The Ruby programming language is becoming increasingly popular, thanks to its clean syntax, its object-oriented features, and its range of high-quality libraries and frameworks.
In this tutorial, you get a gentle introduction to programming in Ruby. You learn:
- How Ruby works, and what it’s used for
- How to install Ruby on your Mac, Windows PC, or Linux PC
- How to create — and run — your first Ruby script
- Some of Ruby’s object-oriented programming features
- How to create and use classes and objects, and
- How to use Interactive Ruby to explore the Ruby language.
At the end of the tutorial, you also explore some resources for taking your Ruby skills further.
Let’s start with a brief introduction to Ruby, and take a look at the language’s features and benefits.
Introducing Ruby
Ruby is a powerful, yet easy-to-learn object-oriented programming language with a nice clean syntax. It was invented in Japan in the mid-nineties, but has really taken off in recent years, largely thanks to the Ruby on Rails framework, which lets you easily write powerful dynamic websites and web apps in Ruby.
As well as being popular with web developers, Ruby is also used for many other purposes, including system administration tasks and writing GUI-based desktop apps for Windows, Mac OS and Linux.
Ruby is, by default, an interpreted language, much like PHP, Perl and Python. This means that you need to install a Ruby interpreter to process and run your Ruby programs and scripts.
There are also Ruby compilers available that can turn your Ruby code into stand-alone apps.
In the next section, you begin your Ruby journey by installing a Ruby interpreter on your Mac or PC.
Installing Ruby
In order to start programming in Ruby, you first need to install the Ruby interpreter on your computer. The Ruby interpreter is the program that takes your Ruby script files and runs them.
The following sections briefly cover how to install Ruby on a Mac, on a Windows PC, and on a Linux PC.
Installing on a Mac
Have a Mac? Lucky you! Ruby comes pre-installed in Mac OS X. Proceed to the next section.
Installing on a Windows PC
RubyInstaller is an app that installs a complete, self-contained Ruby environment on your PC. It’s the quickest way to get Ruby up and running on a Windows machine.
To install Ruby on a Windows 7 PC using RubyInstaller, follow these steps:
- Download RubyInstaller
Visit the RubyInstaller downloads page and download the latest installer; at the time of writing, this isrubyinstaller-2.0.0-p0195.exe
. - Run the installer
Double-click the.exe
file to run the installer. In the Security Warning dialog that appears, click Run. In the Select Setup Language dialog that appears, choose your language and click OK. Follow the installer wizard. Make sure you select the “Add Ruby executables to your PATH” and “Associate .rb and .rbw files with this Ruby installation” options, since it will make it easier for you to run your Ruby scripts.
You’ve now installed Ruby on your PC. Proceed to the next section.
Installing on a Linux PC
Installing Ruby on Linux partly depends on what distro you use. For Debian-based systems (including Ubuntu), you can just run the following command, replacing “1.9.1” with the currently-available Ruby version:
sudo apt-get install ruby1.9.1
If you prefer a GUI approach, you can instead use a package manager such as Ubuntu Software Center to install the Ruby package.
If you start getting seriously into Ruby development on Linux, you might prefer to install RVM (Ruby Version Manager) and use it to install and update Ruby, rather than using the distro’s package manager. RVM is generally better at keeping your Ruby system and libraries up to date.
Read more about various ways to install Ruby, and their pros and cons.
Writing a simple Ruby script
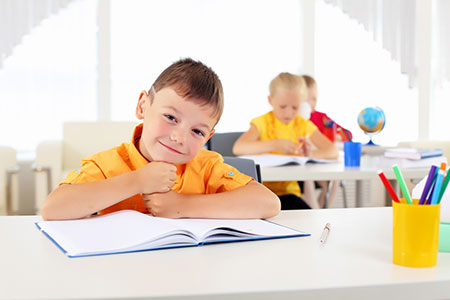
Now that you have the Ruby interpreter installed on your computer, let’s try it out by writing a very simple Ruby script and running it.
Using your favourite text editor, create a new text file called greeting.rb
. Type the following text into the file:
puts "Hello, what's your name?" name = gets.chomp puts "Hi there, " + name + "!"
Now save the file.
Let’s work through this script line by line, and see what it does:
- Display a prompt.
The first line of code displays the message “Hello, what’s your name?” on the screen. To do this, it calls a method namedputs
, passing the string argument"Hello, what's your name?"
to the method. If you’re not familiar with object-oriented programming, a method is a piece of code attached to a class, object, or module. When you call a method (by typing its name in your script), Ruby runs the code inside the method. Theputs
, or “put string”, method happens to be attached to a built-in Ruby module calledKernel
, and its job is to output its string argument to the terminal window.You explore classes, objects and methods in more detail later in this tutorial.
- Get the user’s name.
Now that we’ve prompted the user to type their name, we need to read the text that they enter on the keyboard. We do this by calling thegets
method in line 2. This method is the opposite ofputs
; whereasputs
outputs a string of text,gets
reads in a string of text typed in by the user, and returns it. We then take this string value, which is actually an object (more on that in a moment), and call the object’schomp
method; this simple method merely removes the newline from the end of the string (created when you press Return on your keyboard to enter the string). We then store the resulting string in a new variable calledname
. - Display a greeting.
Finally, line 3 displays a greeting message to the user. The message consists of the string"Hi there, "
, followed by the user’s name (stored in thename
variable), followed by an exclamation mark (!
). Again, we use theputs
method to display the greeting. We also use the concatenation operator,+
, to join the three strings together to produce the greeting string.
Running the script
Try this script out! Open your terminal window and use the cd
command to change to the folder holding your greeting.rb
file. Type the following:
ruby greeting.rb
Then press Return. You should see the following message:
Hello, what's your name?
Type your name, then press the Return (Enter) key on your keyboard. You’ll see a message similar to the following:
Hi there, Matt!
Congratulations — you’ve just created and run your first Ruby script!
In Ruby, almost everything is an object
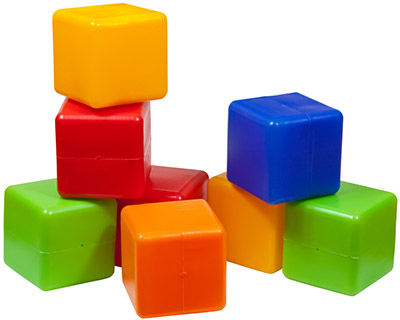
Ruby is, at heart, an object-oriented language, so to write Ruby code effectively you need to understand OOP (object-oriented programming) concepts such as classes, objects, properties and methods. If you’re not familiar with these concepts, have a quick read through Object-Oriented PHP for Absolute Beginners. It includes PHP code, but the OOP concepts also apply to Ruby.
While many languages, such as PHP, have object-oriented features, with Ruby the concept of object-oriented programming goes much deeper. In Ruby, almost everything in the language is an object, with methods that you can run, and instance variables (sometimes known as properties) that you can access via getter and setter methods. This includes:
- Objects that you create from your own classes
- Objects created from built-in Ruby classes and Ruby modules
- Basic data types, such as numbers, strings, arrays, hashes, and even classes themselves
Even literal values, such as the number 3
, are objects! This means you can, quite legitimately, call methods on the number 3
, like this:
puts 3.class # Display the class of 3 puts 3.even? # Display true if 3 is even, false otherwise puts 3.fdiv(2) # Divide 3 by 2 (floating-point division)
As in many other OOP languages, the dot (.
) operator calls methods. object.method
means: “Call method
on object
“.
The hash (#
) symbol signifies a comment. Everything from the hash to the end of the line is ignored by the Ruby interpreter.
If you run this Ruby script, you get the following output:
Fixnum false 1.5
Let’s look at how the script works:
- Line 1 calls the
class
method of the3
object. This method returns the class of the object as a string, which is then displayed withputs
. You can see that3
‘s class isFixnum
, a built-in class that stores and handles integer numbers. - Line 2 calls the
even?
method of the3
object. (Yes, method names in Ruby can include punctuation such as?
and!
.)even?
returns a Boolean value:true
if the number is even;false
if it’s not. The code then displays this Boolean value (false
in this case) usingputs
.Even
false
is an object in Ruby! It belongs to a class namedFalseClass
. - Line 3 calls the
fdiv
method of the3
object.fdiv
takes a floating-point number as an argument —2
in this case — and divides the object’s value (3
) by the argument to produce the result,1.5
, whichputs
then displays. (1.5
is an object of theFloat
class.)
As you can see, the fact that almost everything in Ruby is an object makes the language both powerful and intuitive. Being able to call methods on almost anything gives you a lot of flexibility, and helps you write succinct, readable code.
Creating a Member
class

Now that you understand the basic concepts of classes and objects in Ruby, have a go at creating a class yourself. Save the following file as member.rb
:
class Member def initialize(username) @username = username @loggedIn = false end def username @username end def login @loggedIn = true end def logout @loggedIn = false end def loggedIn? @loggedIn end end
This very simple script creates a class called Member
that you might use to handle members who login to your site or app. Let’s explore each part of this script:
- The
Member
class definition
The first and last lines of code,class Member
andend
, tell Ruby that we want to create a new class calledMember
. All the code between these two lines defines how theMember
class behaves. - The constructor
Lines 3 to 6 form the class’s constructor. A constructor is a method that is called automatically whenever a new object of the class is created. In Ruby, the constructor method is always calledinitialize
.To create a method, you use this syntax:
def methodName # method code here end
Or, if you want your method to take arguments:
def methodName(argumentList) # method code here end
Our constructor method takes a single argument:
username
. It then defines two variables:@username
, which it sets to the value of theusername
argument, and@loggedIn
, which it sets tofalse
.These two variables are instance variables, signified by the fact that they begin with an at symbol (
@
). An instance variable (sometimes called a property) is a variable that is associated with each object created from the class. So eachMember
object that we create has its own@username
and@loggedIn
instance variables.@username
stores the member’s username;@loggedIn
tracks whether the member is logged in or not.Our constructor, then, lets us create new, logged-out
Member
objects with each member’s username set to an initial value. - The
username
accessor method
Lines 8-10 create a method calledusername
. This method takes no arguments. Its single line of code merely references the@username
instance variable. In Ruby, the last expression evaluated within a method is sent back as the return value to the code that called the method. The line:@username
could be written:
return @username
The
return
is not needed here, however, and it’s considered good Ruby programming practice to leave it out.So the
username
method simply returns the value of the@username
instance variable to the code that called the method. This is known as an accessor method (more specifically, a reader method) because its sole purpose is to give outside code access to the@username
instance variable.In OOP it’s considered bad practice to allow outside code to read and alter instance variables directly; you should (nearly) always add accessor methods to the class, and use those instead.
- The
login
andlogout
methods
Thelogin
method on lines 12-14, like theusername
method, takes no arguments. It simply sets the value of the@loggedIn
instance variable totrue
, thereby marking the member as “logged in”. Similarly, thelogout
method on lines 16-18 sets@loggedIn
tofalse
. - The
loggedIn?
method
The last method defined in theMember
class isloggedIn?
, created on lines 20-22. It returnstrue
if the member is logged in, orfalse
if they’re logged out.
As mentioned earlier, method names can contain punctuation symbols, and it is a common Ruby programming convention to use method names ending in a question mark (?
) for methods that return a Boolean value.Since
loggedin?
simply returns the value of the@loggedIn
instance variable, it is in effect an accessor method for@loggedIn
.
Creating and using a Member
object
Now that we’ve built our Member
class, let’s try using it to create a Member
object. Add the following lines of code to the end of your member.rb
file:
member = Member.new("Fred") puts member.username + " is logged " + ( if member.loggedIn? then "in" else "out" end ) member.login puts member.username + " is logged " + ( if member.loggedIn? then "in" else "out" end ) member.logout puts member.username + " is logged " + ( if member.loggedIn? then "in" else "out" end )
Run your script in your terminal window by typing the following:
ruby member.rb
You’ll see the following output appear:
Fred is logged out Fred is logged in Fred is logged out
Let’s take a look at what our script does:
- Create a new
Member
object
The first line of new code – line 26 — creates a newMember
object and stores it in amember
variable. To do this, it calls thenew
method of theMember
class. This method is built into all Ruby classes; it creates a new object of the class, then calls the class’sinitialize
method (if present). We pass a string ("Fred"
) to Member’sinitialize
method, which then sets its@username
instance variable to"Fred"
and its@loggedIn
instance variable tofalse
. - Display the member’s status
Line 27 calls our newMember
object’susername
accessor method, which returns the value of the@username
instance variable ("Fred"
). It also calls theloggedin?
method to determine if the@loggedIn
instance variable istrue
; if so, it displays the message"Fred is logged in"
. In this case,loggedIn?
returnsfalse
, so it displays"Fred is logged out"
.The
if..then..else..end
construct operates much like it does in other languages. If the expression istrue
, the code block betweenthen
andelse
is run. Otherwise, the code block betweenelse
andend
is run.Notice that, in Ruby, you can simply write an expression (
"in"
or"out"
) in a code block, whereas in most languages you would need to write a statement (such asx = "in"
orprint "out"
). Ruby evaluates the wholeif..then..else..end
construct as an expression; in this case it evaluates to"out"
, which we then append to the"Fred is logged "
string using the+
operator. - Log the member in
On line 28 the code logs the member in by calling the object’slogin
method, which sets its@loggedIn
instance variable totrue
. Line 29 then displays the member’s status again, as described in Step 2 above. This time the message displayed is:"Fred is logged in"
. - Log the member out
Line 30 logs the member out again by calling the object’slogout
method, setting its@loggedIn
instance variable tofalse
. Finally, line 31 displays the member’s status, as described in Step 2. Now the message displayed is:"Fred is logged out"
.
Interactive Ruby: Playing with Ruby code
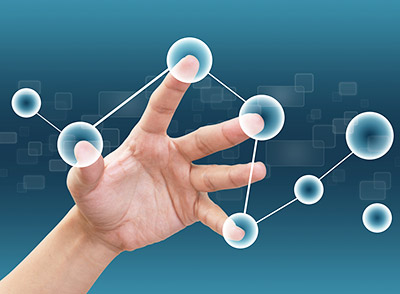
Now that you have a basic understanding of some key Ruby features, a good way to explore the language further is to play around with Interactive Ruby. This is a useful program that comes with your Ruby installation; it lets you type in lines of Ruby code and immediately see the results.
To run Interactive Ruby, open a terminal window and type:
irb
Then press Return. You’ll see a prompt (>>
) appear. Type in a line of Ruby code, and press Return to see what your code evaluates to. Here are some examples (the typed-in code is in bold):
>> puts 3.class Fixnum => nil >> x = 3 => 3 >> puts x 3 => nil >> x => 3 >> 3.fdiv(2) => 1.5
Each time you enter an expression, Interactive Ruby displays the expression’s value on a new line, preceded by =>
.
Notice that almost every Ruby statement is an expression that evaluates to some sort of value. Even the puts
method call evaluates to a value (nil
), in addition to displaying its output.
To exit Interactive Ruby, type exit or quit and press Return.
Summary
In this beginner tutorial, you explored the basics of the Ruby programming language. You looked at:
- What Ruby does, and how it came about
- How to install Ruby on your computer
- How to create and run a simple Ruby script
- Ruby’s approach to object-oriented programming: Almost everything in Ruby is an object
- How to build a simple
Member
class - How to create and use objects from your
Member
class, and - Using Interactive Ruby to learn more about the language.
Further Reading
If you’d like to explore Ruby further, take a look at the following resources:
- The official Ruby site, which has tons of useful online manuals and tutorials.
- Try Ruby, a web-based version of Interactive Ruby that includes a built-in tutorial.
- Ruby-Doc.org, a comprehensive documentation site for Ruby APIs and libraries.
- RubyGems, which hosts thousands of packaged, easy-to-install Ruby libraries and applications, known as gems.
- Ruby on Rails — also known simply as “Rails” — is a popular open-source web development framework that lets you build powerful websites easily using Ruby.
Have fun, and happy Ruby coding!
[Image credits:
Leave a Reply