As I mentioned in my earlier article, Creating Arrays in PHP, arrays are very useful if you need to store and manipulate a large amount of data in one go. One useful way you can manipulate this data is to sort it in a particular order.
PHP gives you a large number of sorting functions that can sort arrays using many different criteria. For example, you can sort in ascending or descending order, by key, or by value. These functions make it really easy to sort arrays without getting bogged down in the details of sort algorithms.
We won’t try to cover every single PHP array-sorting function in this tutorial. Instead, you’ll learn about the functions that you’re likely to find the most useful in your everyday coding.
Let’s get started!
Sorting indexed arrays: sort()
and rsort()
The sort()
function sorts the values in an indexed array in ascending order. This means that A comes before Z, and 0 comes before 9. Uppercase letters come before lowercase letters, and all letters come before numbers. For example:
$myArray = array( 1, 2, 'a', 'b', 'A', 'B' );
sort( $myArray );
// Displays "A B a b 1 2"
foreach ( $myArray as $val ) echo "$val ";
rsort()
works like sort()
, except it sorts in descending order:
$myArray = array( 1, 2, 'a', 'b', 'A', 'B' );
rsort( $myArray );
// Displays "2 1 b a B A"
foreach ( $myArray as $val ) echo "$val ";
All the sorting functions in this tutorial return true
on success and false
on failure.
Sorting associative arrays: asort()
and arsort()
sort()
and rsort()
are fine for indexed arrays, where you don’t usually care about the relationship between keys and values. However, they can cause problems with associative arrays. Take the following example:
$movie = array( "title" => "Rear Window",
"director" => "Alfred Hitchcock",
"year" => 1954,
"minutes" => 112 );
sort( $movie );
// Displays "Array ( [0] => Alfred Hitchcock [1] => Rear Window [2] => 112 [3] => 1954 )"
print_r( $movie );
As you can see, the sort()
function has reindexed the array with numeric indices, destroying the original string indices of "title"
, "director"
, "year"
and "minutes"
.
If you want to sort the values in an associative array while preserving keys, use asort()
and arsort()
instead. These functions preserve not only the keys, but also the relationships between the keys and their values.
To sort the values in ascending order, use asort()
:
$movie = array( "title" => "Rear Window",
"director" => "Alfred Hitchcock",
"year" => 1954,
"minutes" => 112 );
asort( $movie );
// Displays "Array ( [director] => Alfred Hitchcock [title] => Rear Window [minutes] => 112 [year] => 1954 )"
print_r( $movie );
arsort()
sorts the values in descending order — again while preserving the keys:
$movie = array( "title" => "Rear Window",
"director" => "Alfred Hitchcock",
"year" => 1954,
"minutes" => 112 );
arsort( $movie );
// Displays "Array ( [year] => 1954 [minutes] => 112 [title] => Rear Window [director] => Alfred Hitchcock )"
print_r( $movie );
Sorting associative arrays by key: ksort()
and krsort()
As well as sorting associative arrays by value, you can also sort them by key. ksort()
sorts the elements in ascending key order, while krsort()
sorts in descending key order. Like asort()
and arsort()
, these functions preserve the relationships between keys and values.
Here are some examples:
$movie = array( "title" => "Rear Window",
"director" => "Alfred Hitchcock",
"year" => 1954,
"minutes" => 112 );
// Displays "Array ( [director] => Alfred Hitchcock [minutes] => 112 [title] => Rear Window [year] => 1954 )"
ksort( $movie );
print_r( $movie );
// Displays "Array ( [year] => 1954 [title] => Rear Window [minutes] => 112 [director] => Alfred Hitchcock )"
krsort( $movie );
print_r( $movie );
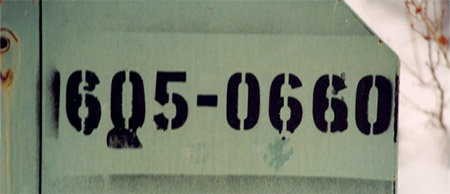
Sorting multiple arrays and multidimensional arrays with array_multisort()
The powerful array_multisort()
function can sort multiple related arrays in one go, preserving the relationships between the arrays. It can also sort multidimensional arrays, so it’s a pretty flexible function! Let’s take a look at sorting multiple arrays first, then we’ll explore sorting multidimensional arrays.
Sorting multiple arrays
To sort multiple arrays using array_multisort()
, simply pass in all the arrays you want to sort. Here’s an example:
$directors = array( "Stanley Kubrick", "Alfred Hitchcock", "Martin Scorsese" );
$titles = array( "Full Metal Jacket", "Rear Window", "Mean Streets" );
$years = array( 1987, 1954, 1973 );
array_multisort( $directors, $titles, $years );
print_r( $directors );
echo "<br />";
print_r( $titles );
echo "<br />";
print_r( $years );
echo "<br />";
The above code displays the following:
Array ( [0] => Alfred Hitchcock [1] => Martin Scorsese [2] => Stanley Kubrick ) Array ( [0] => Rear Window [1] => Mean Streets [2] => Full Metal Jacket ) Array ( [0] => 1954 [1] => 1973 [2] => 1987 )
Notice how array_multisort()
has sorted the values in $directors
in ascending order, then sorted the other 2 arrays so that the element order matches that of the now-sorted $directors
array.
What if you want to sort by, say, title instead? Just put $titles
first in the list:
array_multisort( $titles, $directors, $years );
Sort by… then by…
If the first array contains some identical values then array_multisort()
sorts by the values in the first array, then by the values in the second array, and so on. For example:
$directors = array( "Stanley Kubrick", "Alfred Hitchcock", "Martin Scorsese", "Stanley Kubrick" );
$titles = array( "Full Metal Jacket", "Rear Window", "Mean Streets", "A Clockwork Orange" );
$years = array( 1987, 1954, 1973, 1971 );
array_multisort( $directors, $titles, $years );
print_r( $directors );
echo "<br />";
print_r( $titles );
echo "<br />";
print_r( $years );
echo "<br />";
The above code outputs the following — notice that "A Clockwork Orange"
appears before "Full Metal Jacket"
:
Array ( [0] => Alfred Hitchcock [1] => Martin Scorsese [2] => Stanley Kubrick [3] => Stanley Kubrick ) Array ( [0] => Rear Window [1] => Mean Streets [2] => A Clockwork Orange [3] => Full Metal Jacket ) Array ( [0] => 1954 [1] => 1973 [2] => 1971 [3] => 1987 )
Changing sort order
You can pass an optional flag argument after an array argument to control the sort order for that particular array:
SORT_ASC
- Sort in ascending order (the default)
SORT_DESC
- Sort in descending order
There are other flags that you can pass to array_multisort()
— and other sorting functions, for that matter — to control the way that arrays are sorted. See the sort()
entry in the PHP manual for details.
The following example sorts the $directors
array in ascending order, then the $titles
array in descending order:
$directors = array( "Stanley Kubrick", "Alfred Hitchcock", "Martin Scorsese", "Stanley Kubrick" );
$titles = array( "Full Metal Jacket", "Rear Window", "Mean Streets", "A Clockwork Orange" );
$years = array( 1987, 1954, 1973, 1971 );
array_multisort( $directors, SORT_ASC, $titles, SORT_DESC, $years );
print_r( $directors );
echo "<br />";
print_r( $titles );
echo "<br />";
print_r( $years );
echo "<br />";
Here’s the output — notice that "Full Metal Jacket"
now comes before "A Clockwork Orange"
:
Array ( [0] => Alfred Hitchcock [1] => Martin Scorsese [2] => Stanley Kubrick [3] => Stanley Kubrick )
Array ( [0] => Rear Window [1] => Mean Streets [2] => Full Metal Jacket [3] => A Clockwork Orange )
Array ( [0] => 1954 [1] => 1973 [2] => 1987 [3] => 1971 )
Sorting multidimensional arrays
If you pass a multidimensional array to array_multisort()
then it sorts the array by looking at the first element of each nested array. If 2 such elements have the same value then it sorts by the second element, and so on.
Here’s an example that shows how this works. It uses array_multisort()
to sort a multidimensional array by director, then by title, then by year:
$movies = array(
array(
"director" => "Alfred Hitchcock",
"title" => "Rear Window",
"year" => 1954
),
array(
"director" => "Stanley Kubrick",
"title" => "Full Metal Jacket",
"year" => 1987
),
array(
"director" => "Martin Scorsese",
"title" => "Mean Streets",
"year" => 1973
),
array(
"director" => "Stanley Kubrick",
"title" => "A Clockwork Orange",
"year" => 1971
)
);
array_multisort( $movies );
echo "<pre>";
print_r( $movies );
echo "</pre>";
The above code produces the following:
Array
(
[0] => Array
(
[director] => Alfred Hitchcock
[title] => Rear Window
[year] => 1954
)
[1] => Array
(
[director] => Martin Scorsese
[title] => Mean Streets
[year] => 1973
)
[2] => Array
(
[director] => Stanley Kubrick
[title] => A Clockwork Orange
[year] => 1971
)
[3] => Array
(
[director] => Stanley Kubrick
[title] => Full Metal Jacket
[year] => 1987
)
)
You can see that array_multisort()
has sorted the array by director. Where the director is the same ("Stanley Kubrick"
), it’s then sorted by title.
To sort the array in descending order instead, pass the SORT_DESC
flag as the second argument to array_multisort()
. Easy!
Summary
In this tutorial you’ve looked at a few common PHP functions for sorting arrays:
sort()
andrsort()
for sorting indexed arraysasort()
andarsort()
for sorting associative arraysksort()
andkrsort()
for sorting associative arrays by keyarray_multisort()
for sorting multiple related arrays, as well as multidimensional arrays
PHP’s array sorting functions are very powerful and flexible, and let you easily and quickly sort arrays any way you like. In fact, at the time of writing there are 13 different PHP functions for sorting arrays! To find out more, check out this list in the PHP manual.
Happy coding!
Beautifully nice and simple,
thanks!
@voltumna: Thanks for your comment – I’m happy you enjoyed the tutorial. 🙂