Templates are a great way to separate your website’s code from its design. There are many great web template systems available for different languages and platforms, including Smarty for PHP and the Django template language for Python.
In this tutorial you’ll explore Mustache, a relatively new and very simple template system that you can easily use to create HTML templates. You’ll look at various topics, including:
- The advantages of using Mustache
- How to install and use the Mustache processor
- How Mustache tags, variables, and data objects work
- Using sections to create conditions and loops
- Including one Mustache template inside another
- …and lots more!
Along the way, you’ll see plenty of code examples that show you how Mustache works. At the end of the tutorial, there’s a complete example that uses Mustache, along with jQuery, to create a simple Ajax-based product list page.
What is Mustache?
Mustache is a simple template system that you can use when building websites and web apps. By using a template system, you can keep your back-end code separate from the markup that displays the front end to the user. This clean separation gives you many advantages. For example, it makes it easy for a designer to work on a website’s visual design without the risk of messing up the site’s code. It also makes it easy for you to change the design at a later point without impacting the back-end code.
One of Mustache’s big plus points is that is logic-less, which means it keeps your templates very neat and tidy. There are no messy if ... then
or looping constructs embedded within a Mustache template; it’s all just markup and simple Mustache tags. All the logic is hidden away inside your data objects (and the code that creates or fetches them).
Another advantage of Mustache is that you’re not tied to any particular language. Mustache processors are available in many languages, including JavaScript, PHP, Perl, Ruby, Python, Java and lots more.
You can use Mustache for practically any kind of template, including config files and even source code. However, this article concentrates on using Mustache to build HTML templates, which is a very popular use of the system.
Mustache is used by a lot of popular websites and web apps, including Twitter and OMGPOP (maker of the ever-popular Draw Something game). Here’s a list of sites that use Mustache.js, the JavaScript version of Mustache.
A basic Mustache example
What does a Mustache template look like? Here’s a simple example:
<h4>Product Info: {{name}}</h4> <ul> <li>Product: {{name}}</li> <li>Colour: {{colour}}</li> <li>Price: ${{price}}</li> </ul>
As you can see, this template is essentially HTML mixed with a few special Mustache tags. A Mustache tag begins with two opening braces ({{
) and ends with two closing braces (}}
).
As you might have guessed, the {{
and }}
delimiters are where Mustache gets its name from!
Inside each tag is the name of a variable (in this case, name
, colour
, and price
). When Mustache processes the template, it replaces each variable name with an actual value.
But where does Mustache get the values for the variables in the template? The answer is that, when you pass the template to the Mustache processor, you also pass an object or hash containing the variable names and their associated values:
{ "name": "SuperWidget", "colour": "Green", "price": "19.99" }
The exact format of the object or hash depends on the language you’re using. The above example is a JavaScript (or JSON) object. But essentially you pass Mustache a list of name/value pairs.
When you pass the template and object to the Mustache processor, it combines the two and returns the final HTML, with the variable names replaced by their values:
<h4>Product Info: SuperWidget</h4> <ul> <li>Product: SuperWidget</li> <li>Colour: Green</li> <li>Price: $19.99</li> </ul>
Installing Mustache
The Mustache processor is available in a wide range of languages. As a general rule, you install Mustache like this:
- Visit the GitHub page for your chosen language (for example, JavaScript or PHP).
- Click the ZIP button to download the repository Zip file.
- Unzip the downloaded file, and move the resulting folder to your website.
- Include the relevant library file (such as
mustache.js
orMustache.php
) in your web pages or server-side scripts.
There are some subtle differences between the Mustache implementations for different languages. Make sure you read the documentation specific to the Mustache processor you’re using.
Running the Mustache processor
The syntax for calling the Mustache processor depends on the language you are using. Essentially though, you call the Mustache
class’s or object’s render()
method, passing in the template string followed by the data object. The processor then combines the template with the data object to produce the final markup string, which it returns.
Here are some examples:
JavaScript:
output = Mustache.render( template, data );
PHP:
$m = new Mustache; $output = $m->render( $template, $data );
Perl:
use Template::Mustache; $output = Template::Mustache->render( $template, $data );
A Mustache demo page
Let’s get going with a simple demo page that shows Mustache in action. We’ll use mustache.js
— the JavaScript implementation of Mustache — so that we can run the demo straight in the browser, without needing to write any server-side code.
The JavaScript version of Mustache is very handy for Ajax-driven web apps. Your app can pull JSON data from the server using Ajax, then combine the data with a Mustache template to create the final markup for displaying in the page. You’ll learn how to do this in the complete example at the end of the tutorial.
Grab mustache.js
To start building the demo page, you first need to install the JavaScript Mustache processor:
- Create a folder somewhere on your computer
- Download and unzip the JavaScript Mustache processor
- Copy the resulting
mustache.js
file into your folder
The HTML page
Here’s the demo page — save it as demo.html
in the same folder as your mustache.js
file:
<!doctype html> <html> <head> <title>A Simple Mustache Demo</title> <meta charset="utf-8"> <link rel="stylesheet" href="style.css" /> <script type="text/javascript" src="http://code.jquery.com/jquery-1.7.1.min.js"></script> <script type="text/javascript" src="mustache.js"></script> <script type="text/javascript" src="mustache-demos.js"></script> </head> <body> <h1>A Simple Mustache Demo</h1> <div id="wrap"> <div class="template"> <h3>Mustache Template:</h3> <textarea id="template"> <h4>Product Info: {{name}}</h4> <ul> <li>Product: {{name}}</li> <li>Colour: {{colour}}</li> <li>Price: ${{price}}</li> </ul> </textarea> </div> <div class="data"> <h3>Data Object:</h3> <textarea id="data"> var data = { "name": "SuperWidget", "colour": "Green", "price": "19.99" } </textarea> </div> <div class="process"><button onclick="process()">Process Template</button></div> <div class="html"> <h3>HTML Result:</h3> <textarea id="html"> </textarea> </div> <div class="result"> <h3>How It Looks:</h3> <div id="result"> </div> </div> </div> </body> </html>
This page contains the following elements:
- A
style.css
include
This contains the CSS to display the demo page. We’ll create this file shortly. - Three JavaScript includes
We’ve included jQuery, themustache.js
library file, and amustache-demos.js
JavaScript file (which we’ll create in a moment). - A
textarea
with anid
of"template"
This contains the Mustache template that we want to use. Notice that the template is mainly HTML markup, with a few Mustache tags, indicated by the{{
and}}
delimiters. - A
textarea
with anid
of"data"
This contains JavaScript code that creates an object variable calleddata
, with three properties:"name"
, with a value of"SuperWidget"
;"colour"
, with a value of"Green"
; and"price"
, with a value of"19.99"
. - A
button
with anid
of"process"
When clicked, this button calls a JavaScript function,process()
, which runs the Mustache processor to produce the finished HTML. We’ll create this function in a moment. - A
textarea
with anid
of"html"
This will contain the finished HTML generated by the Mustache processor, displayed as source markup. - A
div
with anid
of"result"
This will contain the finished HTML generated by the Mustache processor and rendered by the browser, so that you can see how the ends result looks in the page.
The style sheet
Here’s the style sheet for the page — save it as style.css
in the same folder as demo.html
:
body { font-family: "Georgia", serif; line-height: 1.8em; color: #333; } #wrap { width: 57em; } textarea, .result div { font-size: .8em; width: 29em; height: 15em; margin: 1em 2em 2em 2em; padding: 1em; } textarea { font-family: Courier, fixed; } .template, .html, .data, .result { float: left; } .result div { border: 1px solid #333; } h3 { display block; margin: 2em 0 0 1.5em; } .process { display: block; clear: both; margin: 1em 0; width: 56em; text-align: center; } button { font-size: 1.5em; margin-top: 1em; }
This CSS aligns the various elements in the demo page — including the textarea
s, the #result
div
, and the #process
button — and styles them.
The JavaScript
Finally, here’s the JavaScript code for the demo page — save it as mustache-demos.js
in the same folder as demo.html
:
function process() { var template, data, html; template = $('#template').val(); eval( $('#data').val() ); html = Mustache.render( template, data ); $('#html').text( html ); $('#result').html( html ); }
This function, process()
, runs when the user presses the Process Template button in the page. The function:
- Extracts the template code stored in the
#template
textarea
, and stores it in the variabletemplate
. - Calls
eval()
on the contents of the#data
textarea
, which creates thedata
object. - Calls
Mustache.render()
, passing in the Mustache template stored intemplate
, as well as the data object stored indata
. This generates the finished markup, which the code then stores in thehtml
variable. - Inserts the markup stored in
html
into the#html
textarea
, as well as the#result
div
, so that the markup can be both viewed by the user and rendered by the browser.
Try it out!
To try out the demo, open demo.html
in your browser, or press the button below:
You’ll see four boxes in the page. The Mustache Template box and the Data Object box are filled with the template code and the JavaScript code to generate the data object. The HTML Result and How It Looks boxes are initially blank.
Now press the Process Template button. This runs the Mustache processor, passing in the template and the data object. The resulting markup then appears in the HTML Result box, and is rendered in the How It Looks box:

Try editing the contents of the Mustache Template and Data Object boxes, then pressing the Process Template button again to see the results. This is a great way to play with Mustache and see what it can do.
Delving deeper into variables
So far you’ve learned how to create a Mustache variable by placing the variable name inside a Mustache tag, then creating a property with the same name inside your data object.
Let’s look at some more features of Mustache variables. In this section you’ll explore expressions and functions; how to access object properties and methods within templates; how Mustache handles missing variables; and how Mustache HTML-escapes certain characters within variable values.
Expressions and functions
With most languages, you’re not limited to using literal values in your Mustache data objects. You can also use expressions and functions as values. This means your object can contain properties such as:
"price": parseFloat(netPrice+tax).toFixed(2)
…or:
"price": calcPrice
(where calcPrice()
is a function). Mustache then replaces the variable tag with the result of the expression, or the return value of the function, in the output.
One notable exception to this rule is JSON, which doesn’t allow expressions as property values.
Accessing object properties and methods
Your data object can also contain other objects — for example:
{ "name": "SuperWidget", "colour": "Green", "price": { "regular": "19.99", "discount": "14.99" } }
To access a property or method of an object from within your Mustache template, you can use the dot (.
) notation, like this:
<h4>Product Info: {{name}}</h4> <ul> <li>Product: {{name}}</li> <li>Colour: {{colour}}</li> <li>Regular Price: ${{price.regular}}</li> <li>Discount Price: ${{price.discount}}</li> </ul>
Combining the above object and template produces the following output:
<h4>Product Info: SuperWidget</h4> <ul> <li>Product: SuperWidget</li> <li>Colour: Green</li> <li>Regular Price: $19.99</li> <li>Discount Price: $14.99</li> </ul>
Missing variables
If you use a variable name in your template that doesn’t appear in the corresponding data object, Mustache simply replaces the tag with an empty string. For example, this Mustache template:
Hello, {{first}} {{last}}!
when combined with this data object:
{ "first": "John", }
produces this result:
Hello, John !
HTML escaping
Mustache automatically replaces certain HTML characters, such as <
and >
, with their equivalent HTML entities, such as <
and >
. If you don’t want Mustache to HTML-escape a value, put triple braces around the variable name instead of double braces, like this:
{{{name}}}
Alternatively, you can place an ampersand after the opening double brace, like this:
{{&name}}
A quick demo
Press the button below to see expressions, functions, accessing object properties, missing variables, and HTML escaping in action:
Working with sections
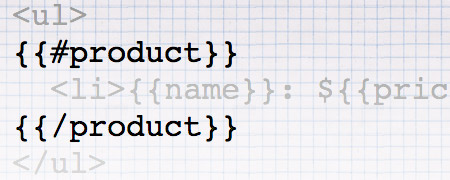
Sections let you add more power to your templates. Using sections, you can display a chunk of markup only if a certain condition is true (or false). You can also create repeating sections, which let you display lists and tables of data.
The basic syntax for a section looks like this:
{{#sectionName}} (more markup in here) {{/sectionName}}
Conditional sections
A conditional section is a block of markup that is displayed only if a certain condition is true. Here’s an example.
Say your template looks like this:
{{#inStock}} <li><a href="#">Buy Now!</a></li> {{/inStock}}
If your data object looks like this:
{ "inStock": true }
…then the output will look like this:
<li><a href="#">Buy Now!</a></li>
On the other hand, if your data object looks like this:
{ "inStock": false }
Or like this:
{ }
…then nothing will be output.
Inverted sections
Inverted sections are the opposite of conditional sections. With an inverted section, the section’s content is only output if the section’s variable is false.
You create an inverted section by replacing the "#"
character with a "^"
character. For example:
{{^inStock}} <li>Sorry, out of stock.</li> {{/inStock}}
The li
element in the above example is only output if the value of the inStock
variable is false
.
To try out conditional sections and inverted sections, press the button below:
Press the Process Template button. Since the value of inStock
is true
, the “Buy Now!” link is displayed. Now try changing inStock
to false
in the data
object:
"inStock": false
Now when you press the Process Template button, the “Sorry, out of stock” message is displayed instead.
Repeating sections
Repeating sections are handy when you want to display a list or table of related data in the page. You create a repeating section like this:
- Add a section ( for example,
{{#mySection}} ... {{/mySection}}
) to your Mustache template. - Inside the section, place the markup and any variable tags that you want to display for each item in the list.
- Add a list (or array) property to your data object. Give the property the same name as the section (for example,
mySection
). - Each item in the list should be an object containing the properties corresponding to the variable tags you added in Step 2.
The Mustache processor then loops through the objects in the list. For each object, it replaces the variable tags in the section with the properties of the object, and outputs the section’s markup.
Here’s an example. First, the Mustache template:
<ul> {{#product}} <li>{{name}}: ${{price}}</li> {{/product}} </ul>
Next, the data object:
{ "product": [ { "name": "SuperWidget", "price": "19.99" }, { "name": "WonderWidget", "price": "24.99" }, { "name": "MegaWidget", "price": "29.99" } ] }
And finally, the resulting output:
<ul> <li>SuperWidget: $19.99</li> <li>WonderWidget: $24.99</li> <li>MegaWidget: $29.99</li> </ul>
You can try out this example by pressing the button below:
Adding comments in Mustache
To insert a comment in a Mustache template, use the following syntax:
{{! this is a comment }}
The whole comment tag is ignored by the Mustache processor.
Including Mustache templates with partials
Partials allow you to include one Mustache template inside another. This lets you keep your templates modular and organized.
In the JavaScript version of Mustache, you create an object containing all your partials:
var partials = { myPartial: "templateString", anotherPartial: "templateString" ... };
Then, to insert a partial at a given point in a template, you use the syntax:
{{>partialName}}
So to include the partial called "myPartial"
, you’d write:
{{>myPartial}}
You can also include a partial within another partial, which lets you make nested includes.
Then, when you run the Mustache processor, you pass your partials object as the third argument, like this:
html = Mustache.render( template, data, partials );
Here’s an example of partials in action. First, we’ll create a couple of partials, productInfo
and buyLink
:
var partials = { productInfo: " <ul> <li>Name: {{name}}</li> <li>Colour: {{colour}}</li> <li>Price: ${{price}}</li> {{>buyLink}} </ul> ", buyLink: " {{#inStock}} <li><a href='#'>Buy Now!</a></li> {{/inStock}} {{^inStock}} <li>Sorry, out of stock.</li> {{/inStock}} " };
productInfo
displays a product’s name, colour and price, It also includes the buyLink
partial, which displays the “Buy Now!” link (or the “Sorry, out of stock” message if the product is out of stock).
Now let’s create our template:
var template = "<h3>Product: {{name}}</h3> {{>productInfo}}";
This template displays the product name inside an h3
element, then includes the productInfo
partial to display the product info.
Here’s the data object that we’ll use for our product data:
var data = { "name": "SuperWidget", "colour": "Green", "price": "19.99", "inStock": true }
Finally, we call the Mustache render()
method to create the final markup:
html = Mustache.render( template, data, partials ); alert( html );
This displays the following alert box:
<h3>Product: SuperWidget</h3> <ul> <li>Name: SuperWidget</li> <li>Colour: Green</li> <li>Price: $19.99</li> <li><a href='#'>Buy Now!</a></li> </ul>
Bringing it all together
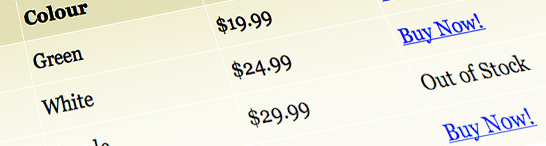
Let’s build a complete JavaScript demo that shows off some of Mustache’s great features. We’ll create a simple product list page with a Get Products button. When the user clicks the button, the JavaScript code uses Ajax to fetch the product data from the server, then uses a Mustache template to display the product data in an HTML table.
The HTML page
First, create the main page for the product list demo. Save the following code as productList.html
in a folder in your website. Also, copy the mustache.js
and style.css
files that you used earlier in the tutorial into the same folder.
<!doctype html> <html> <head> <title>Complete Mustache Demo: Product List</title> <meta charset="utf-8"> <link rel="stylesheet" href="style.css" /> <script type="text/javascript" src="http://code.jquery.com/jquery-1.7.1.min.js"></script> <script type="text/javascript" src="mustache.js"></script> <script type="text/javascript"> var template, data, html; var gotTemplate, gotData; function getProducts() { gotTemplate = gotData = false; $.get( "productListTemplate.mustache", null, function( ajaxData ) { template = ajaxData; gotTemplate = true; if ( gotData ) processTemplate(); } ); $.getJSON( "products.txt", null, function( ajaxData ) { data = ajaxData; gotData = true; if ( gotTemplate ) processTemplate(); } ); } function processTemplate() { html = Mustache.render( template, data ); $('#productList').html( html ); } </script> <style> table { width: 100%; border-spacing: 1px; border: none; } th { text-align: left; background-color: #EFEFD7; color: #000000; font-weight: bold; padding: 4px; } td { text-align: left; background-color: #F7F7E6; color: #000000; font-weight: normal; padding: 4px; } button { margin-bottom: 20px; } </style> </head> <body> <h1>Complete Mustache Demo: Product List</h1> <button onclick="getProducts()">Get Products</button> <div id="productList"> </div> </body> </html>
The page’s body contains:
- A heading
- A Get Products button that, when pressed, calls the JavaScript function
getProducts()
, and - An empty
#productList
div
that will contain the products table.
The page also contains the JavaScript to fetch and display the product list. First the JavaScript sets up some variables:
template
,data
andhtml
will hold the Mustache template, the data object, and the final product list HTML respectively.gotTemplate
andgotData
will be used to track when the JavaScript has finished fetching the Mustache template and the product data respectively. When both have been fetched, the list can be displayed.
The getProducts()
function runs when the user presses the Get Products button. It makes two calls to jQuery Ajax methods in order to retrieve two files from the server:
productListTemplate.mustache
The function fetches this file using the jQueryget()
method. This is the Mustache template file for the products list; we’ll create this file in a moment.products.txt
This file is fetched using the jQuerygetJSON()
method. This method works likeget()
, but is specifically designed for retrieving JSON data, which it then parses and turns into a JavaScript object.products.txt
contains the product data in JSON format. Again, we’ll create this in a minute.
With both Ajax requests, the code passes in an anonymous callback function that runs once the request completes. Each function does the following:
- It stores the returned data in the appropriate variable (
template
ordata
). - It set the appropriate tracking variable to
true
(gotTemplate
orgotData
). - If both tracking variables have been set to
true
, it callsprocessTemplate()
to display the product list.
If you’re new to Ajax programming or jQuery, check out Ajax with jQuery: A Beginner’s Guide.
Finally, the processTemplate()
function calls Mustache.render()
to create the product list markup, passing in the Mustache template stored in template
, and the data object stored in data
. It stores the markup in the html
variable, which it then passes to jQuery’s html()
method to insert the markup into the #productList
div
, displaying the table to the user.
The Mustache template
Here’s the Mustache template that displays the product list table — save it as productListTemplate.mustache
in the same folder as productList.html
:
<table> <tr> <th>Product</th> <th>Colour</th> <th>Price</th> <th>Buy</th> </tr> {{#product}} <tr> <td>{{name}}</td> <td>{{colour}}</td> <td>${{price}}</td> {{#inStock}}<td><a href="buy.php?productId={{id}}">Buy Now!</a></td>{{/inStock}} {{^inStock}}<td>Out of Stock</td>{{/inStock}} </tr> {{/product}} </table>
As you can see, the template is HTML markup interspersed with Mustache tags. The template comprises:
- A header row containing the column headers: Product, Colour, Price and Buy.
- A
{{#product}} ... {{/product}}
Mustache section. This section displays a row of product data in the table. Since theproduct
property in our data object is an array of objects (as you’ll see in a moment), this section will run repeatedly to display all the rows in the products table.The section contains:-
- Mustache variables to display the product name, colour, and price.
- Two conditional sections. The markup between
{{#inStock}} ... {{/inStock}}
is displayed if theinStock
variable istrue
; it comprises a dummy “Buy Now!” link that uses theid
variable to identify the product. The markup between{{^inStock}} ... {{/inStock}}
is displayed ifinStock
isfalse
; it simply displays an “Out of Stock” message.
-
The data file
The last file we need to create contains the product data in JSON format. Save the following code as products.txt
in the same folder as productList.html
:
{ "product": [ { "id": 1, "name": "SuperWidget", "colour": "Green", "price": "19.99", "inStock": true }, { "id": 2, "name": "WonderWidget", "colour": "White", "price": "24.99", "inStock": true }, { "id": 3, "name": "MegaWidget", "colour": "Purple", "price": "29.99", "inStock": false }, { "id": 4, "name": "HyperWidget", "colour": "Yellow", "price": "49.99", "inStock": true } ] }
This file defines a JSON object that contains a product
array. The product
array contains four elements, each of which is an object representing a product. Each product object contains id
, name
, colour
, price
and inStock
properties.
In a real-world site or app, this file would likely be dynamically generated by a server-side script that pulls the data from a database, rather than stored as a static file on the server.
Try it out!
To try out the product list demo, browse to the URL for the productList.html
file in your website — for example, http://mywebsite/productList.html
— or press the button below to see it running on our server:
Since the demo uses Ajax to fetch the template and data files, you need to view the demo running on a web server. Opening the productList.html
file directly in your browser probably won’t work.
Now press the Get Products button in the page. When you do this, the getProducts()
function runs, retrieving the productListTemplate.mustache
and products.txt
files from the server via Ajax. Once the files have been fetched, processTemplate()
calls Mustache’s render()
method to combine the Mustache template with the product data and produce the product list table, which is then displayed in the page:

Summary
In this article you’ve explored the Mustache template system, and seen how you can easily use it to build clean, logic-less templates. You’ve looked at:
- What Mustache is, and why it’s useful.
- A basic example of a Mustache template with tags and variables, as well as a data object.
- How to install the Mustache processor on your website.
- Running the processor by calling the
render()
method. - Building a simple demo page that you can use to try out various Mustache features.
- Using expressions and functions in data objects.
- How to access object properties and methods in your Mustache templates.
- What happens when Mustache can’t find a variable.
- How Mustache escapes HTML characters, and how to bypass escaping.
- The concept of Mustache sections, including conditional sections, inverted sections, and repeating sections (loops).
- How to add comments in Mustache templates.
- Including one Mustache template inside another by using partials.
- A complete example that uses JavaScript, jQuery, Ajax and Mustache to build a simple product list page.
There are a few more Mustache features worth checking out, including lambdas (which let you manipulate un-parsed sections of Mustache templates) and the ability to change the default Mustache delimiters, {{
and }}
, to something else. To find out more, take a look at the Mustache spec.
Have fun!
[Photo credit: thos003]
This is cool technology for templates.
I will surely invest time in this complete tutorial.
I have used demos.
Can anyone tell me list of tool or tools from which i can make my own logo with ease?
I am not a designer, just need for my clients.
So, for this i am not going to learn photoshop like tools.
Mean while, Thanks Matt for introduction of Mustache!
Matt
Great tutorial.
What is the best way to use Mustache with your ‘CMS in an Afternoon’?
Thanks
Phil
Matt
Please, could you redo this tutorial but in PHP?
Thanks again
Phil
@philatnotable: Never done it, but it should be straightforward. Grab the PHP implementation here: https://github.com/bobthecow/mustache.php
Then just create your Mustache templates in the CMS templates folder, and replace the simple template require() calls in the PHP code with calls to $m->render(), passing in the loaded template and an array containing the appropriate variables for the template.
Thanks Matt,
I can get Mustache to work, following your direction, up until I try to turn this:
return ( array ( “results” => $list, “totalRows” => $totalRows[0] )
into a simple Mustache ready array.
Regards, Phil
That statement is a closing paranthesis short.
Great Tutorial! Made my day.Thanks!
Keep up the good work
hi ,
This is great tutorial.
i have one question.
Step1: I have created a java view class called “myView”.
Step2: I have created mustache template called “myView.mustache”
step3: I have created java view variable called “myVar” in my view class and assigned a string which has html tags .
step4 : in my “myView.mustache” template i am trying to use my variable by doing {{myVar}}.
Step5: After running my app, i am seeing “myVar” stuff as a string and not a html.
myVar is looks like this
<div class=”para-3″>
<div class=”para-info”>
<a target=”_blank” href=”http://abc.com”>This is paragraph information.</a>
</div>
</div>
my expectation is i should see “myVar” stuff as a html not like just plain string.
i am not sure what i am doing wrong.why my page is not rendering as HTML for “myVar” stuff .
suggestions are appreciated.
thanks
Chary
Do you mean javascript? Because “Java” and “javascript” are two very different things.
How are you trying to produce the output of your variable?
Hi,
Thanks for the tutorial! I’m new at this and I was wondering if anybody else has had the following error:
“Invalid template! Template should be a “string” but “object” was given as the first argument for mustache#render(template, view, partials)”
There is unfortunately little information about it and my code is exactly as that which is posted in the tutorial.
Could anyone help me with this? Thanks in advance!
Raquel.
You have used a reference to the object rather a name or ID value that represents the object.
I don’t use this ‘mustache’ so this is a guess, but you have probably used ObjVar (the object) rather than ObjVar.Name (a string property of the object.)
Hi, i tried but when i take my title from wordpress site with json it gave me the wrong title like
… ‘Striscia’ …
as you can see the ‘ are not showned well but in the text they are -> … tv ‘Striscia la notizia’, che …
If i has a project with html templates and loaders that uses mustache render without partial and i cannot change the render function, is there other ways to render a template html that include other templates??