One of the lovely things about jQuery is it makes it ridiculously easy to animate page elements. Whereas you previously had to mess around with setTimeout()
or setInterval()
, and lots of JavaScript code, with jQuery you can work wonders with just a line or two of code.
In this tutorial we’ll cover some of the more common effects you can achieve with jQuery. We’ll look at:
- Fading elements in and out
- Showing and hiding elements
- Sliding elements up and down
- Animating any numeric CSS property
- Creating an animation sequence
- Inserting delays into an animation, and
- Stopping animations.
Ready? Let’s get going!
Fading elements: fadeIn()
, fadeOut()
and fadeTo()
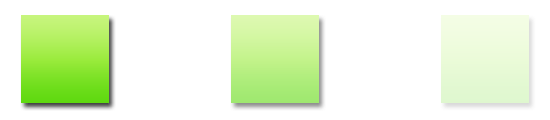
jQuery has 2 easy-to-use functions for fading text, images, or other page elements in and out:
fadeIn( [duration] )
- Fades the selected element(s) from transparent to opaque
fadeOut( [duration] )
- Fades the selected element(s) from opaque to transparent
The optional duration
parameter lets you change the speed of the animation. You can pass any of the following values:
'fast'
- Performs the animation in 200 milliseconds
'slow'
- Performs the animation in 600 milliseconds
- An integer value
- Performs the animation in the specified number of milliseconds
The default duration is 400 milliseconds.
You can also use the fadeTo()
function to fade an element to a specific opacity value. To use it, you pass the duration (required) followed by the target opacity (between 0 and 1).
Here’s an example that shows how fadeIn()
, fadeOut()
and fadeTo()
work:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript">
$( init );
function init() {
$('#fadeIn').click( function() { $('#myText').fadeIn(); } );
$('#fadeOut').click( function() { $('#myText').fadeOut(); } );
$('#fade50').click( function() { $('#myText').fadeTo( 400, .5 ); } );
}
</script>
</head>
<body>
<blockquote id="myText">I think he's right, there is something about this, that's... that's so black, it's like, "How much more black could this be?" and the answer is: "None, none... more black."</blockquote>
<ul>
<li><a id="fadeIn" href="#">Fade in</a></li>
<li><a id="fadeOut" href="#">Fade out</a></li>
<li><a id="fade50" href="#">Fade to 50% opacity</a></li>
</ul>
</body>
</html>
Watch out: fadeIn()
will only fade an element up to its existing opacity value. For example, if you set an element’s opacity to 0.5 and hide it with display: none
, then you call fadeIn()
, the element will only fade up to 50% opacity, not 100%.
fadeOut()
, on the other hand, always fades an element out to zero opacity. After fading out, it also rather handily sets the element to display: none
so that it no longer takes up any space in the page.
Showing and hiding elements: show()
and hide()
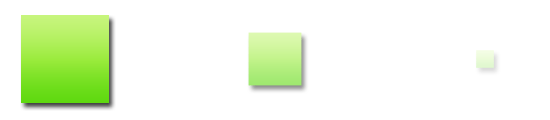
jQuery’s show()
and hide()
methods let you reveal and hide elements respectively. Called without any parameters, they instantly display or hide the selected element(s). However, if you pass a duration parameter then jQuery gradually animates the width, height and opacity of the element(s).
Here’s an example that demonstrates these different ways of using show()
and hide()
:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript">
$( init );
function init() {
$('#show').click( function() { $('#myText').show(); } );
$('#hide').click( function() { $('#myText').hide(); } );
$('#showSlow').click( function() { $('#myText').show('slow'); } );
$('#hideSlow').click( function() { $('#myText').hide('slow'); } );
}
</script>
</head>
<body>
<blockquote id="myText">I think he's right, there is something about this, that's... that's so black, it's like, "How
much more black could this be?" and the answer is: "None, none... more black."</blockquote>
<ul>
<li><a id="show" href="#">Show</a></li>
<li><a id="hide" href="#">Hide</a></li>
<li><a id="showSlow" href="#">Show slowly</a></li>
<li><a id="hideSlow" href="#">Hide slowly</a></li>
</ul>
</body>
</html>
Sliding elements up and down: slideUp()
, slideDown()
and slideToggle()

slideUp()
and slideDown()
hide and show elements by animating their heights. slideUp()
hides an element by reducing its height to zero, while slideDown()
reveals an element by increasing its height from zero up to its normal height.
This effect is great for things like accordions, where you want to reveal and hide blocks of content in a list.
As with fadeIn()
and its friends, you can specify an optional duration argument. If you leave out the duration then the animation defaults to 400 milliseconds.
Once slideUp()
has finished hiding an element, it also sets the element’s display
value to none
.
slideToggle()
toggles the state of an element. If the element is currently hidden, it is revealed by sliding it down. If it’s currently visible, it’s hidden by sliding it up. Once again, you can specify a duration argument, or leave the duration at the default of 400 milliseconds.
Here’s an example that shows how to use slideUp()
, slideDown()
and slideToggle()
:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript">
$( init );
function init() {
$('#slideUp').click( function() { $('#myText').slideUp(); } );
$('#slideDown').click( function() { $('#myText').slideDown(); } );
$('#slideToggle').click( function() { $('#myText').slideToggle(); } );
}
</script>
</head>
<body>
<blockquote id="myText">I think he's right, there is something about this, that's... that's so black, it's like, "How
much more black could this be?" and the answer is: "None, none... more black."</blockquote>
<ul>
<li><a id="slideUp" href="#">Slide up</a></li>
<li><a id="slideDown" href="#">Slide down</a></li>
<li><a id="slideToggle" href="#">Slide up/down</a></li>
</ul>
</body>
</html>
Animating any CSS property: animate()
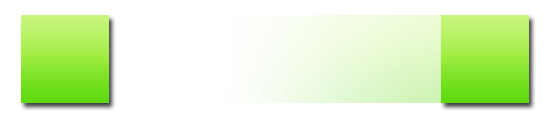
Whereas the previous jQuery methods animate specific properties of an element β such as opacity and height β the animate()
method can animate any CSS property that takes a numeric value. You can even animate several properties of an element at once with a single call to animate()
. Nice!
To specify the property or properties to animate, you pass a map of name/value pairs to animate()
. For example:
$('#myDiv').animate( {
property1: value1,
property2: value2,
...
} );
The method usually assumes your values are in pixels if you don’t specify a unit. You can also specify percentages (%
) or ems (em
) where appropriate.
animate()
also lets you specify values that are relative to an element’s current values by using '+='
and '-='
. For example, width: '+=50'
will animate the element’s width until it is 50 pixels more than its current width.
Instead of numeric values, you can specify the strings 'show'
, 'hide'
, or 'toggle'
to show, hide, or toggle an element respectively. animate()
then animates the property to show or hide the element as appropriate. For example, width: 'hide'
will reduce the element’s width to zero, then hide the element.
animate()
accepts a duration value as an optional second argument. This behaves like the duration argument in the previous methods.
There’s more to animate()
than what we’ve looked at here, including easing functions and other options. Find out more in the jQuery documentation.
Here’s an example that shows how to animate an element’s position, opacity, width and height all at the same time:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<style type="text/css">
#myBlock { background: green; width: 100px; height: 100px; position: relative; }
</style>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript">
$( init );
function init() {
$('#moveRight').click( function() {
$('#myBlock').animate( {
left: '500px',
opacity: .5,
width: '50px',
height: '50px'
} ); } );
$('#moveLeft').click( function() {
$('#myBlock').animate( {
left: 0,
opacity: 1,
width: '100px',
height: '100px'
} );
} );
}
</script>
</head>
<body>
<div id="myBlock"> </div>
<ul>
<li><a id="moveRight" href="#">Move right</a></li>
<li><a id="moveLeft" href="#">Move left</a></li>
</ul>
</body>
</html>
Creating a sequence of animations
You can chain jQuery animations together to form a sequence of animated effects. There are 3 ways that you can do this:
- Method chaining
- Callback functions
- The
queue()
method
Let’s take a quick look at each technique:
1. Method chaining
You may already be familiar with method chaining in jQuery. It works like this: When you call a method on a jQuery object, the method does its thing then returns that same jQuery object. You can then call another method on the returned object, which again returns the object, and so on. This allows you to call many different methods of an object at once, with just a single line of code:
object.method1().method2().method3();
When you create a chain of animation methods in this way, jQuery queues up the animations. Once the first animation completes, the second animation is triggered, and so on.
Here’s an example that uses method chaining to set up a sequence of 2 animations β a quick fade out, then a slow fade back in:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript">
$( init );
function init() {
$('#fadeOutAndIn').click( function() { $('#myText').fadeOut('fast').fadeIn('slow'); } );
}
</script>
</head>
<body>
<blockquote id="myText">I think he's right, there is something about this, that's... that's so black, it's like, "How
much more black could this be?" and the answer is: "None, none... more black."</blockquote>
<ul>
<li><a id="fadeOutAndIn" href="#">Fade out and in</a></li>
</ul>
</body>
</html>
By the way, you can also write out the method calls the long way, if you prefer:
function init() {
$('#fadeOutAndIn').click( function() {
$('#myText').fadeOut('fast');
$('#myText').fadeIn('slow');
} );
}
The important point is that you call the methods one after the other to build up the animation sequence.
2. Callback functions
All jQuery animation methods can take a callback function as an optional argument. For most methods this is the second argument (after the duration). For animate()
it’s the fourth argument, after the easing function (see the jQuery API page for animate()
).
This callback function is called when the animation completes. The callback function can then kick off another animation, thereby creating an animation sequence.
Of course, your callback function can do anything you want it to! But it’s particularly useful for kicking off other animations.
Let’s use callback functions to recreate the “fade-out-and-back-in” effect from the method chaining example above:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript">
$( init );
function init() {
$('#fadeOutAndIn').click( function() {
$('#myText').fadeOut( 'fast', function() {
$('#myText').fadeIn( 'slow' );
} );
} );
}
</script>
</head>
<body>
<blockquote id="myText">I think he's right, there is something about this, that's... that's so black, it's like, "How
much more black could this be?" and the answer is: "None, none... more black."</blockquote>
<ul>
<li><a id="fadeOutAndIn" href="#">Fade out and in</a></li>
</ul>
</body>
</html>
The queue()
method
The final way to create an animation sequence is to use the queue()
method. This method lets you directly manipulate the queue of animations assigned to an element.
By passing a callback function to the queue()
method, you can add the callback to the end of the queue. When that point in the queue is reached, your callback is called. It’s passed the element that is being animated, as the this
variable. The callback can then queue up more animations on the element (or do something else if you want).
In other words, using queue()
is similar to passing a callback function to an animation method (technique #2 above). The main advantage of queue()
is that you can add your callback function to the queue after you’ve called the initial animation method.
In order to keep the queue of animations moving, your callback should call the dequeue()
method on the element. This causes the next item in the queue to be processed.
Here’s the same effect created by the last 2 examples, but done using queue()
instead:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript">
$( init );
function init() {
$('#fadeOutAndIn').click( function() {
$('#myText').fadeOut( 'fast' );
$('#myText').queue( function() {
$(this).fadeIn( 'slow' );
$(this).dequeue();
} );
} );
}
</script>
</head>
<body>
<blockquote id="myText">I think he's right, there is something about this, that's... that's so black, it's like, "How
much more black could this be?" and the answer is: "None, none... more black."</blockquote>
<ul>
<li><a id="fadeOutAndIn" href="#">Fade out and in</a></li>
</ul>
</body>
</html>
queue()
can do a lot more besides adding callbacks to the animation queue. See the jQuery docs for details.
Adding delays
What if you want to add a pause within a sequence of animations? The
delay()
method does just that. You pass in the duration of the delay using the same format as for other animation methods (either the number of milliseconds, or the strings 'fast'
or 'slow'
).
Just like any other animation method, you can use delay()
within method chaining, or inside callbacks triggered within the queue.
Let’s take our method chaining example from earlier, and add in a 2-second delay between the fade-out and fade-in (changes are in bold text):
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript">
$( init );
function init() {
$('#fadeOutAndIn').click( function() { $('#myText').fadeOut('fast').delay(2000).fadeIn('slow'); } );
}
</script>
</head>
<body>
<blockquote id="myText">I think he's right, there is something about this, that's... that's so black, it's like, "How much more black could this be?" and the answer is: "None, none... more black."</blockquote>
<ul>
<li><a id="fadeOutAndIn" href="#">Fade out and in (with delay)</a></li>
</ul>
</body>
</html>
Stopping an animation
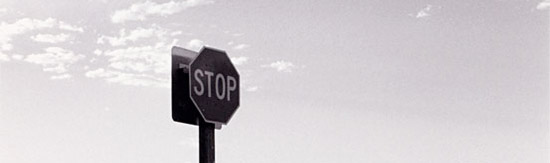
Often it’s useful to be able to stop an animation in its tracks. For example, if you have created a button with an animated rollover effect, you’ll want the animation to stop if the user moves their mouse away from the button.
The stop()
method stops the currently-running animation on an element:
/// Stop the current animation
$('#myElement').stop();
This is fine for a single animation, but what if you’ve set up a queue of animations? In this case, stop()
stops the current animation, and jumps to the next animation in the queue.
If you want to stop all the queued animations as well then pass an argument of true
to stop()
:
/// Stop the current animation and clear the queue of future animations
$('#myElement').stop( true );
By default, stop()
stops the animation completely in its tracks. For example, if you were fading an element in then it would be left in a semitransparent state. To prevent this, you can pass a second argument of true
to stop()
. The animation then stops and the element jumps to the end of the animation, as if the animation had run its course naturally:
/// Stop the current animation, clear the queue, and jump to the end of the animation
$('#myElement').stop( true, true );
Here’s our green square from earlier, this time moving slowly from left to right. The “Start animation” link uses animate()
to start the green block moving. “Stop animation” calls stop()
without any arguments, stopping the block in its tracks, while “Stop animation and jump to end” calls stop()
with true
arguments to clear the queue and move the block to the end of its run:
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<style type="text/css">
#myBlock { background: green; width: 100px; height: 100px; position: relative; }
</style>
<script type="text/javascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript">
$( init );
function init() {
$('#start').click( function() {
$('#myBlock').animate( { left: '500px' }, 10000 );
} );
$('#stop').click( function() { $('#myBlock').stop() } );
$('#stopAndJump').click( function() { $('#myBlock').stop( true, true ) } );
}
</script>
</head>
<body>
<div id="myBlock"> </div>
<ul>
<li><a id="start" href="#">Start animation</a></li>
<li><a id="stop" href="#">Stop animation</a></li>
<li><a id="stopAndJump" href="#">Stop animation and jump to end</a></li>
</ul>
</body>
</html>
A useful jQuery property is jQuery.fx.off
. If you set this to true
then all animations in the page stop and immediately move to their end state. This can be handy when your page is viewed on a less powerful device such as a mobile phone, where animations would slow down the experience for the user.
Summary
In this tutorial you’ve seen just how easy it is to animate page elements with jQuery. Animated effects that take many lines of regular JavaScript code can be accomplished using just 1 or 2 lines of jQuery code. Such is the joy of jQuery!
You can find out more about jQuery’s methods for creating animations in the Effects category on the jQuery site.
Happy coding! π
I made a fairly simple calendar slider using the animate effect discussed which works great in firefox, but won’t work in any of the other browsers. Does anyone know what could be causing this?
Heres the code:
Hi evanmakesthings,
It’s hard to tell what the problem might be without seeing your whole page, with the HTML and CSS – e.g. what’s the value of “$(‘#weekholder’).css(‘left’)”?.
Can you post the URL of the page please?
It took me another day of playing with it before I realized this, but I have not defined the left value in the css. Firefox assumes zero, the rest just break.
Thank you
Lesson learned:
Define any value you want to animate.
[Edited by evanmakesthings on 28-Aug-10 10:07]
Interesting! Glad you got it working. π
Matt
Sometimes it’s not smooth when I use jQuery animation, especially when the left and top value are changing simultaneously, like this:
It’s just sometimes, but kinda annoying. Does Anyone know how to improve it?
Hi youlin,
It might simply be that your browser or computer can’t handle that particular animation smoothly. Are you moving quite a large element/image? Do you have a relatively new computer? Is it jerky on other computers too? What about other browsers?
You might also like to try Spritely – unlike animate() it gives you control over the fps (frames per second) of an animation, which might help you tweak it to run more smoothly:
http://www.spritely.net/
HTH,
Matt