You’re no doubt familiar with using functions in PHP. The language contains hundreds of built-in functions that you can use for all sorts of handy jobs β from manipulating text through to reading files, sending email messages and processing images.
You’re not limited to using just the predefined functions, though β you can create your own functions too! In this tutorial, I’ll show you how. You’ll learn:
- Why it’s good to create your own functions
- How to create and use a function
- How to make your functions flexible by using parameters
- All about optional parameters and default values, and
- How to return values from your functions.
Ready? Let’s get started!
Why make your own functions?

By creating and using your own functions, you get the following advantages:
- You reduce code duplication.
If you’re writing a reasonably long PHP script, chances are that you’ll need to do the same thing more than once at different points in the script. By wrapping this common code in a function, you avoid having to write the code more than once. - You can reuse code across scripts.
Once you’ve encapsulated some code inside a function, it’s easy to separate that function out into a library file. You can then use the function from within many different scripts. - You can write complex code more easily.
It’s easier to write a large application if you split it into many smaller functions, because you only have to think about how to write one simple function at a time. - You can reduce errors in your code.
Rather than having to track down and eliminate the same error in, say, 10 similar blocks of code throughout your application, you only have to isolate and fix the problem in a single function.
How to create a function
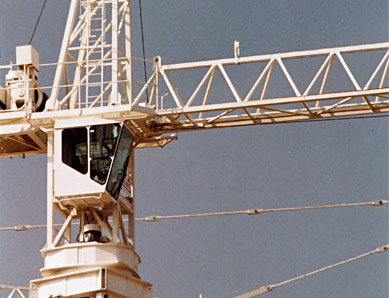
OK, so making your own functions is cool. How do you do it?
Here’s the basic idea:
function myFunctionName() {
// (Your function code goes here)
}
Pretty easy, eh! Here’s an example that shows how to create, then use, a function:
function helloWorld() {
echo "Hello, world!";
}
// Displays: "Hello, world!"
helloWorld();
As you can see, you use, or call, a function you’ve created yourself in exactly the same way as you call a built-in PHP function. You write the function name, followed by ()
.
When the PHP engine encounters your function call, it passes control to the function and runs the code inside it. When the function’s code has finished, control is returned to the point after your function call.
Defining and using parameters

Great! Now you know how to create your own functions, and how to call a function you’ve created from elsewhere in your code. Remember that functions are reusable β you can call the same function from many different points in your code.
Reusable functions are great, but in order to make them even more useful and flexible, you can use parameters and arguments:
- Parameters are special variables that you set up when you create your function. These variables can accept values from the code that calls the function. The code inside your function can then work with those values.
- Arguments are those values that you pass to the function when you call it.
This means that your functions can do different things based on the values that you give them, making them much more flexible.
To define parameters in a function, you specify the parameter names inside the parentheses when you create the function:
function myFunctionName( parameter1, parameter2, ... ) {
// (Your function code goes here)
}
To pass values to your function when you call it, you again put them inside the parentheses:
myFunctionName( argument1, argument2, ... )
Here’s an example to make this crystal clear:
function sayHelloTo( $firstName, $lastName ) {
echo "Hello, $firstName $lastName!<br>";
}
// Displays: "Hello, John Smith!"
sayHelloTo( "John", "Smith" );
// Displays: "Hello, Mary White!"
sayHelloTo( "Mary", "White" );
Here’s how the above code works:
- First we create a function called
sayHelloTo()
that has 2 parameters,$firstName
and$lastName
. The function code displays these 2 values usingecho
. - We then call the function, passing in the values
"John"
and"Smith"
. The value"John"
is transferred to the$firstName
parameter, and"Smith"
is transferred to$lastName
. The end result is that the function displays “Hello, John Smith!”. - We then call the function again with different arguments, producing a different result: “Hello, Mary White!”.
So by adding parameters to our sayHelloTo()
function, we’ve made the function much more flexible. It can now say hi to anybody!
Creating optional parameters
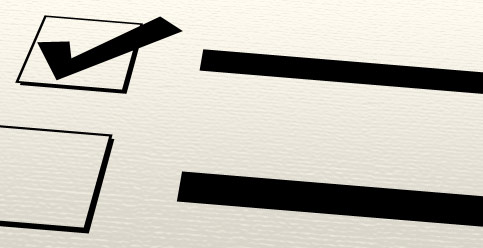
Sometimes it’s useful to make parameters optional. When you make a parameter optional, you also give it a default value. If the calling code doesn’t pass a value for that parameter, the default value is used instead.
Here’s how to define an optional parameter:
function myFunctionName( parameterName=defaultValue ) {
// (Your function code goes here)
}
Let’s make an example that uses optional parameters. We’ll take the sayHelloTo()
function we wrote earlier, and add an optional parameter for a custom greeting. If the calling code doesn’t pass a value for the parameter, we’ll default to “Hello”:
function sayHelloTo( $firstName, $lastName, $greeting="Hello" ) {
echo "$greeting, $firstName $lastName!<br>";
}
// Displays: "Hello, John Smith!"
sayHelloTo( "John", "Smith" );
// Displays: "Howdy, Mary White!"
sayHelloTo( "Mary", "White", "Howdy" );
As you can see, we’ve defined our function with an optional parameter and default value. We then call the function twice. The first time, we don’t pass a value for the $greeting
parameter, so the function defaults to “Hello”. The second time, we pass the value “Howdy” for the $greeting
parameter, so “Howdy” is used instead of the default.
Returning values from your functions
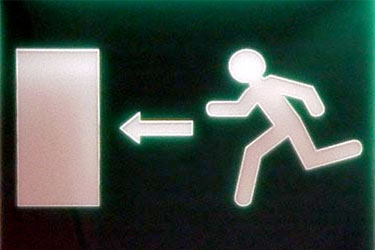
To make your functions even more versatile, you can get them to return values back to your calling code. This means your calling code can have a 2-way conversation with your function: When it calls the function it can pass the function some values, and it can also receive a reply from the function when the function ends.
Both parameters and return values are optional. A function can have parameters or return a value, or it can do both, or neither.
To return a value from a function, you use the return
keyword, as follows:
function myFunctionName() {
// (Your function code goes here)
return value;
}
When you then call the function, the function call takes on the returned value. For example, you can read the returned value in your calling code like this:
$returnValue = myFunctionName();
Let’s rewrite our sayHelloTo()
function above to return the greeting string, instead of displaying it. We can then display it from within our calling code:
function sayHelloTo( $firstName, $lastName, $greeting="Hello" ) {
return "$greeting, $firstName $lastName!<br>";
}
// Displays: "Hello, John Smith!"
echo sayHelloTo( "John", "Smith" );
// Displays: "Howdy, Mary White!"
echo sayHelloTo( "Mary", "White", "Howdy" );
On the surface this seems pretty pointless, since the result is exactly the same as before. However, the key point here is that the function has become more general-purpose. Before, you could only use the function to display a greeting. Now that the function returns the greeting, the calling code can do whatever it wants with the greeting string. For example, rather than displaying the greeting, it could instead store the greeting in a file by calling PHP’s file_put_contents()
function:
$johnsGreeting = sayHelloTo( "John", "Smith" );
file_put_contents( "johnsGreeting.txt", $johnsGreeting );
When you call return
with a value, the function immediately exits at that point and returns the value. You can also call return
without a value, in which case the function simply exits at that point.
Summary
In this tutorial you’ve learned the basics of creating your own functions in PHP. You’ve looked at defining and calling functions; setting up parameters; and returning values from functions.
Once you start writing your own functions, you can create more powerful and flexible PHP applications. In the next couple of tutorials I’ll take you through some more advanced function-related concepts, including scope, references, and recursion. Watch this space!
thanks that was amazing i didn’t know this much about functions
Thanks Vivek – glad you found it helpful. π
Great tutorial, thank you very much for the time and effort you put on it. very useful!
[Edited by yairsaumeth on 23-Oct-11 22:11]
@yairsaumeth: You’re welcome – thanks for the feedback! π