields. It doesn’t validate the email address. If you want to validate an email address in JS see: http://stackoverflow.com/questions/46155/validate-email-address-in-javascript
duh! completely forgot about the browser validation issue – ill be off to do some coding then!
interesting disussions in that stackflow reference – many thanks
well… i think no chance for a bit support to use it in wordpress…
/sad
Hi Matt!
Thank you for this wonderful tutorial 🙂
I have a question regarding the PHP form mailer and email syntax.
I would like to also add the dot com (org, ee etc) there too because at the moment it sends the form even if I just enter m@m in the email field but it should only work when I must enter [email protected] for example.
This is the line you have
I have tried different options but none of them work. For example I tried this
I am not a programmer but I am learning 😉
@littlefox: Sounds like you want to validate your email address field to ensure it contains .com. ,org etc, yes? The line of code in the PHP script is for filtering, not validation.
Your best bet is probably to add some validation to the JavaScript submitForm() method – something simple like this should do it:
This adds a regex to the validation to check that the email address ends with a dot, followed by between 2 and 4 letters.
Hi, and thank you for the great tutorial!
I’ve translated this source code to Persian but I have a little problem with it!
The problem is when I write a Persian name (ex: امین) it doesn’t send the email! but if I add an English word to it like “امین2” it sends the email by the name of “2”!
Can u help with this?! what is causing this?!!
Thank u again!
Regards!
[Edited by amin on 10-Apr-12 22:06]
Hello, it’s the best contact form i have seen on the net. Anyway I’ve got a problem.
I was read almost all posts here but I didn’t found my problem.
How it look’s like:
After “send” if it is success you should get msg “thanks, sending etc” and on other case “fail”.
In my case, after click “Send”, mail is sending to recipient email, but look like my php files doesnt not send success command to ajax in html file and on screen is display second case “fail” – “There was a problem sending your message. Please try again.”
Php on my server should work good and I think it is, cuz it sending email. But i have no idea where the problem is.
Here’s link with your contact form on my server.
http://cactusek.home.pl/contact/index.html
hello,
First thing, great job on this tutorial!
Second thing, i dont know if its just me, but i cant seem to get recaptcha working with this form. i tried it with only the php script and it worked fine. But when javascript is beetween something goes wrong.
Is there anything i need to add to this javascript to get it working?
Ok i found out whats the problem so my post is solved.
Maybe it will helps other guys.
I’ve got statistics on my server which were add script on the bottom code that php could send msg back to jquery form script.
Greetings
ok so u say that for using unicode characters we have to use a “u” flag.
Can you please explain exactly how should we write the preg_replace function here with the “u” flag and the whitelist that you mentiond? cause I cant make it work!!
thanks!
Hi Matt, I added this form to a site last year and it worked great and looks great. The problem is the form has stopped working. There has been some content added over the last few months so its possible something has been changed but I can’t find it.
The form is at http://www.listertrack.co.uk, when you click to submit you are left with a message that says “Sending your message, please wait”. The email is not coming through and that message stays on the screen until you refresh.
Could I please ask that you point me in the right direction to solve this problem.
Many thanks
Dave
@amin: I don’t know the Persian character set or how it works with regular expressions in PHP, so I’m not sure exactly what you’d put in the whitelist. But essentially you want to add the range of all allowable Persian characters (the Persian equivalent of A-Za-z0-9).
@aliveit: Try turning off JavaScript in your browser then reload the page and submit the form. See what happens.
Hi Matt, I used this excellent tutorial for my website but I have several problems with Internet Explorer. The first is that not any text appears on the labels of the placeholder. The second problem is that when I send an email even if it is poorly written and without the “@”, continues to send the email.
This is the link to my website: http://www.kromweb.com
I do not know that I have to do to fix it. I would appreciate your help.
thank you very much
@vassilij: IE <=9 doesn’t support HTML5 placeholders. I believe support is coming in IE10. I covered email address validation earlier in the topic: http://www.elated.com/forums/topic/5171/#post22571
Hi Matt, thanks for answering.
I’m not a programmer and do not know javascript or php.
I looked at the comment that you say but I can not make it work.
I’ve been looking online and found a plugin to validate html5 forms but does nothing.
Is as follows: http://www.matiasmancini.com.ar/jquery-plugin-ajax-form-validation-html5.html
I do not know as I have to do to make the form work well in Internet Explorer, I’m going crazy .. lol
I send my code to see if you can help me.
<?php
// Define some constants
define( “RECIPIENT_NAME”, “Name” );
define( “RECIPIENT_EMAIL”, “[email protected]” );
define( “EMAIL_SUBJECT”, “Asunto del Mensaje” );
// Read the form values
$success = false;
$senderName = isset( $_POST[‘senderName’] ) ? preg_replace( “/[^.-‘ a-zA-Z0-9]/”, “”, $_POST[‘senderName’] ) : “”;
$senderEmail = isset( $_POST[‘senderEmail’] ) ? preg_replace( “/[^.-_@a-zA-Z0-9]/”, “”, $_POST[‘senderEmail’] ) : “”;
$message = isset( $_POST[‘message’] ) ? preg_replace( “/(From:|To:|BCC:|CC:|Subject:|Content-Type:)/”, “”, $_POST[‘message’] ) : “”;
// If all values exist, send the email
if ( $senderName && $senderEmail && $message ) {
$recipient = RECIPIENT_NAME . ” <” . RECIPIENT_EMAIL . “>”;
$headers = “From: ” . $senderName . ” <” . $senderEmail . “>”;
$success = mail( $recipient, EMAIL_SUBJECT, $message, $headers );
}
// Return an appropriate response to the browser
if ( isset($_GET[“ajax”]) ) {
echo $success ? “success” : “error”;
} else {
?>
<html>
<head>
<title>Thanks!</title>
</head>
<body>
<?php if ( $success ) echo “<p>Gracias por enviar su mensaje. Le responderemos lo antes posible.</p>” ?>
<?php if ( !$success ) echo “<p>Ha habido un problema al enviar su mensaje. Por favor, inténtelo de nuevo.</p>” ?>
<p>Pulse atrás en el navegador para volver a la página anterior.</p>
</body>
</html>
<?php
}
?>
Thank you very much.
[Edited by vassilij on 20-May-12 16:54]
Matt,
Thanks for this tutorial! It really is great. I have one problem with the form.
The message sends successfully but the contact form doesn’t reappear after sending and doesn’t come back if the cancel button is pressed.
I’ve looked over the code and it looks like all the fade ins and outs are correct. I can’t figure out why the form doesn’t come back.
Here is a link to my website : http://charlesjulianj.aisites.com/imd311/index3.html
UPDATE: I figured it out. I’m not sure if it was the best way to bring the contact form back but I added fade in to the following code
[Edited by cjdesigns on 24-Jun-12 15:32]
Where shall i change the email address to send the emails to?
Thank you!
*Edit: Found out! Sorry for the silly question!
[Edited by enodia on 29-Jun-12 04:13]
why i always get fail to send the message
i only change the
define( “RECIPIENT_NAME”, “xxxx” );
define( “RECIPIENT_EMAIL”, “[email protected]” );
define( “EMAIL_SUBJECT”, “xxxx” );
is there other value I need to change?
I always getting fail with the php contact system 🙁
Hey guys,
I need your help for a project I am working on, I believe it’s nothing to hard, but I am a newbie with PHP and don’t know how to solve it on my own.
So, I have followed the tutorial and now I have my form working, but if I try to put Chinese characters in the Name field (so in php is in the header), it becomes impossible to submit the form…
I have looked online, but I haven’t been able to understand how to encode stuff in to charset utf8 or something.
Your help would be greatly, hugely, immensely appreciated.
Thanks, and again thanks for such a wonderful tutorial.
Ste
@neutronium: What sort of fail? What error message are you seeing exactly?
@steffo91: What do you mean by “impossible to submit the form”? You’ll probably need to modify the appropriate regex in the PHP to allow for Chinese characters.
not the problem with your php.
actually, i can’t send mail though php…
i using appserv and start server with my home computer
i want to use gmail as smtp, but it was confusing
@neutronium: Sending gmail using SMTP auth: http://stackoverflow.com/questions/10380051/how-do-i-send-email-using-gmail-through-mail-where-do-i-put-the-password
If i put checkboxes into the the form, how would I set up the php to pull the info of which ones get checked?
thanks
@mcall: See http://www.html-form-guide.com/php-form/php-form-checkbox.html
hi matt and everybody,
some month ago i used your form and i forgot to show you the result.
if it can help somebody it’s here:
http://savoie-rando.fr/contact.html
thanks
yan
Of all the forms that I have seen, this example is the best…hands down! I love the way it works. However, here is my question:
When you cancel the form and you have entered data, there is a line of code that clears the text fields. Here it an example:
$(‘#someField’).val(“”);
That works great for a text input field. However, the form that I am working on has a select statement. For example:
<li>
<label for=”typeOfPet”>Favorite type of pet:</label>
<select name=”typeOfPet”>
<option value=””>Select…</option>
<option value=”D”>Dog</option>
<option value=”C”>Cat</option>
<option value=”B”>Bird</option>
<option value=”R”>Reptile</option>
<option value=”O”>Other</option>
</select>
</li>
When I cancel the form, how do I reset the value for the input field? I tried:
$(‘#typeOfPet’).val(0);
to select the first option value. I have learn an incredible amount of coding with this example for which I am very grateful for but I am stuck on how to reset the value because after I cancel the form and then reopen the form, I still see the selected value that was chosen before I hit cancel.
After MANY hours of searching, I stumbled upon how to clear the fields. The method that seems to work is as follows:
$(‘#petForm’).get(0).reset();
Where #petForm is the name of the form and get(0) is the very first field within the form.
Nice form Matt. I’ve just ironed out a few problems I experienced while trying to work it into my existing site and thought I’d share a few things I had trouble with, and how I solved them. A lot of trying to run before I can walk here!
First it was difficult to get the thing to size properly so I had to tweak a few things in my existing CSS to get the form to display at the right scale.
Secondly while developing on my own machine (localhost) I was having a problem with the name and email boxes appearing as different widths – but only in Opera. It turned out (after much head scratching) that the php warning I was living with while working on my local machine (it was a SimplePie warning that the cache folder wasn’t writable) was somehow throwing the form fields out in Opera. They worked great in other browsers but it was only when I decided to get rid of the warning message at the top of the page that Opera decided to play ball.
Thirdly (Opera again) I had a problem with the italicised characters getting “chopped off” in the form header. This is something to do with Opera not liking fonts being italicised when there isn’t an italic version in the font itself (or something like that). Again I was trying to get it to work with the font I was already using on the page (Tahoma) which worked in every other browser than Opera. Changing the font-family back to what you have in the demo cured the problem.
Last but not least I also experienced the problem noted by @aliveit where the error message stayed on the screen instead of fading out and returning to the form. Apart from not actually having a #content div on my existing page (I had one called wrapper which I eventually renamed to content) it turned out that the real culprit in my case was simply that I was already using jQuery for something on the page, and I was trying to use that instead of the one which you have in the source code. When I switched to using that everything worked 🙂
Hope this helps someone.
P.S. Matt,
I want to use the same type of form for my site sign up and also for someone to send me an actually enquiry, rather than a general message. How would you go about implementing that? Would it work if I wrote three separate sets of forms and handling scripts?
I’ve already had to rename the function from init() to initform() to avoid a conflict with your javascript tabs script, so I suppose it should be possible to triplicate everything? Or can you suggest a cleverer way for me to investigate? I was thinking along the lines of having a second set of js tabs which pop up like this contact form, but with a different form on each tab.
Ideally I’d like the enquiry form to allow someone to attach a file too, but I’ll cross that bridge when I come to it.
[Edited by Stanley on 24-Aug-12 23:17]
Hi Matt,
I having a problem with your form since I move my web server to my own iMac. My server before was yahoo server but now i decide to host my own site using OS X Server and since i move all my file the form giving me always some of the no sending messages because it doesn’t send the emails????
what could creating me this situation???
Best Regards
mbarcala
@mbarcala: Could be lots of things. You’ll need to look at each step in the mail sending process to see where the problem is.
– Is the PHP script set up correctly?
– Is the PHP engine correctly configured to send mail on your server?
– Is the Mac OS X MTA (postfix) correctly configured and running?
– Does your ISP allow outgoing mail to be sent on port 25? (Many ISPs don’t)
Hello all,
hi Matt.
For a friend of mine I’m workin on this form which really rocks (thanks so much) but I’ld like to protect it from being spam with those tiny pieces of code:
– in the form:
<input type=”text” name=”bot” id=”spambot” />
– in the CSS:
input#spambot{visibility: hidden; display: none;}
– in processform.php:
if(!empty($_POST) && empty($_POST[‘spambot’]))
but something is going wrong.
As I’m not a PHP expert I don’t know where in the processform.php file I have to put the php line.
If you can help it would be great.
Thanks in advance.
Thomas.
EDIT :
Found this which works great:
just add this:
– if(!strlen($_POST[‘spambot’])>0){
before:
if ( $senderName && $senderEmail && $message ) {
And no more spam.
[Edited by Detoussay on 12-Oct-12 08:42]
I have another question.
What will I have to change if i want to have the Form always visible?
I’s working but after having sent the email, the status message appears on a new page. The Ajax message doesn’t appear on the page itself.
Another question 🙂
Would it be possible to have the send button in javascript?
The idea behind is pretty simple:
as bots do not see javascript buttons (as far as I know) this button will be only seen by humans.
Another special button only seen byt bots will lead the to a kind of blackhole (see http://perishablepress.com/blackhole-bad-bots/ for the script).
And, tada, there we got a spamproof form (I hope)
@Detoussay: If you can post the URL of the form then we can take a look at the problem.
JavaScript-only submit buttons aren’t a good idea from an accessibility point of view. Besides, a spambot can still easily “fake” a form post by just sending a POST request to the server.
Oh, dear, it is no any defence in your script against sql injection and all site can be hacked in 1 sql line in body of request!
I am worring because I planed to use this script for
http://autoshop.kiev.ua
but now I am in doubt – is it safe or not?
it’s a fantastic little heavy-less ajax script, love it, but …
the formprocess … good grief that needs a major rewrite, every little boy can hack and inject you with their little left finger only… 😉
I love the whole setup but yes, … I won’t use it because of what I just addressed.
@Detoussay:
I don’t know PHP at all but, if you open something… ‘{‘ then you need to close it!
Hence, you should mention to add a ‘}’ after the one ‘if’ which you place your ‘if’ just in front of. … 😉
@matt:
you address Detoussay and tell him… a spammer still can …
Well that’s true, but using your form process php as it is, every spammer and all sick folk can do with you whatever they feel like.
Have you planned to come up with a secure form processing php at all?
Cheers
[Edited by hellolittlebro on 30-Nov-12 11:59]
Another thing… I added a message styling and also included the “msg” in the header, but nothing gets sent
Another thing… the current email validation isn’t really a validation… sdsd@q is not an email, is it? So I changed the reg
to
but it still gets validated the old way.
Yes – I cleared cookies and even flushed the cache, tried other browsers I haven’t opened it before and all the same …
Hm… nice as it is but try to add anything, nothing!
So after all, I guess it’s only for people who use it as is and don’t bother about security, hijacking, etc. at all. … perfect for the FaceBook crowd 😉
You can’t “validate” an email address until you send the message and it doesn’t “bounce”.
All validators of any kind do is check that the string entered has the ‘.’s and the ‘@’ in the right places, and does not contain any ‘illegal’ characters.
@chris
you just proved what kind of lousy programmer you are. What are you talking about, shall I send you my code I have on another form, a form which I actually USE!
?
Gee, another “professional” (spammer, hacker, phisher >>> ??? )! Can only be one fo these, to keep your doors open you lie at other! We know how you folks work. 😉 but not everyone is as dumb as you’d hope!
[Edited by hellolittlebro on 03-Dec-12 20:40]
In that case
“Another thing… the current email validation isn’t really a validation… sdsd@q is not an email, is it? ”
What is the question?
Because that is the one I answered.
@gurkin: The tutorial isn’t a lesson in how to prevent SQL injection (it doesn’t even use a database). It’s a tutorial showing how to use Ajax with jQuery and PHP to send an email message.
If you want to adapt the PHP script to insert data into an SQL database then you’ll need to take your own precautions against injection attacks. I recommend using PDO with bound parameters.
On your demo page the modal doesn’t close when clicking the escape key – doesn’t work on my page either 🙂
Any simple fix? Close outside solution would be nice too. ‘Slick’ form indeed otherwise.
Thx in-advance
——————————-
Found out how to make clicking outside the form close it.
// Close the Form when click outside
$(“#subscribeForm”).click(function(e) {
e.stopPropagation(); //stops click event from reaching document
});
$(document).click(function() {
$(“#subscribeForm”).hide(); //click came from somewhere else
$(‘.wrapper’).fadeTo( ‘slow’, 1 );
});
Got it from here: http://stackoverflow.com/questions/3314810/jquery-trigger-event-when-click-outside-the-element
[Edited by freeHat on 12-Jan-13 09:55]
@freeHat: Esc key works for me! What browser are you using?
Excellent tutorial, works straight out of the box. Am wondering why people are asking about SQL injections considering, uhmm, the form doesn’t write to a database!
Anyways my question to Matt, have no problems with the html, php, and css, but am a newbie to jquery 🙁 Trying to add an additional field to the form, Phone Number, and it blows the hide and show aspects 🙁
Have simply copied the Name <li> and renamed the variables to contactPhone, increased the form height, and added a check to the js that Phone has been entered.
Quite perplexing and no doubt something very simple that I am overlooking.
Thanks in the advance for any help.
@Jeff_R: Can you post the URL of your form page with the problem?
Hi Matt, This is certainly a slick contact form and its exactly what Im looking for, but I keep on having an issue with the form submit. Specifically, after I fill out the form and submit, I get the error message that there was a “problem sending.” However, the email actually goes through. I know some others had this same issue, but I can’t find the fix for it. The code I used is identical, from what I can tell, to the one you wrote. I could use a little help.
Thanks!
Update: I did a work around. Given the emails were going through, I just tweaked the javascript. Its not the most elegant, but it sure does feel damn good to not get the error message 🙂
if ( response == “success” ) {
// Form submitted successfully:
// 1. Display the success message
// 2. Clear the form fields
// 3. Fade the content back in
$(‘#successMessage’).fadeIn().delay(messageDelay).fadeOut();
$(‘#senderName’).val( “” );
$(‘#senderEmail’).val( “” );
$(‘#message’).val( “” );
$(‘#content’).delay(messageDelay+500).fadeTo( ‘slow’, 1 );
} else {
// Form submission failed: Display the failure message,
// then redisplay the form
$(‘#successMessage’).fadeIn().delay(messageDelay).fadeOut();
$(‘#senderName’).val( “” );
$(‘#senderEmail’).val( “” );
$(‘#message’).val( “” );
$(‘#content’).delay(messageDelay+500).fadeTo( ‘slow’, 1 );
}
[Edited by busseg on 16-Mar-13 12:05]
Hi Matt,
my hosting provider is yahoo and I having a problem receiving email using your form, is there any problem with you php file version and the 5.3.6 version?
i just don’t understand why is not working now?
i having change anything from your code since i don’t know much about php, but since yahoo change to 5.3.6 this problem start happening to me and i can’t go back to the php version before!
any help i will appreciate please?
Hello!
Can you point me how I can add to form and email body additional field Phone?
Thank yo!
@mbarcala: The PHP code looks OK (although of course you should uncomment the EMAIL_SUBJECT define at the top). What isn’t working exactly?
@one: See:
http://www.elated.com/forums/topic/5171/#post21905
http://www.elated.com/forums/topic/5171/#post21909
Hi Matt,
I have 2 issues for you with your form now
1- my subject field, display all word together when i receive the email?
and 2
with your header i can’t send any email with this form, alway give me an error message “There was a problem sending your message. Please try again.”
with my i can send the email BUT i can’t replay to the senderEmail, it just replay to my self.
what could be my problem? i’m using yahoo server PHP 5.3.6
hope you can help to figure out this situation
Best regards
mbarcala
Sorry subject field here
Hi, this is nice to tutorial 🙂
1)
My question is how use different language character in form filing like croatian Å ÄČĆŽ, i’ve been allready tried your suggestion with preg_replace /u but can’t accomplish it properly.
Could you please write where to add /u into this variable:
$message = isset( $_POST[‘message’] ) ? preg_replace( “/(From:|To:|BCC:|CC:|Subject:|Content-Type:)/”, “”, $_POST[‘message’] ) : “”;
http://croaticainfo.com
[Edited by cro2013 on 01-Aug-13 09:21]
@cro2013:
Hi Matt,
Thanks for the nice form! One thing has me puzzled, though. If I submit the form without filling in the name I get the message:
! Please fill out this field
But this is not the incompleteMessage and, in fact, this message appears before
is even entered. A search through all of your files doesn’t even find “Please fill out this field”. So where is this message coming from? What is checking the form at this level, before submitForm() is entered?
Thanks
[Edited by toonbon on 06-Jan-14 11:53]
Hi,
I cant send the mail through contactform i got below error always
There was a problem sending your message. Please try again.
Click your browser’s Back button to return to the page
. I am not change any code in processform.php. except below one
<?php
// Define some constants
define(“RECIPIENT_NAME”,”krishna”);
define(“RECIPIENT_EMAIL”,”[email protected]”);
define(“EMAIL_SUBJECT”,”Visitor Message”);
// Read the form values
my webpage jetnet.in
pls some one help me to fix it
Thanks
@kirshnam
you are using the wrong version of jqery, this tutorial is using jqery 1.5.1 and in your website you are using jqery 1.6.3, thats why is not working.
I have same problem that kirshnam and I use the correct version of jquery.
I have this problem when I submit the form “No ‘Access-Control-Allow-Origin’ header is present on the requested resource. Origin ‘null’ is therefore not allowed access.”
Thanks
“Origin ‘null’ is therefore not allowed access.”
Make sure it has a value then.
Hi Matt, and others.
This is an awesome form and I can’t wait to use it, however I’m having 1 issue.
I’ll start by saying all the functions are working to send and receive the emails.
My issue is the Java Script isnt firing.
I’m using a wordpress theme.
I’m placing the script in the header.
and the <FORM> in the <BODY> . The forum shows up at the end of the body, and when the button is clicked it just instant scrolls down to the #contactForm Class.
Is there an issue with this script and wordpress?
I want to add a field to the email submit.
I’m able to create the field in the HTML markup but I can’t get the field to be included in the email from the PHP
I tried replicating the $message – and changing all the variables to $phone but I wasn’t able to accomplish this.
Any help would be great! Thanks!
Fistly, fantastic script, I’ve had fun customizing it, very smooth and brilliant.
I’d like to make the email & name optional, is there a way to do that? I tried removing the “required” aspect, and I also tried editing the php file, removing the name and email from this line:
if ( $senderName && $senderEmail && $message )
But not luck. Any suggestions?
This works great if you are having the contact form send to an email on the same server. I’ve tried this on multiple servers trying to get it to email outside the server and it doesn’t work. Am I missing something or does this need additional code added to use sendmail or something similar?
[Edited by vegas on 13-May-14 12:32]
You need to configure sendmail to route mail via an external SMTP server, known as a ‘smarthost’.
http://www.howtoforge.com/configuring-sendmail-to-act-as-a-smarthost-and-to-rewrite-from-address
hi matt,
This is a very nice Contact Form. I have everything working and giving me the correct responses on my web page. The only thing I am having a problem with is. I am not receiving the email. I tried sending it to my yahoo account and then changed it to my gmail account but it is not getting to either one nor does it show up in my spam. Do you know or have a fix for this? thanks
“Do you know or have a fix for this?”
No and no, will you be offering more clues so we can assist?
Such as “Where are you sending mail from”?
@butterman, did you read the reply to my post about that exact problem from chrishirst? The form is not configured to use sendmail to deliver mail outside the server. Follow the directions in the link he gave in my post above yours or you can can create an email account on the server you have the script on and have that forward to your yahoo or gmail.
” The form is not configured to use sendmail to deliver mail outside the server.”
How do you know that??
“butterman” hasn’t actually said where the script is running, it may be the same problem as you have, but then it could be one of a dozen other things that create issues with Gmail and/or Yahoo! rejecting email messages.
You’re such a condescending cock sucker you know that chrishirst! You are the one that said in order to send outside the server the script is on to configure sendmail to use an external smtp.
“You need to configure sendmail to route mail via an external SMTP server, known as a ‘smarthost’.”
Why would your brilliant bitch ass say that if the script was really worth a fuck and had the ability already built into it? It does now that I’ve torn it all apart and rewritten it to save to MySQL and email to any email configured into it.
You’re a douchebag dude, people are asking for help not a punk with a smart ass answer like you have every time!
That was as an answer to a completely different question, one were the real problem was known.
Now, maybe you should go and look up the meaning of tutorial. The script isn’t intended as a fully featured, “one script fits all” solution, it is for anyone learning to code to LEARN FROM and extend as necessary.
Just because the message does not arrive at Yahoo and Gmail account does not mean that it is not leaving the machine the script is.
Anyway when you have grown up enough to know that trying to insult someone you don’t know is rather infantile, and a little pathetic, you might even know that ASSUMING anything is not the way to provide useful answers.
I am sending mail through godaddy and down to my gmail account. It works great when someone puts their hotmail account in and now their gmail account, It’s just not coming though when they use their yahoo email account. I am not sure about outlook or any other email accounts at this time. I think the problem has to be with this SMTP stuff you are talking about so I will go school myself on that and see if I can find a fix. I think this is the best looking contact form out there so I will get it to work. When I find the fix I will let you know. In the mean time if something comes to mind. Please let me know. Thank you for your time.
Chris….I finely understand what you mean about route mail via an external SMTP server. I was actually trying to route the emails straight to my gmail account from the processform.php file. Everything was showing up but the yahoo emails for some reason where not. Then I created a new email name in my godaddy account and put that email name in the processform.php file. I put a forward to my gmail from my new godaddy email and everything works. I am going to assume thats what you mean by route mail via an external SMTP server, (ie… use the email name on the server not you gmail name) Thank you for the help……
Good, and thanks for returning and posting the steps you took to solve it, that will possibly assist future viewers of this thread.
And to vegas as it transpires your guess was correct but you STILL need to learn how to address people without using profanities and infantile insults.
I followed along with this tutorial and I must say, wow. Very insightful. Thank you so much.
I am running into one problem while trying to add a little extra functionality and I cannot seem to wrap my mind around it. If I leave everything as is, the code works great and it sends the email just fine. But what I am trying to add into the PHP page is the functionality to where, before the email is sent, it stores the variables into a MYSQL database. However, the moment I add the code below, I receive the message: “There was a problem sending your message. Please try again.” If I remove the INSERT statement from the PHP page, everything goes back to running smoothly and sending the email. Here is the current code I am using to try and insert the data into the database:
If anyone can help me with this issue, that would be incredible. Thank you so much.
I wanted to post again because, after much head-banging against a wall (LOL), I finally realized the errors of my way. The problem was that I was trying to insert into a database that I hadn’t connected to yet. So al I had to do was actually connect to the database (what a novel idea) and all was well.
Thanks again Matt for a wonderful tutorial.
After getting everything to work properly, I actually do have 1 question about the difference between the example on this site and the one I implemented on my site.
When I downloaded the source codes and applied the files to my server, and tried it out, it worked great both on my desktop and on my mobile phone. On my mobile phone, the modal window fits nicely into the size of my screen. However, when I view my version that I altered a bit on my mobile phone, the modal does not shrink down, and most of the important buttons and text fields fall off the screen and makes it impossible to use. Does anybody have an idea of what would make mine differently? Here is my CSS code that I used:
Any help on this issue would be greatly appreciated.
I’m implementing this form into my site as we speak because without doubt, it is unbelievably slick! BUT one problem I’ve been trying to sort out, can anyone figure out why theres a big blank space in the message text area and it’ll only display the placeholder image after deleting some whitespace? thanks 🙂
my site is at http://joewinfield.net/portfolio
A textarea element will display everything that is between the start and end tags, so if your source code has a line break in the tag structure, … So will the rendered document.
Chrishirst you’re right! i accidentally left in whitespace when cleaning up how my code was laid out. the smallest things always catch you, thanks 🙂
I know you’re showing how to make a contact form appear so it’s non-obstrusive on a page, but if I just wanted to load the contact form so it appears within a page(without onclick) and still functional, how can it be done?
Make it visible by default rather than requiring the mouse click.
Hi Matt, still using this great script but I’ve recently been hit (big time) by spambots.
Rather than mess around with captchas I added a line of code to capture the spammer/bot’s IP address and include this in the email I receive from the form, then I add that IP address to a blacklist I use to filter out unwelcome guests and redirect them to somewhere else.
In case this helps anyone else I thought I’d share:
1) Add the following line to processForm.php, at the end of the section near the top which says “// Read the form values”
2) Add the following script to the top of the page containing your contact form:
There are a couple of examples there to show how I do it – I add the offending IP address AND I also use a wildcard “*” to block any similar IP addresses.
You can redirect the spammer to another address – on a company site I take care of I actually redirect them to our company Facebook page instead.
I entered all the code but when i tried it, it just gave me the “Please complete all the fields in the form before sending.” message and all the fields were filled in. desperate for help
Without seeing YOUR code, were not going to be able to provide any.
Helo house please am having “There was a problem sending your message. Please try again.” error and all the fields are complete. please help its urgent
read the reply above yours and below the one asking EXACTLY the same question.
I have two questions. First is there a fix the yahoo mailing problem. I have my mail which is hosted on godaddy.com forward my mail to my gmail. If the sender post their email account with a domain of @gmail and @hotmail it gets forwarded to my mail wit no problems, but if the sender post with @yahoo domain I don’t receive anything.
Second question: i’m trying to add the css on to an external stylesheet like I do for all css, but for some reason the css only works when it is embedded in the main html page. Am I missing something?
Please help me out
“Am I missing something?”
Probably.
“but if the sender post with @yahoo domain I don’t receive anything.”
You probably need to ask Godaddy support about that.
Is there anyway to send the emails directly to my email rather than fowarding it i.e. to an email outside the server which it is hosted in?
And I figured out the external stylesheet problem, had to back out of the directory with ../
“Is there anyway to send the emails directly to my email rather than fowarding it i.e. to an email outside the server which it is hosted in? ”
Again that has to be a no idea.
Does your server have a MTA installed on it?
Thanks
Greet you the author!
This is a great lesson, and the script feedback form – in my opinion, is the best among others.
But I have trouble you with my problem.
It related with use of the Cyrillic text.
I have read and reviewed all the posts about the problem with the UTF-8 here. Made all the changes and additions that you suggested.
Namely:
and
Sender’s name – it’s OK, however, text of the message looks unpresentable (for example – “аŸб€аОаВаЕб€аКаД, although it should be “Проверка”), if in the form it was writing in Cyrillic.
In addition, viewing the body of the message, I saw that the script does not assign message encoding. In the source code of the letter was written: “…X-Amavis-Alert: BAD HEADER SECTION, Non-encoded 8-bit data…”
Please help!
Hi,
I am trying to use your script within my own form(its always shown).
I copied your js and php code, named my input fields and button as you did. I also put the code in a seperate file and included it at the top of the page after the js CDN ofc.
But the ajax part is not working, i just get the php messages (the mail get send btw).
Any idea why this is happening?
Absolutely no idea.
You have probably missed something out.
Hi very nice contact form works perfectly, I would like to know how i can launch directly the contact form (without seeing the index page ), from for example my menu bar without using the standard link.
Thx in advance!
Put the form code in the menu bar code.
Really nice contact form, any well written tutorial. But I have kind of an odd question. I’ve been tinkering around with the CSS, and debating on whether I want to keep the shadows in the input fields.
This the part I’ve been tinkering with:
When I take out the shadows, and test the form offline by filling in random info, it does what I expect it do, again I haven’t put this on any server. However when I erase the fields after, all of a sudden there’s this red border around the input fields, similar to what one would see after they visited a hyperlink.
I know how to control the visited hyperlink color with CSS, but is there any way to do this with the form fields? By the way this only seems to happen with Firefox, and not Chrome.
“However when I erase the fields after, ”
erase them after, … … what?
AND
“erase them” means what exactly?
“there’s this red border around the input fields, similar to what one would see after they visited a hyperlink.”
I don’t see a “red border” visited links on sites that I visit, is this something specific to the code that you are using?
Let me see if I can explain this a different way. The part of the css code that I was playing with:
produces the inner shadows in the input fields. So I was curious to see what those fields (name, e-mail, and message) would look like with their inner shadows turned off.
So I commented out that bit of code above so the shadows would be turned off. After that I try out the form, typing random info into the fields. Then say for instance, the info I typed was not what I intended to type in, for whatever reason. So I click in the field select whatever text I typed in there, then delete that text.
If I then after that I click anywhere else on the form, but outside of that particular field I just visited, a red border appears surrounding that field. I was comparing this to when a hyperlink that’s already been clicked on turns a different color, then what it originally was.
Hopefully this explanation makes more sense. And again for whatever reason this only seem to happen in Firefox, and not Chrome.
[Edited by Jinjoe on 20-Aug-15 16:39]
That’s what Firefox does with a “required” input that is empty and the document is declared as HTML 5
[Edited by chrishirst on 21-Aug-15 10:58]
Okay that makes sense. Thanks a lot.
Is it possible to send the email to multiple address’s?
Send them are a correctly formatted recipients header to the mail server and yes you can.
Hmmm. Guess I had that coming. Allow me to re-phrase. How do you send to more than one recipient?
In the processForm.php file line #5 . . .
define( “RECIPIENT_EMAIL”, “[email protected]” );
I tried many combinations of separating by commas, semi-colons, adjusting the quotes and even adding a “RECIPIENT_EMAIL2” with no success.
As I said, format it as a valid multiple recipients To: header.
“Friendly Name <[email protected]>; Friendly Name <[email protected]>”
Take note that ALL the recipients will see all the mail addresses.
NOTE: The separator is a semicolon though some servers will allow the use of a comma to separate additional addresses.
[Edited by chrishirst on 09-Sep-15 10:49]
Or you could add a CC and/or BCC header to the script.
And if you leave off the FriendlyName the email address will be displayed in the “To” field in mail clients.
[Edited by chrishirst on 09-Sep-15 10:52]
Chris thanks for your help but it doesn’t look like I’m going to pull this off. With your pseudo you provided I’ve tried a hundred combinations and either get the message that their was a problem or the second email is never received. Below is one of the attempts within the “processForm.php” file.
The semicolons need to be IN the string, immediately following the closing bracket.
Like this ?
That was one of the earlier attempts. I just retried still get the message “their was a problem sending your email”
I’m only trying for two email addresses so the last semi-colon is the end of line.
Same error msg with this method . . .
The semicolons should separate EACH EMAIL ITEM.
This is how it should be formatted
$recipient = “Friendly Name <[email protected]>; Other Friendly Name <[email protected]>”
$recipient should be sent to the server as a single string
If you do not know how to concatenate several variables into a single string, do some basic research before trying anything complex.
AND
IF you do not know how to debug problems in code, do some basic research on that as well.
HINT: it involves printing the values to the browser so you can check it is what you expect.
The Elated tutorials are here so YOU can learn how to write and debug code for yourself. Sure if you want me to rework the entire code to what you need I can send you a proforma invoice if you wish.
But I will not give exact code answers, as that would defeat the entire point of a tutorial. I already know how to write the code so do not need the practice. I will only provide pointers to where the problem may be, then I expect YOU to research what I have told you so YOU learn how to solve them for your self, now and in the future.
It’s the “Teach someone how to fish” vs “Give someone a fish” principle
@jspartan Check out the docs for the mail() function:
http://php.net/manual/en/function.mail.php
Looks like recipients in the $to parameter need to be separated by commas (not semicolons).
@matt it worked perfectly. I appreciate; the absence of wrong psuedo code, proforma invoice and lecture. Just the correct information. Thank you sir!
At the risk of giving away a fish (lol) this is what worked for me . . .
Take care and thanks again!
“I appreciate; the absence of wrong psuedo code, proforma invoice and lecture. Just the correct information”
So you didn’t even bother checking the source of all PHP information, before you went down the “lazy coder” route.