Many modern websites — and practically all web apps — use a technique called Ajax to provide a smooth, fast experience for the user. From apps like Gmail and Google Maps through to JavaScript frameworks like jQuery Mobile, Ajax is a popular way to fetch data from a server in the background and update the page dynamically.
Writing Ajax JavaScript code from the ground up can be fiddly. However, many JavaScript libraries, including jQuery, have excellent high-level support for Ajax, making it easy for you to build Ajax-driven websites and apps with minimal hassle.
In this tutorial, you’ll learn the basics of making Ajax requests using jQuery. You’ll look at:
- Exactly what Ajax is, how it works, and why it’s a good thing
- How to make various types of Ajax requests using jQuery
- Sending data to the server along with an Ajax request
- Handling the Ajax response from the server, and capturing any data returned in the response
- How to customize jQuery’s Ajax handling and change default settings, and
- A few links to some more advanced Ajax-related info and tips.
To keep things simple, this tutorial concentrates on the JavaScript side of things, rather than delving into server-side coding. However, it’s pretty easy to write server-side scripts that work with Ajax. If you want to learn a server-side language, check out my PHP tutorials.
Ready to learn Ajax? Let’s get started!
What is Ajax, and why is it useful?
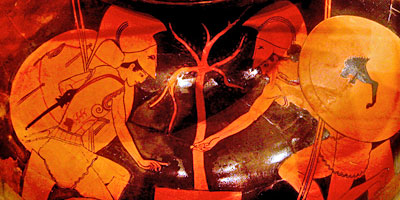
Ajax is a web development technique in which JavaScript code running in the browser communicates with the web server asynchronously — that is, in the background. It differs from conventional web programming in the following ways:
- A conventional web page contains links or forms that, when clicked or sent, make a request to a new URL on the web server. The server then responds by sending back a whole new HTML page, which the browser then displays, replacing the original page. This can be time-consuming and disruptive to the user, since the user has to wait while the new page loads.
- With the Ajax approach, JavaScript code makes a request to a URL on the server. The code can also send data along with the request. The JavaScript code then handles the server’s response, and acts appropriately. For example, it might perform some calculations on the returned data; add or update some widgets in the page; or notify the user that their changes were saved in the server’s database.
Since an Ajax request happens in the background, the JavaScript code (and the user) can continue working with the page while the request is happening. The whole process is invisible to the user, and the user never needs to leave the page they’re currently viewing. This makes Ajax-enabled pages smoother and nicer to use, and means that Ajax web apps can more closely resemble desktop apps in the way they behave.
The cornerstone of Ajax is the catchily-named XMLHttpRequest
JavaScript object. This object provides various methods, such as open()
, send()
and onreadystatechange()
, that can be used to make an Ajax request to the server and handle the response in the background.
Writing reliable cross-browser Ajax JavaScript code can get quite tedious. Luckily, jQuery gives you some easy-to-use Ajax methods that abstract away a lot of the low-level processes.
Let’s start by looking at one of the most useful of these methods: $.get()
.
In case you’re wondering, “Ajax” stands for Asynchronous JavaScript And XML. However, the term is somewhat misleading — requests don’t have to be asynchronous, and you don’t have to use XML to send data.
Making GET requests with $.get()

jQuery’s $.get()
method gives you an easy, convenient way to make simple Ajax requests. As the name implies, it makes the request using the HTTP GET method (used for retrieving URLs such as web pages and images), rather than the POST method (which is traditionally used for sending form data).
In its simplest form, you call the method like this:
$.get( url );
…where url
is the URL of the resource that you want to retrieve. Typically, this will be a server-side script that performs some action on the server, and probably returns some data:
$.get( "http://example.com/getForecast.php" );
…although you could just request a static document, too:
$.get( "http://example.com/mypage.html" );
As well as requesting a URL, you can send some data along with the request. You can pass this data in a query string, just as you do with regular GET requests:
$.get( "http://example.com/getForecast.php?city=rome&date=20120318" );
A cleaner way to do the same thing is to pass a data object as the second parameter to $.get()
. The data object should contain the data to send, as property name/value pairs. Here’s an example:
var data = { city: "rome", date: "20120318" };
$.get( "http://example.com/getForecast.php", data );
Alternatively, you can pass the data to $.get()
as a string, like this:
var data = "city=rome&date=20120318";
$.get( "http://example.com/getForecast.php", data );
Retrieving data from the server
So far, the $.get()
examples you’ve seen merely make requests to the server, ignoring any response that might be sent by the server-side script. Most of the time, however, your JavaScript will want to retrieve the response sent by the server-side script, and do something with the returned data.
Remember that Ajax requests are asynchronous, which means that they happen in the background while the rest of your JavaScript code continues to run. So how can your JavaScript code retrieve the response from the server once the request completes?
The answer is that you need to write a callback function that will be run automatically once the Ajax request has been completed and the server has sent the response. At a minimum, your function should accept the data returned from the server as its first argument, like this:
function myCallback( returnedData ) {
// Do something with returnedData here
}
Once you’ve created your callback function, you pass it as the third argument to $.get()
:
var data = { city: "rome", date: "20120318" };
$.get( "http://example.com/getForecast.php", data, myCallback );
Specifying the response data type
Data is typically returned from a server-side script in one of several common formats, including XML, JSON, JavaScript, or HTML. By default, jQuery does its best to guess the returned data format and parse it appropriately; however it’s a good idea to specify a format if possible.
To specify a data format, pass a fourth parameter to $.get()
. This parameter can be one of the following strings:
"xml"
"json"
"script"
"html"
For example, if you know that your server-side script returns data in JSON format, you can call $.get()
like this:
$.get( "http://example.com/getForecast.php", data, myCallback, "json" );
A complete $.get()
example
Here’s a complete example that shows how to make an Ajax request with $.get()
and retrieve the response. First, create a simple text file on your server called getForecast.txt
, containing the following text:
{
"city": "Rome",
"date": "18 March 2012",
"forecast": "Warm and sunny",
"maxTemp": 28
}
This file simulates the JSON response that might be returned by a real weather forecast script running on a server.
Next, save the following page as showForecast.html
in the same folder as getForecast.txt
:
<!doctype html> <html lang="en"> <head> <title>Weather Forecast</title> <meta http-equiv="Content-Type" content="text/html;charset=utf-8"> </head> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js"></script> <script> $( function() { $('#getForecast').click( function() { var data = { city: "rome", date: "20120318" }; $.get( "getForecast.txt", data, success, "json" ); } ); function success( forecastData ) { var forecast = forecastData.city + " forecast for " + forecastData.date; forecast += ": " + forecastData.forecast + ". Max temp: " + forecastData.maxTemp + "C"; alert( forecast ); } } ); </script> </head> <body> <button id="getForecast">Get Forecast</button> </body> </html>
Open showForecast.html
in your browser, or press the button below, then press the Get Forecast button. You’ll see the weather forecast appear in an alert box.
Here’s how the code works:
showForecast.html
contains a Get Forecastbutton
element with an ID ofgetForecast
.- The JavaScript at the top of the page runs once the page has loaded and the DOM is ready.
- The JavaScript first attaches a
click
handler to the#getForecast
button. Thisclick
handler makes an Ajax GET request togetForecast.txt
, passing in the city and date to get the forecast for. It also specifies a callback function,success()
, that should be run once the request completes, and specifies that the server response will be in JSON format. - The
getForecast.txt
file returns the forecast data in JSON format to the browser. - The
success()
callback function is called. jQuery parses the JSON data returned fromgetForecast.txt
, converts it into a JavaScript object, and passes it to the function. - The function retrieves the data object in
forecastData
and displays an alert box containing the various object properties, including the city name, the date, the forecast, and the temperature.
With this example, you can see how easy it is to make Ajax requests with $.get()
using just a few lines of code.
Making POST requests with $.post()
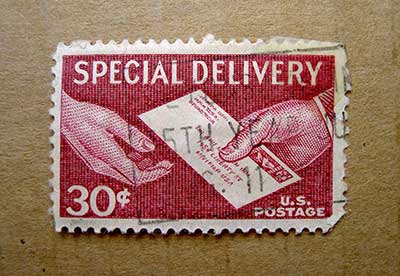
Just as $.get()
lets you make Ajax requests using the GET HTTP method, $.post()
makes requests with the POST HTTP method. The syntax is identical to $.get()
; the only difference between the two functions is that $.post()
uses the POST method.
For example, we could rewrite our “weather forecast” $.get()
call above to use $.post()
, like this:
$.post( "getForecast.txt", data, success, "json" );
Try this out by pressing the button below. Notice that when you press the Get Forecast button in the page, it displays the same weather forecast alert box as the previous example.
As I mentioned earlier, the POST HTTP method is commonly used to send form data. The reason is that the GET method only allows you to send a small amount of data (typically a few hundred characters), whereas POST can send as much data as you like. So if you need to send a large amount of data with your Ajax request, use $.post()
.
Loading markup into the page with load()

jQuery’s handy load()
method lets you easily retrieve HTML markup from the server via Ajax, and insert it into the current page automatically. If your server-side script does all the work of processing data and creating the markup, and you just want to display the result in the page, then load()
is the method to use.
In its simplest form, you call load()
like this:
$('#myElement').load( url );
This makes an Ajax request to url
, retrieves the markup returned from the server, and replaces the contents of #myElement
with the markup.
You can also pass data in the request, just like $.get()
and $.post()
:
var data = { city: "rome", date: "20120318" };
$('#myElement').load( url, data );
A complete load()
example
Let’s modify our previous “weather forecast” example so that the server-side “script” creates markup for the forecast. Then we can just call load()
to display the markup in the page. First, save the following markup as getForecast.html
in your website:
<h2>Rome Weather Forecast</h2> <h3>18 March 2012</h3> <p>It will be warm and sunny. Max temperature: 28 degrees C.</p>
getForecast.html
simulates the effect of a server-side script running and returning the markup for the forecast.
Now save the following file as showForecast.html
in the same folder as getForecast.html
:
<!doctype html> <html lang="en"> <head> <title>Weather Forecast</title> <meta http-equiv="Content-Type" content="text/html;charset=utf-8"> </head> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js"></script> <script> $( function() { $('#getForecast').click( function() { var data = { city: "rome", date: "20120318" }; $('#forecast').load( "getForecast.html", data ); } ); } ); </script> </head> <body> <button id="getForecast">Get Forecast</button> <div id="forecast"></div> </body> </html>
To try this script out, press the button below, then press the Get Forecast button in the page. You’ll see the weather forecast appear below the button within the page.
Let’s look at how this code works:
- The page contains an extra
div
with anid
offorecast
, which will contain the forecast markup. - The
getForecast
button’sclick
handler creates adata
object holding the data to send to the server side script. - The handler then selects the
#forecast
div
and calls the jQueryload()
method on it, passing in the URL to retrieve (getForecast.html
) as well as thedata
object. - jQuery then makes an Ajax request to
getForecast.html
. When the browser receives the response, jQuery automatically replaces the contents of#forecast
with the markup returned in the response.
One reason load()
is so easy to use is that you don’t have to write a callback function to handle the response — jQuery handles it for you. However, if you also need to do other stuff after the markup is inserted, you can pass a “complete” callback function as the third argument to load()
. See the jQuery API docs for details.
Loading just part of a page
Sometimes your server might return more markup than you need. For example, you might want to request an article page from the server, and just insert the article body in the current page, rather than the entire returned document.
jQuery’s load()
method makes this easy. Just add a space to the end of the URL to request, followed by a selector that selects just the portion of the page you want to insert. jQuery then retrieves the entire document via Ajax, but only inserts the selected portion of the markup into the current page.
Here’s an example:
$('#articleContainer').load( "newArticle.html #articleBody" );
This can be a good way to retrieve new pages from the server. It’s nice and smooth, since the entire page isn’t redrawn each time. You can also display a friendly “loading” message to the user, so that they know something is happening. For example, you could add a “Loading, please wait” message inside #articleContainer
, then call load()
to load the new content into #articleContainer
. Once the new markup is retrieved, the “Loading, please wait” message is automatically replaced by the article content.
Getting JSON data with $.getJSON()

$.getJSON()
gives you an easy way to request JSON data from the server. It’s the equivalent to calling $.get()
with the "json"
data format parameter. The syntax is identical to $.get()
, except that you don’t need to specify the data format.
For example, we can rewrite our earlier $.get()
weather forecast click
handler like this:
$('#getForecast').click( function() { var data = { city: "rome", date: "20120318" }; $.getJSON( "getForecast.txt", data, success ); } );
Try this out by pressing the button below. Press the Get Forecast button in the page to make the JSON request and display the forecast.
Getting and running JavaScript code with $.getScript()
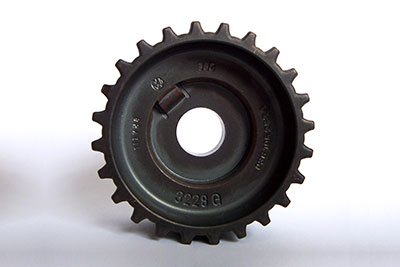
Just as $.getJSON()
is a shorthand version of $.get()
for retrieving JSON data, $.getScript()
is a shorthand version of $.get()
that retrieves and executes JavaScript code. It’s similar to passing the "script"
data format parameter to $.get()
. $.getScript()
takes just two arguments:
- The URL of the JavaScript file to load
- An optional callback function that runs once the JavaScript has been loaded and run
$.getScript()
is useful for loading JavaScript libraries and plugins on the fly, as and when they’re needed. This can reduce your page’s initial load time, since you don’t need to include every JavaScript library that you might want to use in your page’s head element.
To try out $.getScript()
, let’s move the code that displays our weather forecast alert box into a separate JavaScript library file, showForecast.js
. We’ll wrap the code in a function called showForecast()
:
function showForecast( forecastData ) { var forecast = forecastData.city + " forecast for " + forecastData.date; forecast += ": " + forecastData.forecast + ". Max temp: " + forecastData.maxTemp + "C"; alert( forecast ); }
We can then modify the showForecast.html
page to retrieve our JavaScript library using $.getScript()
, then add the click
handler to the button once the script has loaded:
<!doctype html> <html lang="en"> <head> <title>Weather Forecast</title> <meta http-equiv="Content-Type" content="text/html;charset=utf-8"> </head> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js"></script> <script> $( function() { $.getScript( "showForecast.js", function() { $('#getForecast').click( function() { var data = { city: "rome", date: "20120318" }; $.get( "getForecast.txt", data, showForecast, "json" ); } ); } ); } ); </script> </head> <body> <button id="getForecast">Get Forecast</button> </body> </html>
Try this out by pressing the button below, then pressing the Get Forecast button. You’ll see the forecast alert box appear as it did in our original $.get()
example.
The JavaScript code in showForecast.html
starts by calling $.getScript()
to load and run the showForecast.js
library file. It also creates an anonymous callback function that runs once showForecast.js
has loaded. This function adds a click
handler to the #getForecast
button, which calls $.get()
to retrieve getForecast.txt
and run the loaded showForecast()
function to display the alert.
$.getScript()
appends a random timestamp parameter to the query string in the request — for example, ?_=1330395371668
— to prevent the browser caching the JavaScript file.
Making generic Ajax requests with $.ajax()
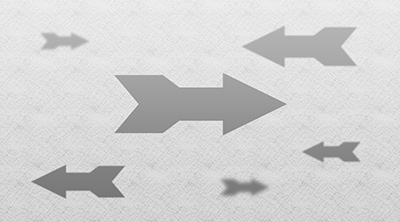
The high-level Ajax methods you’ve looked at so far in this tutorial — $.get()
, $.post()
, load()
, $.getJSON()
and $.getScript()
— give you easy, convenient ways to make various common types of Ajax requests. However, sometimes you may need more control over a request, or you may need to do something that isn’t possible with these higher-level methods.
In these situations, you’ll need to use jQuery’s low-level $.ajax()
method. As with the other methods mentioned above, calling $.ajax()
makes an Ajax request to a specified URL. Here’s the syntax:
$.ajax( url [, settings] );
url
is, of course, the URL to request, while settings
is an optional object containing various settings that let you control exactly how the request works.
Here’s a sample of the settings you can specify with $.ajax()
:
Setting | Description | Default Value |
---|---|---|
cache |
Set to true to cache the response from the server, or false to always make the request. A value of false also makes jQuery append a random timestamp parameter to the request, as it does with $.getScript() , to ensure the browser doesn’t cache the response. |
true (false when used with the 'script' and 'jsonp' dataType setting) |
complete |
Specifies a callback function to run when the request completes (whether successful or not). | None |
data |
Any data to be sent to the server in the request. This works in the same way as $.get() ‘s data argument. |
None |
dataType |
The type of data to expect in the response. This works in the same way as $.get() ‘s fourth argument. You can also specify a value of "jsonp" to make a JSONP request. |
Intelligent guess |
error |
Specifies a callback function to run if the request fails. | None |
headers |
Additional HTTP headers to send with the request, as a map of key/value pairs. | {} |
password |
A password to use if the server requires HTTP authentication. | None |
success |
Specifies a callback function to run if the request succeeds. This is the same as the third argument passed to $.get() . |
None |
timeout |
How long, in milliseconds, to wait for the Ajax request to complete. A value of zero means jQuery will wait indefinitely. | 0 |
type |
The type of request to make: "GET" or "POST" . |
"GET" |
username |
A username to use if the server requires HTTP authentication. | None |
For a full list of Ajax settings, see the jQuery documentation.
Let’s try an example. By using $.ajax()
instead of $.get()
, we can enhance our weather forecast example to disable caching and handle errors. Here’s the modified showForecast.html
page:
<!doctype html> <html lang="en"> <head> <title>Weather Forecast</title> <meta http-equiv="Content-Type" content="text/html;charset=utf-8"> </head> <script src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js"></script> <script> $( function() { $('#getForecast').click( function() { var data = { city: "rome", date: "20120318" }; $.ajax( "getForecast.txt", { cache: false, data: data, dataType: "json", error: errorHandler, success: success } ); } ); function success( forecastData ) { var forecast = forecastData.city + " forecast for " + forecastData.date; forecast += ": " + forecastData.forecast + ". Max temp: " + forecastData.maxTemp + "C"; alert( forecast ); } function errorHandler() { alert( "There was a problem retrieving the forecast." ); } } ); </script> </head> <body> <button id="getForecast">Get Forecast</button> </body> </html>
Try this out by pressing the button below, then pressing the Get Forecast button in the page. You should see the forecast appear as before.
In this example we use $.ajax()
to make the Ajax request, instead of $.get()
. We pass the URL to request (getForecast.txt
), followed by a list of options. Three of these options — data
, dataType
and success
— are the same as the arguments passed to $.get()
earlier in the tutorial. The remaining two — cache: false
and error: errorHandler
— turn off caching and set the errorHandler()
callback function to handle errors respectively.
We’ve also defined our errorHandler()
callback function, which simply displays an error message to the user.
Specifying default settings using $.ajaxSetup()

Rather than specifying various settings, such as success
, cache
and type
, every single time you make an Ajax call, you can use the $.ajaxSetup()
method to specify these settings by default. All future jQuery Ajax requests will then use these settings, unless overridden.
For example, you can turn off caching by default for all future Ajax requests like this:
$.ajaxSetup( {
cache: false
} );
Summary
In this article you learned how to use jQuery to build Ajax-driven websites. You looked at:
- How Ajax works, and some of Ajax’s advantages.
- Using jQuery’s
$.get()
method to request URLs using the HTTP GET method. - How to pass data along with an Ajax request.
- How to write a callback function, and capture data returned from the server.
- Specifying the type of data to expect in the server response.
- Making HTTP POST requests using
$.post()
. - jQuery’s
load()
method, which gives you an easy way to retrieve HTML via Ajax and inject it into the page. - Retrieving JSON data with the
$.getJSON()
method. - Retrieving and running JavaScript code with the
$.getScript()
method. - Using jQuery’s flexible
$.ajax()
method to make any kind of Ajax request. - Specifying default Ajax settings with the
$.ajaxSetup()
method.
Further reading
You’ve now explored the basics of making Ajax requests using jQuery. However, there’s a lot more Ajax-related stuff under jQuery’s hood. If you want to take things further, check out the following links:
- Other jQuery Ajax methods: There are many more handy jQuery methods for working with Ajax, including methods for attaching handlers to various Ajax events, as well as some useful helper methods for serializing data to make it Ajax-ready.
- The
jQXHR
object: jQuery’sjqXHR
object is returned by the$.ajax()
method, and is also passed to various callback functions. Using this object, you can access the raw response headers and data returned from the server; override the MIME type returned by the server; and create queues of callback functions. - Cross-domain Ajax: By default, Ajax requests can only be made to URLs on the same server as the page containing the Ajax JavaScript — a restriction known as the same origin policy. There are two ways round this restriction: JSONP, which wraps the returned JSON object in a JavaScript function call that can then work across servers, and cross-origin resource sharing, a new W3C spec that provides a cleaner way to share resources across different web servers and domains.
I hope you enjoyed this introduction to the world of Ajax programming in jQuery. Have fun!
[Achilles & Ajax photo credit: clairity]
Please note that some info is not up-to-date. Under “Making generic Ajax requests with $.ajax()†complete, error, success
callback methods are being presented. These will be deprecated in next jquery version (1.8). Better to use done(), fail() that can be chained due to the fact $.ajax now returns a jquery promise.
From:
http://api.jquery.com/jQuery.ajax/
Deprecation Notice: The jqXHR.success(), jqXHR.error(), and jqXHR.complete() callbacks will be deprecated in jQuery 1.8. To prepare your code for their eventual removal, use jqXHR.done(), jqXHR.fail(), and jqXHR.always() instead.
@hvaleanu: Interesting, I didn’t know those were going to be deprecated. I’ll update the article when I get a chance. Thanks for the info!
I wrote an article about simple ajax requests with jQuery sometime ago… not as comprehensive as this one though. Thank you very much for all the details.
Matt,
I realize that this is an older posting, but I must say, WELL DONE! Concise, purpose-driven progressions that do exactly what is needed; give understanding and familiarity. I wish EVERY guide was written in this manner. WRITE MORE STUFF. Go rub off some of that awesomeness on to the Oracle documentation people. Thank you very much.